nclude #include #include using namespace std; class Caesar { public: void encrypt(char *inp,char *out,int key); void decrypt(char *inp,char *out,int key); void readText(char *inp); void encrypt1(); void decrypt1(); }; void Caesar::encrypt(char *inp,char *out,int key)
#include<iostream>
#include<string>
#include<fstream>
using namespace std;
class Caesar
{
public: void encrypt(char *inp,char *out,int key);
void decrypt(char *inp,char *out,int key);
void readText(char *inp);
void encrypt1();
void decrypt1();
};
void Caesar::encrypt(char *inp,char *out,int key)
{
ifstream input;
ofstream output;
char buf;
input.open(inp);
output.open(out);
buf=input.get();
while(!input.eof())
{
if(buf>='a'&&buf<='z')
{
buf-='a';
buf+=key;
buf%=26;
buf+='A';
}
output.put(buf);
buf=input.get();
}
input.close();
output.close();
readText(inp);
readText(out);
}
void encrypt1()
{
char message[100], ch;
int i, key;
ofstream myfile ("encrypt.dat");
cout << "Enter a message to encrypt: ";
cin>>message;
cout << "\nEnter key: ";
cin >> key;
for(i = 0; message[i] != '\0'; ++i){
ch = message[i];
if(ch >= 'a' && ch <= 'z'){
ch = ch + key;
if(ch > 'z'){
ch = ch - 'z' + 'a' - 1;
}
message[i] = ch;
}
else if(ch >= 'A' && ch <= 'Z'){
ch = ch + key;
if(ch > 'Z'){
ch = ch - 'Z' + 'A' - 1;
}
message[i] = ch;
}
myfile << message[i] << " " ;
}
}
void decrypt1()
{
char message[100], ch;
int i, key;
ofstream myfile ("decrypt.txt");
cout << "Enter a message to decrypt: ";
cin>>message;
cout << "Enter key: ";
cin >> key;
for(i = 0; message[i] != '\0'; ++i){
ch = message[i];
if(ch >= 'a' && ch <= 'z'){
ch = ch - key;
if(ch < 'a'){
ch = ch + 'z' - 'a' + 1;
}
message[i] = ch;
}
else if(ch >= 'A' && ch <= 'Z'){
ch = ch - key;
if(ch > 'a'){
ch = ch + 'Z' - 'A' + 1;
}
message[i] = ch;
}
myfile << message[i] << " " ;
}
}
void Caesar::decrypt(char *inp,char *out,int key)
{
ifstream input;
ofstream output;
char buf;
input.open(inp);
output.open(out);
buf=input.get();
while(!input.eof())
{
if(buf>='A'&&buf<='Z')
{
buf-='A';
buf+=26-key;
buf%=26;
buf+='a';
}
output.put(buf);
buf=input.get();
}
input.close();
output.close();
readText(inp);
readText(out);
}
void Caesar::readText(char *inp)
{
ifstream input;
char buf;
input.open(inp);
cout<<"\n\n <--- "<<inp<<" --->\n";
buf=input.get();
while(!input.eof())
{
cout<<buf;
buf=input.get();
}
input.close();
}
int main()
{
Caesar a;
int choice,key,ch;
char inp[30],out[30];
cout<<"\n\n 1. using file\n 2. Using keyboard input\n\n Select choice(1 or 2): ";
cin>>ch;
if(ch==1){
cout<<"\n Enter input file: ";
cin>>inp;
cout<<"\n Enter output file: ";
cin>>out;
cout<<"\n Enter key: ";
cin>>key;
cout<<"\n\n 1. Encrypt\n 2. Decrypt\n\n 3. exit.Select choice(1 or 2. Press 0 for exit): ";
cin>>choice;
do{
switch(choice){
case 1:
a.encrypt(inp,out,key);
break;
case 2:
a.decrypt(inp,out,key);
break;
case 3:
exit(0);
default: cout<<"\n\n Unknown choice";
}
}while(choice!=0);
}
else if(ch==2)
{
cout<<"\n\n 1. Encrypt\n 2. Decrypt\n\n Select choice(1 or 2): ";
cin>>choice;
do{
switch(choice){
case 1:
encrypt1();
break;
case 2:
decrypt1();
break;
default: cout<<"\n\n Unknown choice";
}
}while(choice!=0);
}
}
Question
The code above has an infinite loop when it is being executed in code blocks please fix it

Step by step
Solved in 2 steps

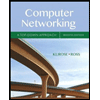
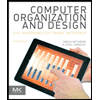
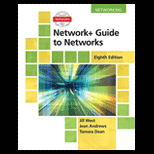
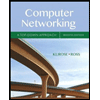
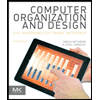
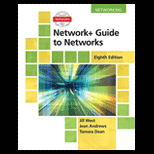
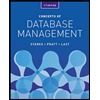
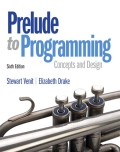
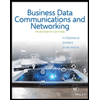