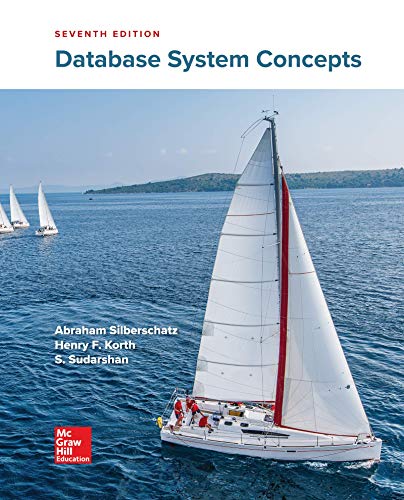
Concept explainers
Help, I making a elevator simulator, I need help in writing code in the Elevator class and it's polymorphism class. I am not sure what to do next. Any help is appreciated.
There are 4 types of passengers in the system:
Standard: This is the most common type of passenger and has a request percentage of 70%.
Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority
and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large
items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items
that need to be transported and are more likely to be picked up by glass elevators.
There are 4 types of elevators in the system:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a
maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a
maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a
maximum capacity of 6 passengers and are designed to transport fragile item
This is all I could think of
import java.util.Random;
public abstract class Passenger {
public static int passengerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger() {
this.passengerID = "" + passengerCounter;
passengerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
class StandardPassenger extends Passenger {
public StandardPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
class VIPPassenger extends Passenger {
public VIPPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
// Request an express elevator
int expressElevator = settings.getNofloors() / 2;
this.startFloor = expressElevator;
this.endFloor = expressElevator;
return true;
}
}
class FreightPassenger extends Passenger {
public FreightPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
class GlassPassenger extends Passenger {
public GlassPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
public abstract class Elevator{
}
public class StandardElevator extends Elevator{
}
public class ExpressElevator extends Elevator{
}
public class FreightElevator extends Elevator{
}
public class GlassElevator extends Elevator{
}

Step by stepSolved in 4 steps with 3 images

- The goal of this coding exercise is to create two classes BookstoreBook and LibraryBook. Both classes have these attributes: author: Stringtiltle: Stringisbn : String- The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like 20% off...etc). For a LibraryBook, we add the call number (that tells you where the book is in the library) as a string. The call number is automatically generated by the following procedure:The call number is a string with the format xx.yyy.c, where xx is the floor number that is randomly assigned (our library has 99 floors), yyy are the first three letters of the author’s name (we assume that all names are at least three letters long), and c is the last character of the isbn.- In each of the classes, add the setters, the getters, at least three constructors (of your choosing) and override the toString method (see samplerun…arrow_forwardOverride the testOverriding() method in the Triangle class. Make it print “This is theoverridden testOverriding() method”. (add it to triangle class don't make a new code based on testOverriding in SimpleGeometricObject class.) (this was the step before this one) Add a void testOverriding() method to the SimpleGeometricObject class. Make it print“This is the testOverriding() method of the SimpleGeometricObject class”arrow_forwardFor this lab task, you will work with classes and objects. Create a class named text that works similar to the built-in string class. You will need to define a constructor that can be used to initialize objects of this new class with some literal text. Next, define three methods: to_upper() that will convert all characters to uppercase, reverse() that will reverse the text and length() that will return the length of the text. After you have completed this part, copy the following mainfunction to your code and check if the implementation is correct. int main() { text sample = "This is a sample text"; cout << sample.to_upper(); // This should display "THIS IS A SAMPLE TEXT" cout << endl;cout << sample.reverse(); // This should display "txet elpmas a si sihT"cout << endl; cout << sample.length(); // This should display 21 }arrow_forward
