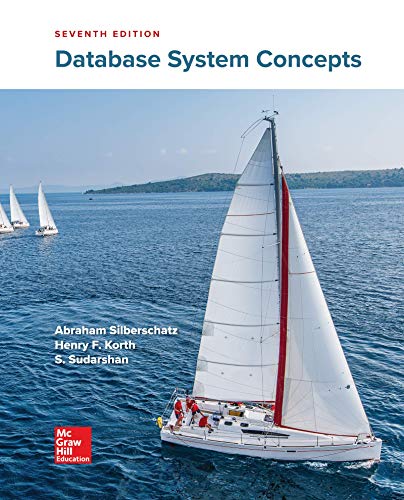
I need help for Microsoft Visual C#, 7th edition, chapter 5 debugging exercise 5-2
//
// If the stock number is not 209, 312, or 414 the user must reenter the number
// The program displays the correct price
using System;
using static System.Console;
using System.Globalization;
class DebugFive2
{
static void Main()
{
const string ITEM209 = "209";
const string ITEM312 = "312";
const string ITEM414 = "414";
const double PRICE209 = 12.99, PRICE312 = 16.77, PRICE414 = 109.07;
double price;
string stockNum;
Write("Please enter the stock number of the item you want ");
stockNum = ReadLine();
while(stockNum != ITEM209 || stockNum != ITEM312 || stockNum != ITEM414)
{
WriteLine("Invalid stock number. Please enter again. ");
stockNum = ReadLine();
}
if(stockNum == ITEM209)
price = PRICE209;
else
if(stockNum == ITEM312)
price = PRICE312;
else
price = PRICE414;
WriteLine("The price for item # {0} is {1}}", stockNum, price.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C++ Help me fix my code please! I'm missing something and can't figure it out. Code and error picture are below. #include <iostream> using namespace std; class Employee{ public: string first_name; string last_name; int monthly_sal; int increment; Employee() { first_name=""; last_name=""; monthly_sal=0; increment=0; } void setFirst_Name(string first_name) { this->first_name=first_name; } void setLast_Name(string last_name) { this->last_name=last_name; } void setSal(int monthly_sal) { if(monthly_sal<0) this->monthly_sal=0; this->monthly_sal=monthly_sal; } void setInc(int increment) { monthly_sal += increment; } string getFirst_Name() { return first_name; } string getLast_Name() { return last_name; } int get_salary() { return monthly_sal; } int get_increment() { return increment;…arrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardI need help with this PLEASE NO JAVA NO C++ ONLY PYTHON PLZ Create a class object with the following attributes and actions: Class Name Class number Classroom Semester Level Subject Actions: Store a class list Print the class list as follows: Class name Class Number Semester Level Subject Test your object: Ask the user for all the information and to enter at least 3 classes test using all the actions of the object print using the to string action Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.arrow_forward
- C# and I half to use what's given below using System; using static System.Console; using System.Globalization; class GreenvilleRevenue { static void Main() { // Your code here } } In Chapter 2, you created an interactive application named GreenvilleRevenue. The program prompts a user for the number of contestants entered in this year’s and last year’s Greenville Idol competition, and then it displays the revenue expected for this year’s competition if each contestant pays a $25 entrance fee. The programs also display a statement that compares the number of contestants each year. Using the program you wrote in Case Study 1 of Chapter 2, replace that statement with one of the following messages: If the competition has more than twice as many contestants as last year, display The competition is more than twice as big this year! If the competition is bigger than last year’s but not more than twice as big, display The competition is bigger than ever! If the…arrow_forwardstructions: Write the following programs in C# using concepts learnt in this chapter and abmit the .cs file with the screenshot of your output for each question in the Lab Assignment 2 Submission page. 1. FRENCH TRANSLATOR Look at the following list of French words and their meanings: In French: gauche milieu droite middle In English: right left Create an application that translates the French words to English. The form should have three buttons, one for each French word. When the user clicks a button, the application should display the English translation in a Label control.arrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- Flight assignment runs but there is no imput values for the user to interact when running the program. Kindly fix this issue.import java.util.*; class Flight { String airlineCode; int flightNumber; char type; // D for domestic, I for international String departureAirportCode; String departureGate; char departureDay; int departureTime; String arrivalAirportCode; String arrivalGate; char arrivalDay; int arrivalTime; char status; // S for scheduled, A for arrived, D for departed public Flight(String airlineCode, int flightNumber, char type, String departureAirportCode, String departureGate, char departureDay, int departureTime, String arrivalAirportCode, String arrivalGate, char arrivalDay, int arrivalTime) { this.airlineCode = airlineCode; this.flightNumber = flightNumber; this.type = type; this.departureAirportCode = departureAirportCode; this.departureGate = departureGate;…arrow_forwardtranlate source program in the picture into quadruples tranlate source program in the picture into quadruples tranlate source program in the picture into quadruplesarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
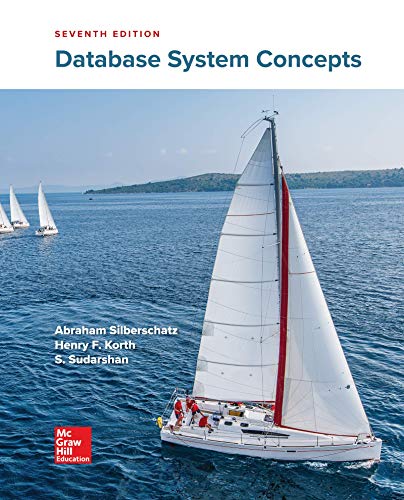
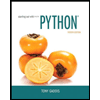
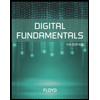
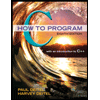
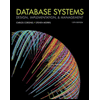
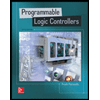