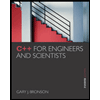
PLleasae convert this in C language, thanks!
...
#include <iostream>
using namespace std;
int main()
{
int a[10][10], b[10][10], s[10][10];
int i,j,row, column;
cout <<"Enter size of row:";
cin >> row;
cout <<"Enter size of column:";
cin >> column;
cout <<"Enter elements of matrix A" << endl;
for(i=0;i<row;i++)
{
for(j=0;j<column;j++)
{
cin >> a[i][j];
}
}
cout <<"Enter elements of matrix B" << endl;
for(i=0;i<row;i++)
{
for(j=0;j<column;j++)
{
cin >> b[i][j];
}
}
cout << "Difference of A and B" << endl;
for(i=0;i<row;i++)
{
for(j=0;j<column;j++)
{
cout << a[i][j] - b[i][j] << " ";
}
cout << endl;
}
getch();
return 0;
}

Step by stepSolved in 3 steps with 1 images

- (File creation) Write a C++ program that creates an array containing the integer numbers 60, 40, 80, 90, 120, 150, 130, 160, 170, and 200. Your program should then write the data in the array to a text file. (Alternatively, you can create the file with a text editor.)arrow_forward(Data processing) Construct a three-dimensional weather array for a two-week time period. Include this array in a C++ program that displays the temperatures correctly in response to any of the following user requests: • Any day’s high and low temperatures • Average high and low temperatures for a given month • Month and day with the highest temperature • Month and day with the lowest temperaturearrow_forward(Conversion) Write a C++ program that converts gallons to liters. The program should display gallons from 10 to 20 in 1-gallon increments and the corresponding liter equivalents. Use the relationship that 1gallon=3.785liters.arrow_forward
- (Data processing) Your professor has asked you to write a C++ program that determines grades at the end of the semester. For each student, identified by an integer number between 1 and 60, four exam grades must be kept, and two final grade averages must be computed. The first grade average is simply the average of all four grades. The second grade average is computed by weighting the four grades as follows: The first grade gets a weight of 0.2, the second grade gets a weight of 0.3, the third grade gets a weight of 0.3, and the fourth grade gets a weight of 0.2. That is, the final grade is computed as follows: 0.2grade1+0.3grade2+0.3grade3+0.2grade4 Using this information, construct a 60-by-7 two-dimensional array, in which the first column is used for the student number, the next four columns for the grades, and the last two columns for the computed final grades. The program’s output should be a display of the data in the completed array. For testing purposes, the professor has provided the following data:arrow_forward(Electrical eng.) a. An engineer has constructed a two-dimensional array of real numbers with three rows and five columns. This array currently contains test voltages of an amplifier. Write a C++ program that interactively inputs 15 array values, and then determines the total number of voltages in these ranges: less than 60, greater than or equal to 60 and less than 70, greater than or equal to 70 and less than 80, greater than or equal to 80 and less than 90, and greater than or equal to 90. b. Entering 15 voltages each time the program written for Exercise 7a runs is cumbersome. What method could be used for initializing the array during the testing phase? c. How might the program you wrote for Exercise 7a be modified to include the case of no voltage being present? That is, what voltage could be used to indicate an invalid voltage, and how would your program have to be modified to exclude counting such a voltage?arrow_forward(Conversion) Write a C++ program to convert kilometers/hr to miles/hr. The program should produce a table of 10 conversions, starting at 60 km/hr and incremented by 5 km/hr. The display should have appropriate headings and list each km/hr and its equivalent miles/hr value. Use the relationship that 1 kilometer=0.6241miles.arrow_forward
- Mark the following statements as true or false. A double type is an example of a simple data type. (1) A one-dimensional array is an example of a structured data type. (1) The size of an array is determined at compile time. (1,6) Given the declaration: int list[10]; the statement: list[5] - list[3] * list[2]; updates the content of the fifth component of the array list. (2) If an array index goes out of bounds, the program always terminates in an error. (3) The only aggregate operations allowable on int arrays are the increment and decrement operations. (5) Arrays can be passed as parameters to a function either by value or by reference. (6) A function can return a value of type array. (6) In C++, some aggregate operations are allowed for strings. (11,12,13) The declaration: char name [16] = "John K. Miller"; declares name to be an array of 15 characters because the string "John K. Miller" has only 14 characters. (11) The declaration: char str = "Sunny Day"; declares str to be a string of an unspecified length. (11) As parameters, two-dimensional arrays are passed either by value or by reference. (15,16)arrow_forward(Numerical) Write and test a function that returns the position of the largest and smallest values in an array of double-precision numbers.arrow_forward(Electrical eng.) Write a program that declares three one-dimensional arrays named volts, current, and resistance. Each array should be declared in main() and be capable of holding 10 double-precision numbers. The numbers to store in current are 10.62, 14.89, 13.21, 16.55, 18.62, 9.47, 6.58, 18.32, 12.15, and 3.98. The numbers to store in resistance are 4, 8.5, 6, 7.35, 9, 15.3, 3, 5.4, 2.9, and 4.8. Your program should pass these three arrays to a function named calc_volts(), which should calculate elements in the volts array as the product of the corresponding elements in the current and resistance arrays (for example ,volts[1]=current[1]resistance[1]). After calc_volts() has passed values to the volts array, the values in the array should be displayed from inside main().arrow_forward
- (Data processing) Write a C++ program that reads the file created in Exercise 4, permits the user to change the hourly wage or years for any employee, and creates a new updated file.arrow_forward(Civil eng.) Write a C++ program to calculate and display the maximum bending moment, M, of a beam that’s supported on both ends (see Figure 3.8). The formula is M=XW(LX)/L, where X is the distance from the end of the beam that a weight, W, is placed, and L is the beam’s length. You program should produce this display: The maximum bending moment is xxxx.xxxx The xxxx.xxxx denotes placing the calculated value in a field wide enough for four places to the right and left of the decimal point. For your program, assign the values1.2,1.3,and11.2toX,W,andL.arrow_forwardWhen you perform arithmetic operations with operands of different types, such as adding an int and a float, ____________. C# chooses a unifying type for the result you must choose a unifying type for the result you must provide a cast you receive an error messagearrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
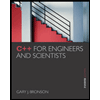
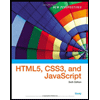
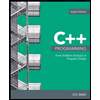
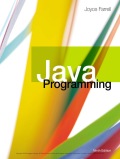
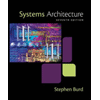