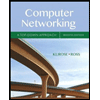
I keep getting these errors when I run the code below: Code is in C++
____animal.cpp_______
#include "animal.h"
Animal::Animal()
{
}
Animal::Animal(string name,string gender, string color, double weight)
{
this->name = name;
this->gender = gender;
this->color = color;
this->weight = weight;
}
void Animal::PrintInfo()
{
cout<<"Name : "<<name<<endl;
cout<<"Gender : "<<gender<<endl;
cout<<"Color : "<<color<<endl;
cout<<"Weight : "<<weight<<endl;
}
__animal.h___
#ifndef __ANIMAL_H
#define __ANIMAL_H
#include<iostream>
using namespace std;
class Animal
{
private:
double weight;
string name;
string gender;
string color;
public:
Animal();
Animal(string,string , string, double);
virtual void PrintInfo();
};
#endif
___horse.h___
#ifndef __HORSE_H
#define __HORSE_H
#include "animal.h"
class Horse : public Animal
{
private:
string breed;
int id;
string comments;
public:
Horse();
Horse(string,double,string,string,int,string,string);
void PrintInfo();
};
#endif
____horse.cpp____
#include "horse.h"
Horse::Horse()
{
}
Horse::Horse(string breed, double weight , string name ,string gender, int id, string color , string comments):Animal(name,gender,color,weight)
{
this->breed = breed;
this->id = id;
this->comments = comments;
}
void Horse::PrintInfo()
{
Animal::PrintInfo();
cout<<"Breed : "<<this->breed<<endl;
cout<<"Id : "<<this->id<<endl;
cout<<"Comments : "<<this->comments<<endl;
}
____ horsemain.cpp_____
#include "horse.h"
#include<limits>
#include<cstdlib>
void clearBuffer()
{
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(),'\n');
}
int main()
{
int size = 5;
Horse *snakes = new Horse[5];
string breed;
double weight;
string name;
string gender;
int id;
string color ;
string comments;
for(int i = 0;i<size;i++)
{
cout<<"\nEnter Breed : ";
getline(cin,breed);
cout<<"Enter Weight : ";
cin >> weight;
clearBuffer();
cout<<"Enter Name : ";
getline(cin,name);
cout<<"Enter Gender : ";
getline(cin,gender);
cout<<"Enter Id : ";
cin >> id;
clearBuffer();
cout<<"Enter Color : ";
getline(cin,color);
cout<<"Enter Comments : ";
getline(cin,comments);
snakes[i] = Horse(breed,weight,name,gender,id,color,comments);;
}
for(int i = 0;i<size;i++)
{
Animal *animal;
animal = &snakes[i];
cout<<"\n";
animal->PrintInfo();
}
delete[] snakes;
return 0;
}
![## Understanding Compiling Errors in C Programming
### Error Message Transcript:
```
/usr/bin/make -j8 -e -f Makefile
---------Building project:[ animal.h - Debug ]---------
/usr/bin/g++ -o Debug/animal.h @"animal.h.txt" -L.
Undefined symbols for architecture x86_64:
"_main", referenced from:
implicit entry/start for main executable
ld: symbol(s) not found for architecture x86_64
clang: error: linker command failed with exit code 1 (use -v to see invocation)
make[1]: *** [Debug/animal.h] Error 1
make: *** [All] Error 2
=== build ended with errors (1 errors, 0 warnings) ===
```
### Explanation:
This error output occurs when attempting to compile a project using the `make` build tool in a C or C++ environment. Here's a breakdown of the error and potential solutions:
- **Undefined Symbols**: The error message indicates "Undefined symbols for architecture x86_64." This typically means the compiler is looking for the `main` function, which is the entry point of any C or C++ program.
- **Implicit Entry**: The message "_main, referenced from: implicit entry/start for main executable" means that the `main` function is missing or not correctly linked. In C/C++ programs, `main` is essential as it is the starting point of execution.
- **Linker Command Failed**: The error "linker command failed with exit code 1" signifies that the linker could not complete its task, which is often due to missing symbols or incorrect paths to libraries or source files.
- **Makefile Error**: The `make` tool exits with errors denoted by "make: *** [All] Error 2", indicating that the build process cannot proceed because of the errors encountered.
### Solutions:
1. **Ensure Main Function Exists**: Verify that there is a `main` function in your source files. If your project is a library without a `main` function, adjust your build settings accordingly.
2. **Check File Extensions**: Ensure that you're compiling source files (like `.cpp` or `.c`), not header files (`.h`) which typically don't contain a `main` function.
