Download the file Insect.cpp(mentioned below) and use this as your start file. Inside the file you will see the implementation of the class Insect. Do the following: a. Write a class BumbleBee that inherits from Insect. Add the public member function void sting() const . This function simply prints "sting!" and a newline. b. Write a class GrassHopper that inherits from Insect. Add the public member function void hop() const . This function simply prints "hop!" and a newline. When you are done your program output should look exactly like the output provided at the end of the file. insect.cpp #include using namespace std; // Insect class declaration class Insect { protected: int antennae; int legs; int eyes; public: // default constructor Insect(); // getters int getAntennae() const; int getLegs() const; int getEyes() const; }; // BumbleBee class declaration // GrassHopper class declaration // main int main() { BumbleBee bumble; GrassHopper hopper; cout << "A bumble bee has " << bumble.getLegs() << " legs and can "; bumble.sting(); cout << endl; cout << "A grass hopper has " << hopper.getLegs() << " legs and can "; hopper.hop(); return 0; } // member function definitions Insect::Insect() { antennae = 2; eyes = 2; legs = 6; } int Insect::getAntennae() const { return antennae; } int Insect::getLegs() const { return legs; } int Insect::getEyes() const { return eyes; } /* A bumble bee has 6 legs and can sting! A grass hopper has 6 legs and can hop! */ Do not modify the main program. Do not modify the Insect class.
Download the file Insect.cpp(mentioned below) and use this as your start file. Inside the file you will see the implementation of the class Insect. Do the following:
a. Write a class BumbleBee that inherits from Insect. Add the public member function void sting() const . This function simply prints "sting!" and a newline.
b. Write a class GrassHopper that inherits from Insect. Add the public member function void hop() const . This function simply prints "hop!" and a newline.
When you are done your program output should look exactly like the output provided at the end of the file.
insect.cpp
#include <iostream>
using namespace std;
// Insect class declaration
class Insect
{
protected:
int antennae;
int legs;
int eyes;
public:
// default constructor
Insect();
// getters
int getAntennae() const;
int getLegs() const;
int getEyes() const;
};
// BumbleBee class declaration
// GrassHopper class declaration
// main
int main()
{
BumbleBee bumble;
GrassHopper hopper;
cout << "A bumble bee has " << bumble.getLegs() << " legs and can ";
bumble.sting();
cout << endl;
cout << "A grass hopper has "
<< hopper.getLegs() << " legs and can ";
hopper.hop();
return 0;
}
// member function definitions
Insect::Insect()
{
antennae = 2;
eyes = 2;
legs = 6;
}
int Insect::getAntennae() const
{ return antennae; }
int Insect::getLegs() const
{ return legs; }
int Insect::getEyes() const
{ return eyes; }
/*
A bumble bee has 6 legs and can sting!
A grass hopper has 6 legs and can hop!
*/
Do not modify the main program. Do not modify the Insect class.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

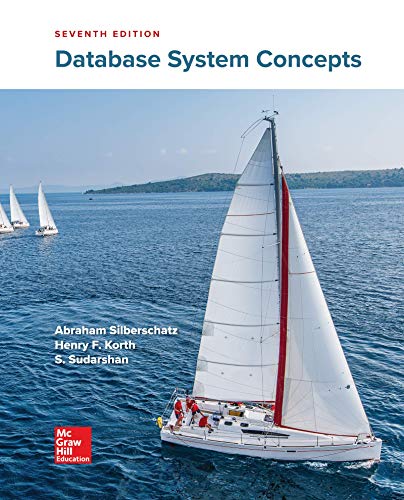
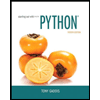
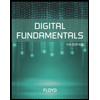
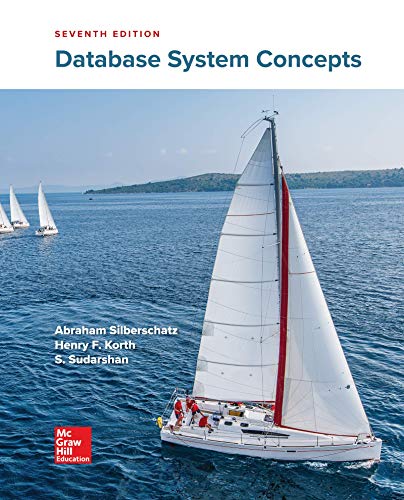
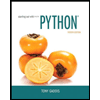
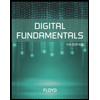
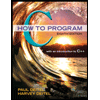
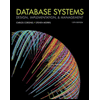
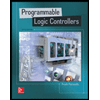