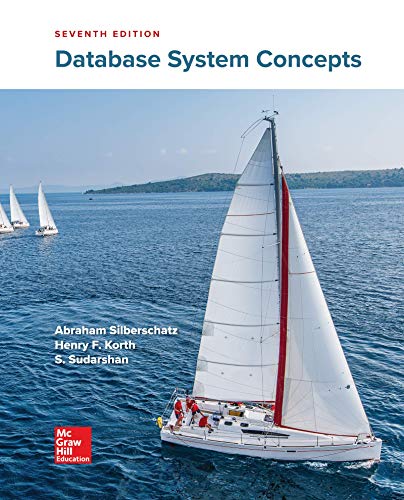
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
#include <iostream>
#include <cmath>
using namespace std;
// declare functions
void display_menu();
void convert_temp();
double to_celsius(double fahrenheit);
double to_fahrenheit(double celsius);
int main() {
cout << "Convert Temperatures\n\n";
display_menu();
char again = 'y';
while (again == 'y')
{
convert_temp();
cout << "Convert another temperature? (y/n): ";
cin >> again;
cout << endl;
}
cout << "Bye!\n";
}
// define functions
void display_menu()
{
cout << "MENU\n"
<< "1. Fahrenheit to Celsius\n"
<< "2. Celsius to Fahrenheit\n\n";
}
void convert_temp()
{
int option;
cout << "Enter a menu option: ";
cin >> option;
double f = 0.0;
double c = 0.0;
switch (option)
{
case 1:
cout << "Enter degrees Fahrenheit: ";
cin >> f;
c = to_celsius(f);
c = round(c * 10) / 10; // round to one decimal place
cout << "Degrees Celsius: " << c << endl;
break;
case 2:
cout << "Enter degrees Celsius: ";
cin >> c;
f = to_fahrenheit(c);
f = round(f * 10) / 10; // round to one decimal place
cout << "Degrees Fahrenheit: " << f << endl;
break;
default:
cout << "You must enter a valid menu number.\n";
break;
}
}
double to_celsius(double fahrenheit)
{
double celsius = (fahrenheit - 32.0) * 5.0 / 9.0;
return celsius;
}
double to_fahrenheit(double celsius)
{
double fahrenheit = celsius * 9.0 / 5.0 + 32.0;
return fahrenheit;
}
- Now, modify it to do the following:
- Modify the while loop to utilize tolower() or toupper().
-
- Add default values to the to_celsius() and to_fahrenheit() functions.
- Add default values to the to_celsius() and to_fahrenheit() functions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardC++ programming task. main.cc file: #include <iostream>#include <map>#include <vector> #include "plane.h" int main() { std::vector<double> weights{3.2, 4.7, 2.1, 5.5, 9.8, 7.4, 1.6, 9.3}; std::cout << "Printing out all the weights: " << std::endl; // ======= YOUR CODE HERE ======== // 1. Using an iterator, print out all the elements in // the weights vector on one line, separated by spaces. // Hint: see the README for the for loop syntax using // an iterator, .begin(), and .end() // ========================== std::cout << std::endl; std::map<std::string, std::string> abbrevs{{"AL", "Alabama"}, {"CA", "California"}, {"GA", "Georgia"}, {"TX", "Texas"}}; std::map<std::string, double> populations{ {"CA", 39.2}, {"GA", 10.8}, {"AL", 5.1}, {"TX", 29.5}}; std::cout <<…arrow_forwardMain.cpp #include <iostream>#include "Deck.h" int main() { Deck deck; deck.shuffle(); std::cout << "WAR Card Game\n\n"; std::cout << "Dealing cards...\n\n"; Card player1Card = deck.Deal(); Card player2Card = deck.Deal(); std::cout << "Player 1's card: "; player1Card.showCard(); std::cout << std::endl; std::cout << "Player 2's card: "; player2Card.showCard(); std::cout << std::endl; int player1Value = player1Card.getValue(); int player2Value = player2Card.getValue(); if (player1Value > player2Value) { std::cout << "Player 1 wins!" << std::endl; } else if (player1Value < player2Value) { std::cout << "Player 2 wins!" << std::endl; } else { std::cout << "It's a tie!" << std::endl; } return 0;} Card.h #ifndef CARD_H#define CARD_H class Card {public: Card(); Card(char r, char s); int getValue(); void showCard();…arrow_forward
