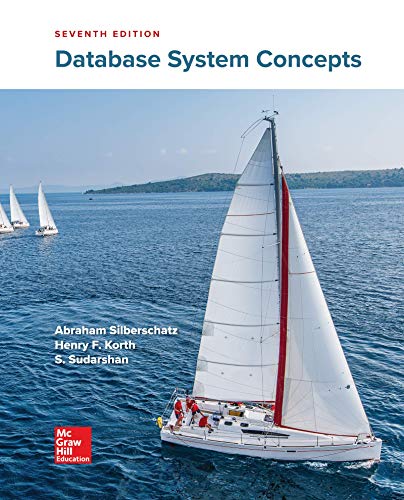
Concept explainers
convert it into file handling.
#include<iostream>
#include<conio.h>
#include<string>
#include<fstream>
using namespace std;
class votes
{
private:
int no_of_people = 0;
string arr[15] = { "Arif ur Rehman", "Waqar Chohan", "Sumaira Kausar", "Momina Moetesum","Muhammad Muzammal" };
int no[15] = { 77,56,55,44,36 };
public:
void work()
{
string name;
char selection;
do
{
cout << "\n Enter The name of Candidate you Like: ";
getline(cin, name);
char press;
for (int i = 0; i < 10; i++)
{
if (name == arr[0])
{
no_of_people = no[0];
}
else if (name == arr[1])
{
no_of_people = no[1];
}
else if (name == arr[2])
{
no_of_people = no[2];
}
else if (name == arr[3])
{
no_of_people = no[3];
}
else if (name == arr[4])
{
no_of_people = no[4];
}
else if (name == arr[5])
{
no_of_people = no[5];
}
if (name == arr[i])
{
cout << "\n The Candidate is in
cout << " Do you want to vote for him as well(y/n): ";
cin >> press;
if (press == 'y')
{
cout << endl;
no_of_people++;
}
else
{
goto jump;
}
cout << "\n\n Now Candidate is voted by " << no_of_people << " people.";
goto jump;
}
}
cout << "\n\n Candidate is not found in database Do you want to add it in database(y/n).";
char press1;
cin >> press1;
if (press1 == 'y')
{
arr[5].append(name);
cout << "\n\n Candidate added in database";
}
else
{
goto jump;
}
jump:
cout << "\n\n Do you want to look for another Candidate(y/n): ";
cin >> selection;
cin.ignore();
} while (selection != 'n');
}
void Total()
{
int total = 0;
for (int i = 0; i < 10; i = i + 1)
{
total = total + no[i];
}
cout << "\n\n The Total Number of People Who Have Alredy Voted : " << total;
}
void file()
{
ofstream file;
file.open("Data.txt");
if (!file)
{
cout << "\n File Not Open...\n\n";
exit(0);
}
else
{
for (int i = 0; i < 6; i = i + 1)
{
file << arr[i] << "," << no[i] << endl;
}
cout << "\n The file has written..";
}
file.close();
}
void display()
{
cout << "\n **";
cout << "\n The Top five Candidates are: " << endl;
for (int i = 0; i < 6; i++)
{
cout << "\n " << arr[i] << "," << no[i] << endl;
}
cout << "\n **";
}
};
int main()
{
votes v1;
v1.work();
v1.display();
v1.Total();
v1.file();
_getch();
system("pause");
}

Step by stepSolved in 3 steps

- Programming Problems doubleVowel Write a function doublevowel that accepts a word as an argument and returns True if the word contains two adjacent vowels and False otherwise. Sample usage: >>> doublevowel ('apple') False >>> doubleVowel ('pear') True >>> doubleVowel ('pear') True >>> doublevowe]('DURIAN') True >>> doublevowel ('baNaNa') False >>> doubleVowel ('baNaNa')== False Truearrow_forwardScala programmingarrow_forwardWrite a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forward
- Fix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forward#include <iostream> #include <iomanip> #include <string> using namespace std; struct Teletype { string name; string phonenum; Teletype *nextaddr; }; void populate(Teletype *); void displayrecord(Teletype *); //void insertrecord(Teletype *); // create //void removerecord(Teletype *); //create //void modifyrecord(Teletype *); // create //int find(TeleType *, string); // Extra Credit create bool check(); int main() { int location = 0; int count = 0; char answery_n; Teletype *list, *current; list = new Teletype; current = list; cout << "Please "; do { count++; populate(current); if (check() == false) { cout << " Not storage available" << endl; } else { current->nextaddr = new Teletype; current = current->nextaddr;…arrow_forward#include <iostream>#include <cstdlib>#include <time.h>#include <chrono> using namespace std::chrono;using namespace std; void randomVector(int vector[], int size){ for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; }} int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast<microseconds>(stop - start); cout << "Time taken by function: " << duration.count()…arrow_forward
- Create pseudocode for the following #include <iostream> #include <string> #include <cstdlib> #include <ctime> using namespace std; char water[10][10] = {{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'}}; void createBoard(int &numShip); void promptCoords(int& userX, int &userY); void shipGen(int shipX[] ,int shipY[], int &numShip); void testCoords(int &userX, int &userY, int shipX[], int shipY[], int &numShip, int& victory); void updateBoard();arrow_forward>> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forward3. int count(10); while(count >= 0) { count -= 2; std::cout << count << endl;arrow_forward
- In C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forward#include<stdio.h>#include<string.h>struct info{char names[100];int age;float wage;};int main(int argc, char* argv[]) {int line_count = 0;int index, i;struct info hr[10];if (argc != 2)printf("Invalid user_input!\n");else {FILE* contents = fopen (argv[1], "r");struct info in;if (contents != NULL) {printf("File has been opened successfully.\n\n");while (fscanf(contents, "%s %d %f\n",hr[line_count].names, &hr[line_count].age, &hr[line_count].wage) != EOF) {printf("%s %d %f\n", hr[line_count].names, hr[line_count].age, hr[line_count].wage);line_count++;}printf("\nTotal number of lines in document are: %d\n\n", line_count);fclose(contents);printf("Please enter a name: ");char user_input[100];fgets(user_input, 30, stdin);user_input[strlen(user_input)-1] = '\0';index = -1;for(i = 0; i < line_count; i++){if(strcmp(hr[i].names, user_input) == 0){index = i;break;}}if(index == -1)printf("Name %s not found\n", user_input);else{while(fread(&in, sizeof(struct info), 1,…arrow_forwardC++ program #include<iostream> #include<fstream> #include<string> using namespace std; const int NAME_SIZE = 20; const int STREET_SIZE = 30; const int CITY_SIZE = 20; const int STATE_CODE_SIZE = 3; struct Customers { long customerNumber; char name[NAME_SIZE]; char streetAddress_1[STREET_SIZE]; char streetAddress_2[STREET_SIZE]; char city[CITY_SIZE]; char state[STATE_CODE_SIZE]; int zipCode; char isDeleted; char newLine; }; void add_data(int no_of_records, ofstream& fout) { struct Customers c; cout << "Enter the Customer data:" << endl; cout << "Name:"; cin >> c.name; cout << "Street Address 1:"; cin >> c.streetAddress_1; cout << "Street Address 2:"; cin >> c.streetAddress_2; cout << "City:"; cin >> c.city; cout << "State:"; cin >> c.state; cout << "Zip Code:"; cin >> c.zipCode; cout << endl; c.customerNumber = no_of_records; c.isDeleted = 'N'; c.newLine = '\n'; cout…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
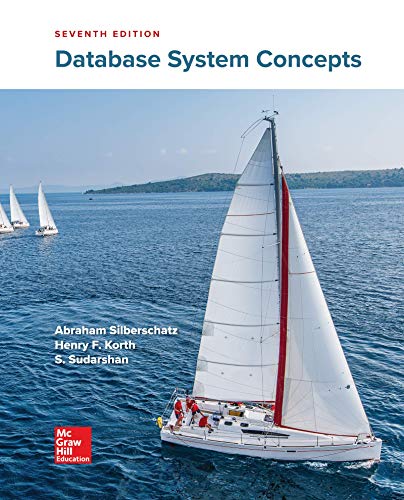
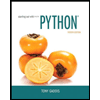
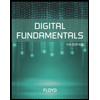
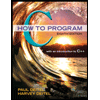
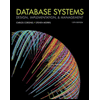
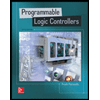