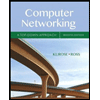
C
Down below is a menu-driven program about library operations. Help me because I don't know how to put an update and delete transactions. Also, please edit the program so it can have file manipulation/handling.
Required input:
CODE:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
struct book
{
int b_no;
char b_name[40];
char b_author[40];
int no_pg;
};
int main()
{
struct book b[20];
int ch,n,i,count = 0;
char temp[40];
do
{
printf("\n**************************************\n");
printf("\nMENU DRIVEN LIBRARY OPERATIONS PROGRAM\n");
printf("\n**************************************\n");
printf("\n\nCHOOSE FROM THE GIVEN OPTIONS BELOW:\n");
printf("\n--------------------------------------------\n");
printf("\nPRESS 1 - TO ADD BOOK DETAILS");
printf("\nPRESS 2 - TO DISPLAY BOOK DETAILS");
printf("\nPRESS 3 - TO SEARCH A BOOK/S OF A GIVEN AUTHOR");
printf("\nPRESS 4 - TO EXIT\n");
printf("\n--------------------------------------------\n");
printf("Enter Your Choice: ");
scanf("%d",&ch);
switch(ch)
{
case 1:
printf("\nHow many records you want to add? : ");
scanf("%d",&n);
printf("-------------------------------------\n");
printf("ADD BOOK/S DETAILS\n",n);
printf("-------------------------------------\n");
for(i = 0 ; i < n ; i++)
{
printf("Enter Book Number : ");
scanf("%d",&b[i].b_no);
printf("Book Name : ");
scanf("%s",b[i].b_name);
printf("Enter Author Name : ");
scanf("%s",b[i].b_author);
printf("Enter Number of Pages : ");
scanf("%d",&b[i].no_pg);
printf("-------------------------------------\n");
}
break;
case 2:
printf("\n\t\tDETAILS OF THE BOOK/S");
printf("\n-----------------------------------------------------------\n");
printf("Book No. Book Name\t Author Name\tNo. of Pages");
printf("\n------------------------------------------------------------");
for( i = 0 ; i < n ; i++)
{
printf("\n %d\t %s\t %s\t %d",b[i].b_no,b[i].b_name,b[i].b_author,b[i].no_pg);
}
printf("\n\n");
break;
case 3:
printf("-------------------------------------\n");
printf("SEARCH A BOOK/S OF GIVEN AUTHOR\n",n);
printf("-------------------------------------\n");
printf("\nEnter Author Name: ");
scanf("%s",temp);
printf("--------------------------------------");
for( i = 0 ; i < n ; i++)
{
if(strcmp(b[i].b_author,temp) == 0)
{
printf("\n%s\n",b[i].b_name);
}
}
break;
case 4 :
exit(0);
}
}while(ch != 4);
return 0;
}

Step by stepSolved in 2 steps

- Create pseudocode for the following #include <iostream> #include <string> #include <cstdlib> #include <ctime> using namespace std; char water[10][10] = {{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'}}; void createBoard(int &numShip); void promptCoords(int& userX, int &userY); void shipGen(int shipX[] ,int shipY[], int &numShip); void testCoords(int &userX, int &userY, int shipX[], int shipY[], int &numShip, int& victory); void updateBoard();arrow_forwardC++ PLEASE!! // FILE: simplestring.h// CLASS PROVIDED: string (a sequence of characters)//// CONSTRUCTOR for the string class:// string(const char str[ ] = "") -- default argument is the empty string.// Precondition: str is an ordinary null-terminated string.// Postcondition: The string contains the sequence of chars from str.//// CONSTANT MEMBER FUNCTIONS for the string class:// size_t length( ) const// Postcondition: The return value is the number of characters in the// string.//// char operator [ ](size_t position) const// Precondition: position < length( ).// Postcondition: The value returned is the character at the specified// position of the string. A string's positions start from 0 at the start// of the sequence and go up to length( )-1 at the right end.//// MODIFICATION MEMBER FUNCTIONS for the string class:// void operator +=(const string& addend)// Postcondition: addend has been catenated to the end of the string.//// void operator +=(const char addend[ ])//…arrow_forward#include<stdio.h>#include<string.h>struct info{char names[100];int age;float wage;};int main(int argc, char* argv[]) {int line_count = 0;int index, i;struct info hr[10];if (argc != 2)printf("Invalid user_input!\n");else {FILE* contents = fopen (argv[1], "r");struct info in;if (contents != NULL) {printf("File has been opened successfully.\n\n");while (fscanf(contents, "%s %d %f\n",hr[line_count].names, &hr[line_count].age, &hr[line_count].wage) != EOF) {printf("%s %d %f\n", hr[line_count].names, hr[line_count].age, hr[line_count].wage);line_count++;}printf("\nTotal number of lines in document are: %d\n\n", line_count);fclose(contents);printf("Please enter a name: ");char user_input[100];fgets(user_input, 30, stdin);user_input[strlen(user_input)-1] = '\0';index = -1;for(i = 0; i < line_count; i++){if(strcmp(hr[i].names, user_input) == 0){index = i;break;}}if(index == -1)printf("Name %s not found\n", user_input);else{while(fread(&in, sizeof(struct info), 1,…arrow_forward
- C# program in Visual Studio Code. I need to be able to enter five integer values and then have the Unique values entered display. Private member variable to store 5 unique values (hint: use an Array or a List) Public function to get 5 unique values from the user and store them in the member variable loop to get the numbers, if a number is already stored, ignore it and keep looping until you have 5 unique numbers if a number is out of range, don't store the value, throw an exception and handle it in such a way that you don't break the loop but do message the user that the value was out of rangearrow_forwardinitial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forwardC++main.cc file #include <iostream>#include <map>#include <vector> #include "bank.h" int main() { // =================== YOUR CODE HERE =================== // 1. Create a Bank object, name it anything you'd like :) // ======================================================= // =================== YOUR CODE HERE =================== // 2. Create 3 new accounts in your bank. // * The 1st account should belong to "Tuffy", with // a balance of $121.00 // * The 2nd account should belong to "Frank", with // a balance of $1234.56 // * The 3nd account should belong to "Oreo", with // a balance of $140.12 // ======================================================= // =================== YOUR CODE HERE =================== // 3. Deactivate Tuffy's account. // ======================================================= // =================== YOUR CODE HERE =================== // 4. Call DisplayBalances to print out all *active* // account…arrow_forward
- C++arrow_forwardc++ code Screenshot and code is mustarrow_forward# dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forward
- struct Info { int id; float cost; }; Given the struct above, the following code segment will correctly declare and initialize an instance of that struct. Info myInfo = {10,4.99}; Group of answer choices True False ============== Consider the following code fragment. int a[] = {3, -5, 7, 12, 9}; int *ptr = a; *ptr = (*ptr) + 1; cout << *ptr; Group of answer choices 4 -5 3 Address of -5 =============== What will happen if I use new without delete? Group of answer choices The program will never compile The program will never run The program will run fine without memory leak None of thesearrow_forwardin C code not c++arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
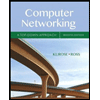
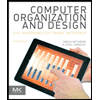
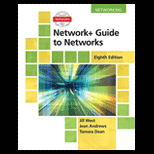
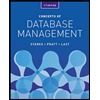
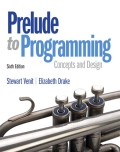
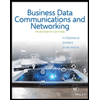