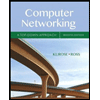
Given two integers as user inputs that represent the number of drinks to buy and the number of bottles to restock, create a VendingMachine object that performs the following operations:
- Purchases input number of drinks
- Restocks input number of bottles
- Reports inventory
#include <iostream>
#include "VendingMachine.h"
using namespace std;int main() {
VendingMachine.h
/* Type your code here */
}#ifndef VENDINGMACHINE_H_
#define VENDINGMACHINE_H_#include <iostream>
using namespace std;class VendingMachine {
public:
VendingMachine();
void Purchase(int amount);
int GetInventory();
void Restock(int amount);
void Report();private:
int bottles;
};#endif
VendingMachine.cpp#include <iostream>
#include <string>#include "VendingMachine.h"
using namespace std;
VendingMachine::VendingMachine() {
bottles = 20;
}void VendingMachine::Purchase(int amount) {
bottles = bottles - amount;
}int VendingMachine::GetInventory() {
return bottles;
}
void VendingMachine::Restock(int amount) {
bottles = bottles + amount;
}// set the random number generator seed for testing
void VendingMachine::Report() {
cout << "Inventory: " << bottles << " bottles" << endl;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- #include <iostream> #include <iomanip> #include <string> using namespace std; int main() { constdouble MONSTERA_PRICE =11.50; constdouble PHILODENDRON_PRICE =13.75; constdouble HOYA_PRICE =10.99; constint MAX_POTS =20; constdouble POINTS_PER_DOLLAR =1.0/0.75; char plantType; int quantity; double totalAmount =0.0; int totalPoints =0; int availablePots =0; double plantPrice =0.0; cout << "Welcome to Tom's Plant Shop!" << endl; cout << "******************************" << endl; cout << "Enter your full name: "; string fullName; getline(cin, fullName); output: compiling code... Welcome to Tom's Plant Shop!----------------------------------------Enter your full name: ______ Available plants:M - Monstera ($ 11.5)P - Philodendron ($13.75)H - Hoya ($10.99)Q - Quit shoppingEnter the plant type (M/P/H/Q): ________ Part 1 Tom has recently started a plant-selling business, and he offers three different types of plants, namely Monstera,…arrow_forward// EmployeeBonus2.cpp - This program calculates an employee's yearly bonus. #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is…arrow_forwardPlease written by computer source Unit 4 - Lab – Individual Retirement Account Calculation Assignment An Individual Retirement Account (IRA) is a special bank / brokerage account that a person can use to save money for retirement. An individual can put up to $5,500 per year tax free and the money grows tax free until the person is ready to retire. The tax rules related to IRA’s are complex, but for this assignment, we’ll assume that the person puts in the same amount of money every year (up to $5,500) and the money grows at a constant interest rate. Write a Flowgorithm program that asks the user to input an annual IRA contribution (in dollars), and interest rate (percent), and a time period (years). Calculate the value of the IRA every year for the duration of the time period provided by the user. Be sure and provide user friendly prompts. Include a comment at the beginning of the program with your name, date, and a short description of the program.arrow_forward
- main.cc file #include #include #include #include "cup.h" int main() { std::string drink_name; double amount = 0.0; std::cout << "What kind of drink can I get you?: "; std::getline(std::cin, drink_name); while (std::cout << "How much do you want to fill?: " && !(std::cin >> amount)) { // If the input is invalid, clear it, and ask again std::cin.clear(); std::cin.ignore(); std::cout << "Invalid input; please re-enter.\n"; } //================== YOUR CODE HERE ================== // Instantiate a `Cup` object named `mug`, with // the drink_name and amount given by the user above. //==================================================== while (true) { char menu_input = 'X'; std::cout << "\n=== Your cup currently has " << mug.GetFluidOz() << " oz. of " << mug.GetDrinkType() << " === \n\n"; std::cout << "Please select what you want to do with…arrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
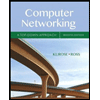
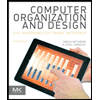
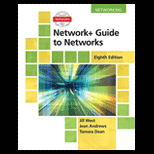
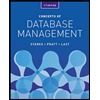
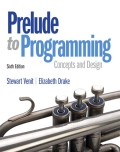
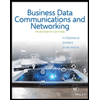