- Flowchart, create. #include <iostream>using namespace std;// Write function declaration here void multiplyNumbers(int, int, int &);int main(){ int num1 = 10; int num2 = 20; int product = 0; // Print value of product before function call cout << "Value of product is: " << product << endl; // Call multiplyNumbers using pass by reference for product multiplyNumbers(num1, num2, product); // Print value of calculated product cout << num1 << " * " << num2 << " is " << product << endl; return 0;} // End of main function// Write multiplyNumbers function here; use pass by reference for result of multiplication. Then use pass by address. void multiplyNumbers(int n1, int n2, int &result) { result = n1*n2;}arrow_forwardchange normal body temperature to 36.5–37.5 °C #include<iostream>using namespace std;int main(){ float temp;cout<<"Enter the body temperature in Celsius\n";cin>>temp;if (temp>=37){cout<<"The quarantine of the patient is required\n";cout<<"Enter the name of the patient\n";string patient;cin>>patient;cout<<"Where the passenger is from\n";string place;cin>>place;cout<<patient<<" from "<<place<<"is to be quarantined at Hospital"; }cout<<"Normal temperature\n";}arrow_forwardجواب هذا السؤال اذا امكن بىمجة ثاني كليهarrow_forward
- Create the following c functions:- void acceptSubscribers(Subscriber *, int size); // accept all subscriber inputs- void displaySubscribers(Subscriber *, int size); // display all subscribers- void displaySubscriber(Subscriber); // display 1 subscriber- Subscriber * getSubscribersWithPlan(Subscriber *, int size, char *); // get subscribers with desired planarrow_forward/* Programming concept: structures Program should: be a C program, define a structure to store the year, month, day, and high temperature. write a function get_data that has a parameter that is a pointer to a struct and reads from the user into the struct pointed to by the parameter. write a function print_data that takes the struct pointer as a parameter and prints the data. in main, declare a struct, call the get_data function, call the print_data function. */ #include <stdio.h> int main(int argc, char *argv[]) { return 0;}arrow_forward#include <iostream> using namespace std; void times(int& prod, int mpr, int mcand) { prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } } int main(){ int product, n, m; cout << "Enter two numbers: "; cin >> n >> m; times(product, n, m); cout << "Product: " << product << endl; return 0; } Convert the product into pep9 assembly language.arrow_forward
- int x1 = 66; int y1 = 39; int d; _asm { } mov EAX, X1; mov EBX, y1; push EAX; push EBX; pop ECX mov d, ECX; What is d in decimal format?arrow_forward4. s = 1; do { s++; cout 12) 468 10 148 12arrow_forward// SumAndProduct.cpp - This program computes sums and products // Input: Interactive// Output: Computed sum and product #include <iostream>#include <string>void sums(int);void products(int);using namespace std; int main() { int number; cout << "Enter a positive integer or 0 to quit: "; cin >> number; while(number != 0) { // Call sums function here // Call products function here cout << "Enter a positive integer or 0 to quit: "; cin >> number; } return 0;} // End of main function// Write sums function here// Write products function herearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
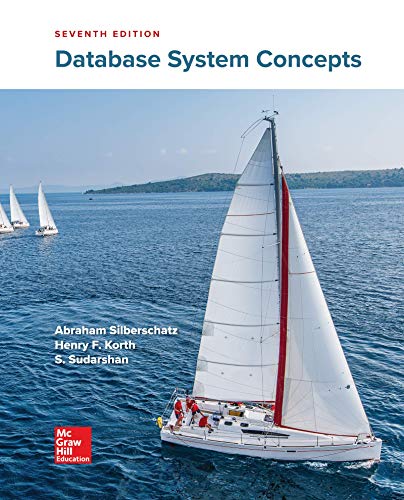
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
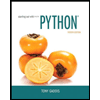
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
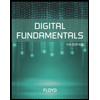
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
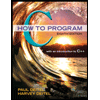
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
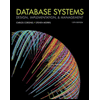
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
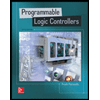
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education