- Below for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate…arrow_forwardJAVA QUESTION REQUIRES SEPARATE CLASSES, CANNOT BE WRITTEN IN ONE MAIN METHOD E.G. ACCOUNT SECTION WRITTEN IN ACCOUNT CLASS, CUSTOMER SECTION WRITTEN IN CUSTOMER CLASS ETC. Phase 2 In this phase you’ll be writing code within the methods of your classes to make the tests pass. Account Each account should have a constant, unique id. Id numbers should start from 1000 and increment by 5. The ACCOUNT_ID attribute should be initialised when the account object is created. The id should be generated internally within the class, it should not be passed in as an argument. The deposit method should increase the balance by the value passed in as an argument. The withdraw method should reduce the balance by the value passed in as an argument. It should return the value of the argument The account should be able to go overdrawn The correctBalance method should change the balance to match the value passed in as an argument. Testing your code for Account Run TestAccountInitialisation to check…arrow_forwardUsing classes, design an online address book to keep track of the names, addresses, phone numbers, and birthdays of family members, close friends and certain business associates. Your program should be able to handle a maximum of 10 entries. For that, (10) • Define the class Address that can store a street address, city, state, and zip code. Use the appropriate methods to print and store the address. Also, use constructors to automatically initialize the data members. Define the class Person and its attributes. Add a data member to this class to classify the person as a family member, friend, or business associate. Also, add a data member to store the phone number. You must Add (or override) methods to print and store the appropriate information. Use constructors to automatically initialize the data members. • Now, Define the class AddressBook using previously defined classes. The program should perform the following operations: o Load the data into the address book. Search for a…arrow_forward
- is this just for the candidate class or is the election class included?arrow_forwardCan I get a help with this in Java please? Introduce a new class, called Borrower to the project. Its purpose is to represent the borrower of the CD. It should have two fields surname and libraryId; where the latter is a mix of letters and numbers, and a suitable constructor with parameters for only these two fields in the order specified above as well as appropriate accessor methods.arrow_forwarduse Pyhton. The tossball simulation we developed together in class is attached to this week's notes. Study it carefully to understand exactly what it is doing. It currently launches a ball in the main graphics window. You need to make the following changes and improvements and send me at the end your finished project in zipped folder format. Only python modules should be included. 1) Add a new class named "Cannon". This class it to represent the cannon that launches the balls. It should draw a rectangle (hint: use Polygon class from Graphics.py) at the launch point. Its width should be equal to the diameter of the balls that it shoots out and its length should be proportional to the speed, say something like speed * 0.15. The cannon should also be directed in the same direction that the balls shooting. Add proper width, height, and angle attributes to the class for supporting the settings above. The balls should start from the bottom of the cannon and be projected out through the…arrow_forward
- Can comments be added to describe the functionality of each class/method and any other important detail worth knowing about? Also, does the program follow standard practices of modularity? Thank youarrow_forwardI have provided you with 3 interfaces (HasLegs, HasWings, and BreathesUnderwater) and a Tester class. I want you to create 6 new classes: • 2 that implement HasLegs • 2 that implement HasWings • 2 that implement BreathesUnderwater For each of the classes, you will have to implement the methods that the interface dictates. In addition, override toString so that it prints out a simple message describing what the object is. See the example output of my implementation below for an idea how to do that. The Tester class creates 3 ArrayLists, one for each interface. It also loops through each ArrayList and prints out its contents using toString and the interface methods. In between those two chunks of code, you’ll see a //TODO: comment. It is there that I want you to create one object for each of the 6 classes you created and add that object to the appropriate ArrayList. Once you have done all that, you should be able to run your program and see output like the following: I am a table. I have…arrow_forwardHelp, I making a elevator simulator. Can someone please help me improve this code I have. The remaining code is in the pictures. Any help is appreciated. Thank You! The simulation should have 4 types of Passengers: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. The simulation should also have 4 types of Elevators: StandardElevator: This is the most common type of elevator…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
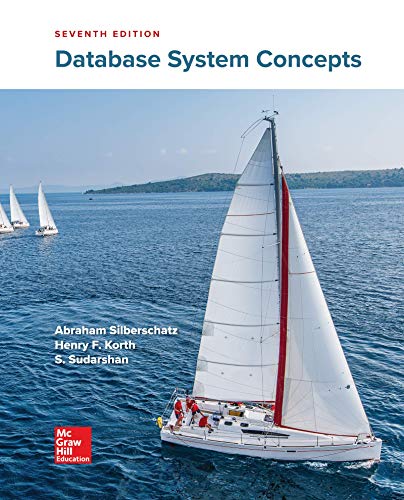
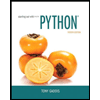
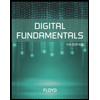
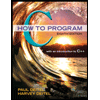
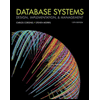
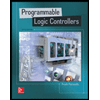