Address any questions or misconceptions the reviewer may have posted. (I like your program! I don't see very many things to nitpick at. Perhaps, instead of using "cout << "---------------" << endl;" you could use something along the lines of "cout << setw(10) << setfill('-') << "-" << endl;" in case you wanted to change that part or prevent changes to how it looks on different screens. Good job on the comments and everything.)
#include <iostream>
#include<iomanip>
#include<fstream>
#include<sstream>
#include<string.h>
using namespace std;
//Define the main function
int main()
{
//Create the object of fstream class and opening the file
ifstream inpfile("order.txt");
string fline;
double sub_total, tax, shipping=14.95, grand_total, line_total;
double priceA=17.46, priceB=10.13, priceC=2.11, priceD=23.13, priceE=74.56, priceF=1.11, priceG=9.34, priceH=3.45;
cout<<"Thank You! Your order is summarized below: "<<endl;
cout<<"-----------------------------------------------"<<endl;
cout<<"| Product\t|Quantity\t|Line total |"<<endl;
cout<<"-----------------------------------------------"<<endl;
while (getline(inpfile, fline)) {
istringstream ists(fline);
string product;
int quantity;
if (!(ists >>product>> quantity)) {
break;
}
else{
if(product=="A")
line_total=quantity*priceA;
else if (product=="B")
line_total=quantity*priceB;
else if (product=="C")
line_total=quantity*priceC;
else if (product=="D")
line_total=quantity*priceD;
else if (product=="E")
line_total=quantity*priceE;
else if (product=="F")
line_total=quantity*priceF;
else if (product=="G")
line_total=quantity*priceG;
else if (product=="H")
line_total=quantity*priceH;
cout<<"| "<<setw(2)<<product<<"\t\t| "<<setw(2)<<quantity <<"\t\t| "<<fixed<<setprecision(2)<<setw(10)<<line_total<<setw(2)<<" |"<<endl;
cout<<"-----------------------------------------------"<<endl;
sub_total=sub_total+line_total;
}
tax=sub_total*0.17;
grand_total=sub_total+tax+shipping;
}
cout<<"| "<<setw(2)<<"Sub_total"<<"\t| "<<setw(2)<<" " <<"\t\t| "<<fixed<<setprecision(2)<<setw(10)<<sub_total<<setw(2)<<" |"<<endl;
cout<<"-----------------------------------------------"<<endl;
cout<<"| "<<setw(2)<<"Tax"<<"\t\t| "<<setw(2)<<" " <<"\t\t| "<<fixed<<setprecision(2)<<setw(10)<<tax<<setw(2)<<" |"<<endl;
cout<<"-----------------------------------------------"<<endl;
cout<<"| "<<setw(2)<<"Shipping"<<"\t| "<<setw(2)<<" " <<"\t\t| "<<fixed<<setprecision(2)<<setw(10)<<shipping<<setw(2)<<" |"<<endl;
cout<<"-----------------------------------------------"<<endl;
cout<<"| "<<setw(2)<<"Grand Total"<<"\t| "<<setw(2)<<" " <<"\t\t| "<<fixed<<setprecision(2)<<setw(10)<<grand_total<<setw(2)<<" |"<<endl;
cout<<"-----------------------------------------------"<<endl;
inpfile.close();
return 0;
}
Programming Assignment 2
#include <iostream>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
{
float lowerTemp,higherTemp,increment;
cout<<"Please Enter the lower value for the conversion table (Celsius)";
cin>> lowerTemp;
cout<<"Please Enter the higher value for the conversion table (Celsius)";
cin>> higherTemp;
//input validation
if(lowerTemp > higherTemp){
cout<<"lower value should not greater that higher value";
return 1;
}
cout<<"what do you want to use for incremented value";
cin>> increment;
if(increment <= 0){
cout<<"The increament value should be greater than 0.";
return 1;
}
ofstream write_data;
write_data.open("conversion_table.txt");
if(!write_data) { //error
cerr << "Error in opening the file." << endl;
exit(1);
}
//print heading
write_data << "C \t F \t N" <<endl;
//compute temp equivalent
//save data into the file
while (lowerTemp <= higherTemp){
float f = (9/5) * lowerTemp + 32;
float n = 0.33 * lowerTemp;
write_data << fixed << setprecision(2) <<lowerTemp << "\t" <<
setprecision(2) << f << "\t" << setprecision(2) << n <<endl;
lowerTemp += increment;
}
cout<<"\nData is saved in the file.";
write_data.close();
return 0;
}
Question-
-
- Address any questions or misconceptions the reviewer may have posted. (I like your program! I don't see very many things to nitpick at. Perhaps, instead of using "cout << "---------------" << endl;" you could use something along the lines of "cout << setw(10) << setfill('-') << "-" << endl;" in case you wanted to change that part or prevent changes to how it looks on different screens. Good job on the comments and everything.)
- Indicate how useful the feedback was.
- Did you understand the comments/observations/suggestions?
- Did the review point out flaws/shortcomings in your program?
- Did you learn anything from the critique?
- Did the process help you better understand the original programming assignment? would you modify your program based on the feedback/observations?

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

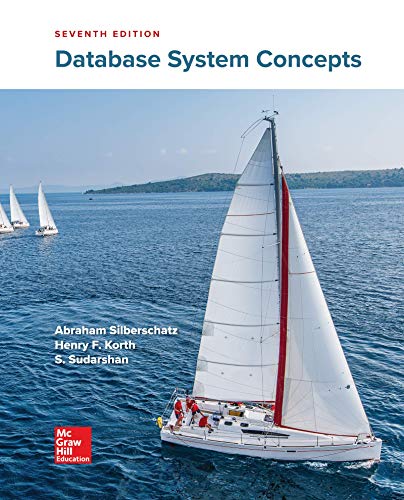
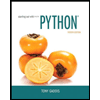
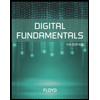
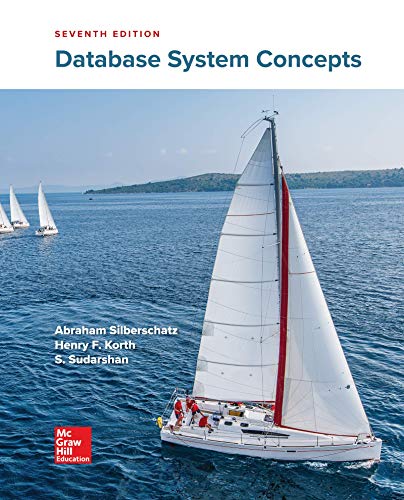
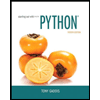
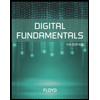
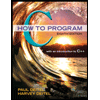
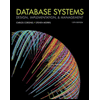
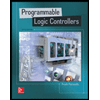