3. **Linking Libraries**: Make sure all necessary libraries are correctly linked. Check your `Makefile` or](https://content.bartleby.com/qna-images/question/7b9451f1-a2f9-47cb-b8b6-654ac5ea1321/23100457-ea04-4575-8fd7-5d35c94417af/iqv22om_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C++ Programming The expression (6-2)*(5+3)/3 evaluates to ___________. Write a c++ expression for .____________________ What is the process of manually converting an int to a double called? _______________ Write a statement that prints the value of 3.0/7.0 to 4 decimal places. ____________________________ The ____________ iomanip operator sets the width of the field for the << operator. Write a statement that computes the square root of a squared plus b squareddouble a = 5.0; double b = 12.0;double result = _______________________________ What include file is needed for the code in the previous question? _______________ The ___________________ library function computes the tangent of an angle. The _________ binary relational operator tests if two operands are equal. The _________ unary relational operator inverts the truth value of a boolean expression. True or false: x >= y is the same as x > y && x == y? _________________ In the following code, for what values of…arrow_forwardC# i need to Write a program named SortWords that includes a method named SortAndDisplayWords that accepts any number of words, sorts them in alphabetical order, and displays the sorted words separated by spaces. Write a Main() to test this function. The SortAndDisplayWords method will be tested with one, two, five, and ten words. Method SortAndDisplayWords sorts and displays one word Method SortAndDisplayWords sorts and displays two words Method SortAndDisplayWords sorts and displays ten wordsMethod SortAndDisplayWords sorts and displays five words using System; using static System.Console; public class SortWords { public static void Main() { // Write your main here } public static void SortAndDisplayWords(params string[] words) { } }arrow_forwardC++ Write a function named “createPurchaseOrder” that accepts the quantity(integer), the cost per item (double), and the description (string). It will return anewly created PurchaseOrder object holding that information if they are valid:quantity and cost per item cannot be negative and the description cannot be empty or blank(s). When it is invalid, it will return NULL to indicate that it cannot create such PurchaseOrder object. PurchaseOrder createPurchaseOrder(int quantity, double costPerItem, string description) { if(quantity < 0 || costPerItem < 0.0 || description == "") return NULL; else { PurchaseOrder p = new PurchaseOrder(quantity,costPerItem,description); return p; } } what's the issue with this function and how to rewrite itarrow_forward
- C++ coding project just need some help getting the code thank you!arrow_forwardC++YOU ONLY NEED TO EDIT date.h, dateOFYear.h, and dateOFYear.cpp date.h | date.cpp | dateOfYear.h | dateOfYear.cpp | dateTest.cpp You have been provided a Date class. Each date contains day and month parameters, a default and a custom constructor, mutator and accessor functions for the parameters, and input and output functions called within operator >> and <<. You will be writing a DateOfYear class, which expands on the Date class by adding a year parameter. This will be achieved via inheritance – DateOfYear is the derived class of the Date base class. In DateOfYear, will need to write… The include guards for the class A default constructor that initializes all parameters to 1 A custom constructor that takes in a month, day, and year to initialize the parameters Mutator and accessor for the year parameter Redefined versions of inputDate and outputDate that takes into account the new year parameter. The driver program, dateTest.cpp, is already set for use with both Date…arrow_forwardhere's the 3rd. Vehicle.cpp // Vehicle.cpp #include <iostream> using namespace std; class Vehicle { public: void setSpeed(double); double getSpeed(); void accelerate(double); void setFuelCapacity(double); double getFuelCapacity(); void setMaxSpeed(double); double getMaxSpeed(); private: double fuelCapacity; double maxSpeed; double currentSpeed; }; void Vehicle::setSpeed(double speed) { currentSpeed = speed; return; } double Vehicle::getSpeed() { return currentSpeed; } void Vehicle::accelerate(double mph) { if(currentSpeed + mph < maxSpeed) currentSpeed = currentSpeed + mph; else cout << "This vehicle cannot go that fast." << endl; } void Vehicle::setFuelCapacity(double fuel) { fuelCapacity = fuel; } double Vehicle::getFuelCapacity() { return fuelCapacity; } void Vehicle::setMaxSpeed(double max) { maxSpeed = max; } double Vehicle::getMaxSpeed() {…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
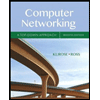
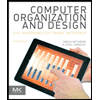
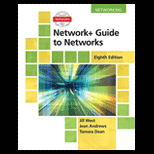
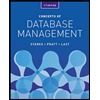
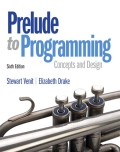
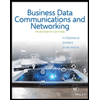