TODO 10 Now it's your turn to define an entire class. Complete the TODO by creating the LogTransformer class. Define the LogTransformer class by following the below steps: Create a class called LogTransformer. Make sure this class inherits BaseEstimator and TransformerMixin. Note: as we don't need the constructor method __init__() we don't have to define it. Define the fit() method. Be sure it takes the arguments self, X, and y=None just like we did above. Further, make sure to return self otherwise you will get errors. Define the transform() method which takes the arguments self, X, and y=None just like we did above. In the transform() method, return the log of the input data X. Be sure you add 1 to the data before computing the log as it is not possible to take ???(0)! # TODO 10.1 log = LogTransformer() log_area_df = log.fit_transform(forestfire_df['area']) display(log_area_df) todo_check([ (log_area_df.shape == (517,), 'log_area_df does not have the shape (517,)'), (np.all(np.isclose(log_area_df.values[-5:], np.array([2.00687085, 4.01259206, 2.49815188, 0. , 0. ]),rtol=.01)), 'log_area_df did not contain the correct values!') ])
TODO 10 Now it's your turn to define an entire class. Complete the TODO by creating the LogTransformer class. Define the LogTransformer class by following the below steps: Create a class called LogTransformer. Make sure this class inherits BaseEstimator and TransformerMixin. Note: as we don't need the constructor method __init__() we don't have to define it. Define the fit() method. Be sure it takes the arguments self, X, and y=None just like we did above. Further, make sure to return self otherwise you will get errors. Define the transform() method which takes the arguments self, X, and y=None just like we did above. In the transform() method, return the log of the input data X. Be sure you add 1 to the data before computing the log as it is not possible to take ???(0)! # TODO 10.1 log = LogTransformer() log_area_df = log.fit_transform(forestfire_df['area']) display(log_area_df) todo_check([ (log_area_df.shape == (517,), 'log_area_df does not have the shape (517,)'), (np.all(np.isclose(log_area_df.values[-5:], np.array([2.00687085, 4.01259206, 2.49815188, 0. , 0. ]),rtol=.01)), 'log_area_df did not contain the correct values!') ])
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
TODO 10
Now it's your turn to define an entire class. Complete the TODO by creating the LogTransformer class.
- Define the LogTransformer class by following the below steps:
- Create a class called LogTransformer. Make sure this class inherits BaseEstimator and TransformerMixin.
- Note: as we don't need the constructor method __init__() we don't have to define it.
- Define the fit() method. Be sure it takes the arguments self, X, and y=None just like we did above. Further, make sure to return self otherwise you will get errors.
- Define the transform() method which takes the arguments self, X, and y=None just like we did above.
- In the transform() method, return the log of the input data X. Be sure you add 1 to the data before computing the log as it is not possible to take ???(0)!
- Create a class called LogTransformer. Make sure this class inherits BaseEstimator and TransformerMixin.
# TODO 10.1
log = LogTransformer()
log_area_df = log.fit_transform(forestfire_df['area'])
display(log_area_df)
todo_check([
(log_area_df.shape == (517,), 'log_area_df does not have the shape (517,)'),
(np.all(np.isclose(log_area_df.values[-5:], np.array([2.00687085, 4.01259206, 2.49815188, 0. , 0. ]),rtol=.01)), 'log_area_df did not contain the correct values!')
])
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
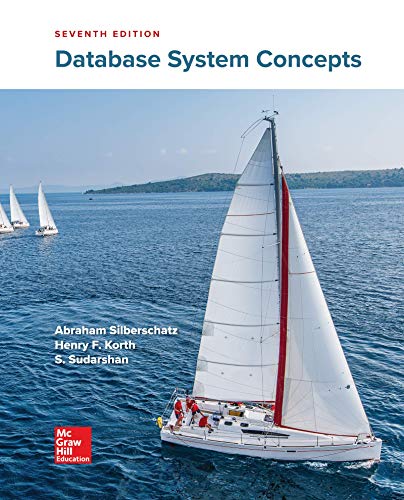
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
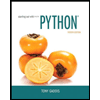
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
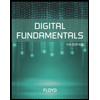
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
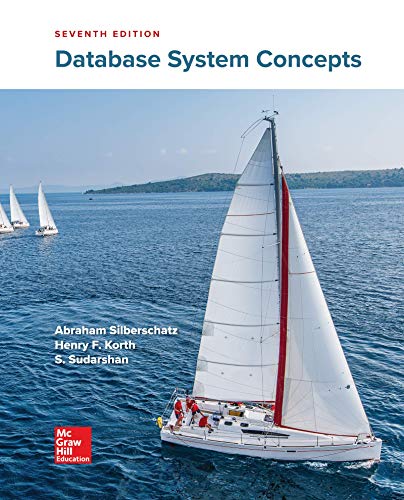
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
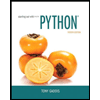
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
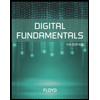
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
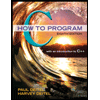
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
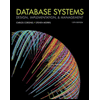
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
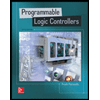
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education