Complete function RollSpecific Number() that has three parameters: a GVDie struct object, an integer representing a desired face number of a die, and an integer representing the goal amount of times to roll the desired face number. The function RollSpecific Number() then rolls the die until the desired face number is rolled the goal amount of times and returns the number of rolls required. Note: For testing purposes, a GVDie struct object is created in the main() function using a pseudo-random number generator with a fixed seed value. The program uses a seed value of 15 during development, but when submitted, a different seed value will be used for each test case. Ex: If a GVDie struct object is created with a seed value of 15 and the input of the program is: 3 20 the output is: It took 140 rolls to get a "3" 20 times.
Complete function RollSpecific Number() that has three parameters: a GVDie struct object, an integer representing a desired face number of a die, and an integer representing the goal amount of times to roll the desired face number. The function RollSpecific Number() then rolls the die until the desired face number is rolled the goal amount of times and returns the number of rolls required. Note: For testing purposes, a GVDie struct object is created in the main() function using a pseudo-random number generator with a fixed seed value. The program uses a seed value of 15 during development, but when submitted, a different seed value will be used for each test case. Ex: If a GVDie struct object is created with a seed value of 15 and the input of the program is: 3 20 the output is: It took 140 rolls to get a "3" 20 times.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
>> IN C PROGRAMMING LANGUAGE ONLY <<
COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "GVDie.h"
int RollSpecificNumber(GVDie die, int num, int goal) {
/* Type your code here. */
}
int main() {
GVDie die = InitGVDie(); // Create a GVDie variable
die = SetSeed(15, die); // Set the GVDie variable with seed value 15
int num;
int goal;
int rolls;
scanf("%d", &num);
scanf("%d", &goal);
rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.
printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal);
return 0;
}

Transcribed Image Text:9.18 LAB: Rolling for X
Complete function RollSpecificNumber() that has three parameters: a GVDie struct object, an integer representing a
desired face number of a die, and an integer representing the goal amount of times to roll the desired face number.
The function RollSpecificNumber() then rolls the die until the desired face number is rolled the goal amount of times
and returns the number of rolls required.
Note: For testing purposes, a GVDie struct object is created in the main() function using a pseudo-random number
generator with a fixed seed value. The program uses a seed value of 15 during development, but when submitted, a
different seed value will be used for each test case.
Ex: If a GVDie struct object is created with a seed value of 15 and the input of the program is:
3 20
the output is:
It took 140 rolls to get a "3" 20 times.
457462.2786122.qx3zqy7
?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
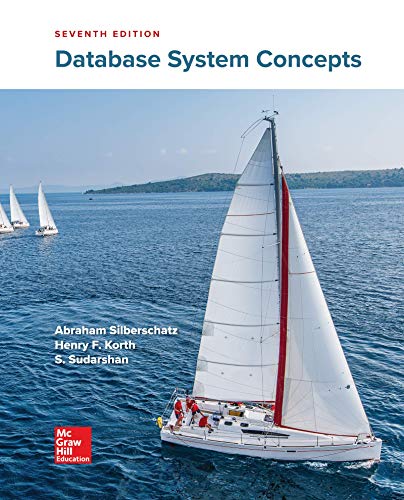
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
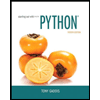
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
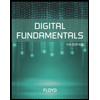
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
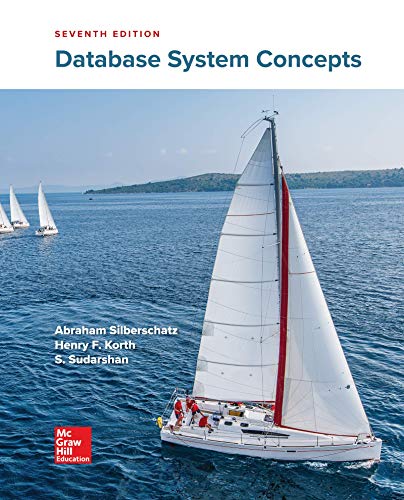
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
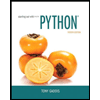
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
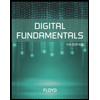
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
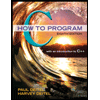
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
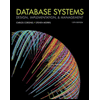
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
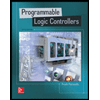
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education