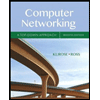
Concept explainers
For this task, you are to complete a program which manages a movie collection.
Instructions
The movie collection program found below is currently missing two important pieces of functionality---it doesn't add movies to the collection, nor is it able to print the longest movies in the collection.
Provided for you is the Movie class which contains the title and duration for a particular movie, and the MovieCollection class which is responsible for maintaining a collection of movies. Your task is to implement missing functionality by a) completing the interactive loop, and b) defining a print_longest_movies method on the MovieCollection class.
a) Completing the interactive loop
To complete the interactive loop, you must instantiate a Movie object using the information provided by the user and add it to the MovieCollection object using the provided add_movie method.
b) Defining print_longest_movies
This method is to take no arguments and print the title and duration of the top three longest movies in descending order (i.e. highest to lowest). Additionally, each movie should be numbered in the output (i.e. the longest movie is 1, the second longest is 2, etc). Here's an example of output which could be produced when print_longest_movies is called:
1. Titanic (194 minutes)2. Vertigo (128 minutes)
3. Jaws (124 minutes)
In order to sort the movies you should call the sort list method (documented here). You will need to make use of the sort method's two named arguments when calling it: key and reverse:
-
The key named argument can be provided with the name of a function (no parentheses). That function will be called for each item, and sorting will be based on the values returned by the function.
-
The reverse named argument can be provided with a boolean which determines whether the sort order should be reversed or not.
Hint: A function which returns the duration of a movie has already been defined for you, and can be used as the key named argument for sort.
Requirements
To achieve full marks for this task, you must follow the instructions above when writing your solution. Additionally, your solution must adhere to the following requirements:
- You must use the sort list method with appropriate named arguments to sort movies in descending order of duration.
- You must make appropriate use of a loop to print the longest movies.
- You must not use a return, break, or continue statement in print_longest_movies.
- You must limit the number of movies printed to three. If there are fewer than three movies in the collection, all of them should be printed.
Example Runs
Run 1 (more than three movies)
Movie title (or blank to finish): VertigoMovie duration (minutes): 128
Movie title (or blank to finish): Titanic
Movie duration (minutes): 194
Movie title (or blank to finish): Rocky
Movie duration (minutes): 120
Movie title (or blank to finish): Jaws
Movie duration (minutes): 124
Movie title (or blank to finish):
= Longest movies in the collection =
1. Titanic (194 minutes)
2. Vertigo (128 minutes)
3. Jaws (124 minutes)
Run 2 (fewer than three movies)
Movie title (or blank to finish): BraveheartMovie duration (minutes): 178
Movie title (or blank to finish):
= Longest movies in the collection =
1. Braveheart (178 minutes)
Your code should execute as closely as possible to the example runs above. To check for correctness, ensure that your program gives the same outputs as in the examples, as well as trying it with other inputs.

Step by stepSolved in 2 steps with 3 images

- Write a C#program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string) ClassRegistration -…arrow_forwardIn Python, Create a CourseGrades application that simulates a grade book for a class with 12 students that each have 5 test scores. The CourseGrades application should use a GradeBook class that has member variablesgrades, which is a two-dimensional list or integers, and a function getGrades() for prompting the user for the test grades for each student, showGrades() that displays the grades for the class, studentAvg()that has a student number parameter and then returns the average grade for that student, and testAvg() that has a test number parameter and then returns the average grade for that test.arrow_forwardMemoryManagerFirstFit The MemoryManagerFirstFit class is derived from the MemoryManagerBase class. Based on the TODO entries in memory_manager_first_fit.h and memory_manager_first_fit.cpp, implement the functions as instructed. memory_manager_first_fit.cpp #include "memory_manager_first_fit.h" #include using namespace std; MemoryManagerFirstFit::MemoryManagerFirstFit(int size) { // Set up the list of memory blocks with the info for this initial // unallocated block. memory_block b; // TODO: Fill in the appropriate values here based on the data elements // you added in the header file. // Add the block to the list of blocks m_blocks.push_back(b); } // Allocate a block of memory of the given size // We will walk through our current list of blocks and // find the block with the first fit. int MemoryManagerFirstFit::allocate(int size, string name) { // TODO: Implement the allocate logic here // // Iterate through the current list of blocks // and find the first one that is big enough…arrow_forward
- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardJava programming This problem set will test your knowledge of System I/O, and variable assignment. Your task is to create several different java classes (.java files) that will produce a specific output based on the user input. All input will be given to you either as a command-line argument or typed in from the keyboard. Below you will find directions for each class you need to create. Please make sure that the class name and java file name match the name 1, ContainsAnyCase This program will accept two user inputted values that are strings. The first user input will be a single word the second user input will be a sentence of random words. This program should print "true" if the first word is in the sentence of words regardless of the casing. In other words case is ignored when checking if the word is within the sentence. The last string that this program should print is "true" or "false", nothing else. 2 PrintMathResult Write an application that will wait for three user inputted…arrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forward
- C++ Assignment---This is an unfinished code please complete it. Make changes if necessary. Upvote for finished working code. Objectives • To apply knowledge of classes and objects Instructions Implement all of the following, each in a separate Visual Studio project (and/or solution). 1. Define a class called CounterType to implement a simple counter. The class must have a private field (data member) counter of type int. You must also provide methods (member functions), including the following: a. A constructor to set the counter to the value specified by the user b. A constructor to set the counter to 0 c. A method to allow the user to set the counter to a value they specify d. A method to increment the counter by 1 e. A method to decrement the counter by 1 Your code must ensure the value of counter is never negative. If the user calls a method to attempt to make the counter negative, set the counter to 0 and print out an error to the user (e.g., “The counter must not be negative.”) Do…arrow_forwardProblem Statement: Develop an Inventory Management System (IMS) for a small retail business that allows the user to manage their product inventory. The system should enable the user to add, edit, update, and delete product information stored in a .csv file. The IMS should be console-based with a menu-driven interface. Requirements: 1. Classes and Objects: - Create a `Product` class with attributes such as `productID`, `productName`, `price`, `quantity`. - Implement a `InventoryManager` class that will handle operations like adding, editing, updating, and deleting products. - Use a `Main` class with the `main` method to run the program and display the menu. 2. File Operations: - Store product information in a .csv file named `inventory.csv`. - Implement methods in `InventoryManager` for reading and writing to the .csv file. 3. Menu-Driven Interface: - Implement a menu in the `Main` class that allows the user to select operations like Add, Edit, Update, Delete, and View Inventory. - Use…arrow_forwardPLEASE help with the following C#.NET using the following class make a driver class which outputs the patient health record Write a driver class (app) that prompts for the person’s data input, instantiates an object of class HeartRates and displays the patient’s information from that object by calling the DisplayPatientRecord, method. essentially make a main method which asks the user to manually enter the data using System;namespace A1Question2{public class HealthProfile{ // attibutes which holds the following valueprivate String _FirstName;private String _LastName;private int _BirthYear;private double _Height;private double _Weigth;private int _CurrentYear;public HealthProfile(string firstName, string lastName, int birthYear, double height, double wt, int currentYear){_FirstName = firstName;_LastName = lastName;_BirthYear = birthYear;_Height = height;_Weigth = wt;_CurrentYear = currentYear;}public string firstName { get; set; }public string lastName { get; set; }public int birthYear…arrow_forward
- In C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forwardPLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forwardHi, I am having trouble with this homework question for my C++ course 1. Implement a Student class. a. Create a class Student with the following private data members:1. name2. exam_1 grade3. exam_2 gradeb. Create all appropriate accessor and mutator functions.c. Assign appropriate access modifiers to insure encapsulation.d. Add a private calc GPA() function that calculates and returns the GPAbased upon the two exam grades.e. Add a public getGrade() function that: 1. Obtains the GPA from the private calc GPA() function.2. Returns a letter grade based upon the numerical GPA value.90 to 100 = A80 to 90 = B70 to 80 = C60 to 70 = D0 to 60 = F f. Test all functions use following main.int main(){Student a;a.setName("David");a.setExam1(90);a.setExam2(80);cout<<a.getName()<<endl;cout<<a.getExam1()<<endl;cout<<a.getExam2()<<endl;cout<<a.getGrade()<<endl;}arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
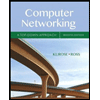
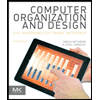
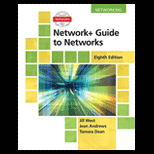
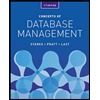
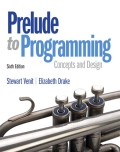
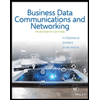