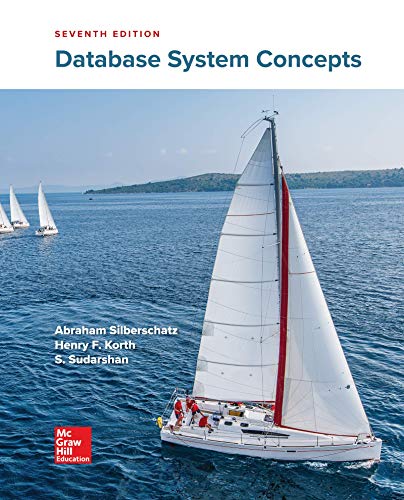
IN PYTHON - please help with lines beginning with ###TODO
import csv
import random as rand
import sys
# define functions
def load_csv_data(filename):
"""
load the data from csv file
returns a tuple containing two lists:
col_names: first row of csv file (list of strings)
row_data: all other rows of csv file (list of list of strings)
if error is encountered reading filename, returns -1
"""
try:
data = []
### TODO - load the data from csv file
### use csv.reader to append rows of filename to data
except:
print("Error loading data.")
return -1
# if successful, return tuple of column names and row data
col_names = data[0]
row_data = data[1:]
return (col_names, row_data)
def display_menu():
### TODO - print the main menu
print("Main Menu")
print("1 - Get Clean Sample")
print("2 - Calculate Total Profit in Sample")
print("Q - Quit")
def get_valid_menu_option():
### TODO - get valid menu option from the user
### use prompt ">>> choose menu option: "
### if not valid selection, print message "invalid input" and get again
### repeat until valid and return that result
user_input = (">>> choose menu option: ")
if user_input != '1':
print("invalid input")
elif user_input != '2':
print("invalid input")
elif user_input != 'Q':
print("invalid input")
else:
print(">>> choose menu option: ")
return user_input
def get_clean_sample(data, n=20):
# get n rows randomly sampled w/o replacement from the data
sample = rand.sample(data, n)
### TODO - convert string types in all rows of sample to int / float type
### positions 0 and 8 should be integers
### positions 9 and 10 should be floats
### return the updated sample
def print_sample_summary(sample):
"""print summary data for a given sample, returns nothing"""
print(f"\nSample size {len(sample)} rows x {len(sample[0])} cols.")
print("First order number:\t", sample[0][0])
print("Last order number:\t", sample[-1][0])
tot = 0
for row in sample:
tot += row[8]
avg = tot / len(sample)
print("Average units per order:", avg)
# check if run as module or script
if __name__ == "__main__":
# set random seed
seed = input("*** Enter random seed (integer) or ENTER for none: ")
if seed:
rand.seed(int(seed))
print("\nRandom seed set to", seed)
else:
print("\nNo random seed set")
# load csv data file
# this file must be in the same directory as your .py file
file = "5000 Sales Records.csv"
data = load_csv_data(file)
if data == -1:
print("Ending.")
sys.exit()
else:
# unpack the data tuple into header and rows; print result
col_names, row_data = data
print(f"\nLoaded '{file}', {len(row_data)} rows x {len(row_data[0])} cols.")
### TODO - implement main menu loop per specification

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- javascript please //20. function initPage//a. Dependencies: getUsers, populateSelectMenu//b. Should be an async function//c. No parameters.//d. Call await getUsers//e. Result is the users JSON data//f. Passes the users JSON data to the populateSelectMenu function//g. Result is the select element returned from populateSelectMenu//h. Return an array with users JSON data from getUsers and the select element//result from populateSelectMenu: [users, select] html <body> <header> <h1>Acme Blogs</h1> <form id="filterForm"> <select id="selectMenu"> <option selected>Employees</option> </select> </form> </header> <main> <p class="default-text">Select an Employee to display their posts.</p> </main> <footer> <p>Acme Company</p> </footer> <div id="mocha" class="testResults"></div>…arrow_forwardIn C++ Create a program that outputs 10 strings (that the user inputs) to a file.arrow_forwardComputer Science Please use go language programma to write this code. Write a program that creates a CSV file to store and retrieve data such as name, age, and salary. You should be able to read the data out of the file and display the data sorted by name. Hint: Make use of the Sort package to sort the data. Make sure you check the existence of the file, closing the file when there is an error Use defer, panic, and recover as needed.arrow_forward
- Please let me know the code for this as I am not able to upload the JSON file The JSON fileprovided, climate.json contains monthly climate data of over one hundred destinations and includes average high and low temperature, dry days, snow days, and rainfall, for every month.Program TasksWrite a program display Climate.py that performs the following functionalitiesa. reads the JSON file climate.jsonb. displays the cities as a listc. prompts the user to enter a city or citiesd. searches for the relevant data and display ((i) the average rainfall for each month as a list(ii) minimum and maximum rainfall per year.(iii) the average rainfall per year.A brief introduction to the JSON fileStructure of the climate JSON file:• JSON data works just like python dictionary and you can access the value usingdictionaryName[key]• The JSON file contains a list of 104 dictionary items and each dictionary item has 4 keys.• The monthlyAvg key has a list as its value, which in return contains 12…arrow_forward5. In lab12_p5.py a function read_data_file has been created. This function takes in one string parameter that represents the name of the file to be processed. This function reads data from the provided file and returns a dictionary representation of the data stored in this file. The data file is a comma-separated file with the following columns: • County name • Tons of coal produced in 1900 • Tons of coal produced in 1910 © Run Online (1) zbessant Bessant lab12_p1.py x lab12_p5.py x lab12 p3.pyx lab12_p2.py x coal production. 1 # Problem #5 2 3 4 5 6 7 8 # Read the contents of a coal data file and return the data in a dictionary format. def read_data_file(filename): data file open(filename, "r") header_line = data_file.readline () # Read the header line of the file to extract years years = header_line.strip().split(",")[1:] # Extract the years from the header line Tons of coal produced in 1920 9 10 Tons of coal produced in 1930 11 # Initialize an empty dictionary to store the data…arrow_forwardPython 3 Given that a variable named pathName contains a string describing a directory path, write a single Python statement that will store the names of all files and subdirectories in that path in a List structure named contents. DO NOT traverse the path/directory. You are only interested in the immediate path and its contents. You may assume that any necessary import statements already exist.arrow_forward
- Use C++ Language Problem 2 (Save and Get Info): Write a program that asks for the user's name, phone number, and address. The program then saves all information in a data file (each information in one line) named list.txt. Finally, the program reads the information from the file and displays it on the screen in the following format:Name: User's Name Phone Number: User's Phone Number Address: User's Street Address User's City, State, and Zip Codearrow_forwardIn C++ Create a function that takes in an array and outputs the min, max and average of the array to a file.arrow_forwardmatlabarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
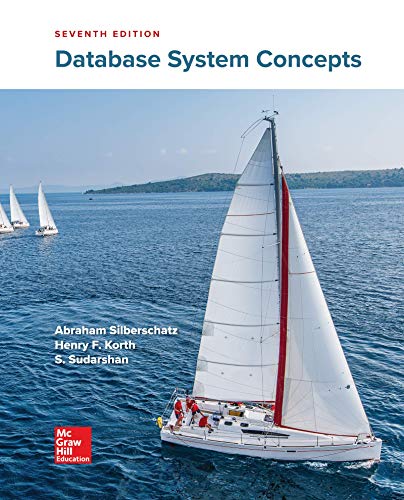
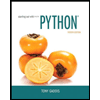
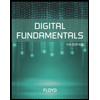
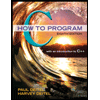
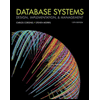
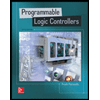