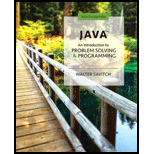
Explanation of Solution
Creating a class “RationalAssert.java”:
- Import required package.
- Define the class “RationalAssert”.
- Declare the private variables “the_numerator”, and “the_denominator”.
- Define parameterized constructor.
- Assign “numerator” to “the_numerator”.
- Assign “denominator” to “the_denominator”.
- Create an assertion with the condition “assert the_denominator != 0”.
- Give mutator methods to set numerator and denominator.
- Give accessor methods to get the value of numerator and denominator.
- Give function definition for “value ()”.
- Return the value produced by “the_numerator / the_denominator”.
- Given function “toString ()”.
- Return the string “the_numerator / the_denominator”.
- Define the “main ()” method.
- Create an object for “Scanner” class.
- Declare the variables “n”, and “d”.
- Get the numerator and denominator from the user.
- Call the constructor by passing “n”, and “d”.
- Call the function “value”.
- Call the function to get the numerator and denominator.
- Print new line.
Program:
Rational.java:
import java.util.Scanner;
//Define a class
public class RationalAssert
{
//Declare required variables
private int the_numerator;
private int the_denominator;
//Parameterized constructor
public RationalAssert(int numerator, int denominator)
{
//Assign the value of numerator
the_numerator = numerator;
//Assign the value of denominator
the_denominator = denominator;
//Declare an assertion
assert the_denominator != 0;
}
//Function to set the value of numerator
public void setNumerator(int numerator)
{
//Set the value
the_numerator = numerator;
}
//Function to set the denominator
public void setDenominator(int denominator)
{
//Declare an assertion
assert the_denominator != 0;
//Assign the value
the_denominator = denominator;
}
�...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Write a C++ class and choose any name for it. The class should have two privatemembers and two public members. Write a constructor to initialize these membersto zero. Write another constructor to initialize these members to non-zero values.Write a method to return the average of the class members. Write a C++ programand initialize the members of the class with [2,5,8,3] respectively and call its methodto calculate the average.arrow_forward16. Create a class SubstitutionCipher that implements the interface MessageEncoder, as described in the previous exercise. The constructor should have one parameter called shift. Define the method encode so that each letter is shifted by the value in shift. For example, if shift is 3, a will be replaced by d, b will be replaced by e, c will be replaced by f, and so on. (Hint: You may wish to define a private method that shifts a single character.)arrow_forward*python coding please Write a class named Person with data attributes for a person’s name, address, and telephone number. Next, write a class named Customer that is a subclass of the Person class. The Customer class should have a data attribute for a customer numberand a Boolean data attribute indicating whether the customer wishes to be on a mailing list. Demonstrate an instance of the Customer class in a simple program.arrow_forward
- Write C# equivalent statements for the following: An “Account” class will be created having properties: accountName, accountNumber, accountType, totalBalance and methods: WithdrawAmount(), CheckBlance(), DepositAmount(). Derive child classes, that is, “SavingAccount” and “CurrentAccount” from the Account class and implement the following functionality. While taking input from user, check whether the account type is Saving or Current. If user enters “Saving”, an object of Saving Account will be instantiated and while depositing amount in the account an extra 3% of the amount will be deposited (as an extra profit) with the actual amount entered by user. If user enters “Current”, an object of Current Account will be instantiated and while depositing amount in the account, no extra amount will be deposited. Only the actual amount will be deposited. Also implement all the methods mentioned above.arrow_forwardJava Define the class InvalidSideException, which inherits from the Exception class. Also define a Square class, which has one method variable -- an int describing the side length. The constructor of the Square class should take one argument, an int meant to initialize the side length; however, if the argument is not greater than 0, the constructor should throw an InvalidSideError. The Square class should also have a method getArea(), which returns the area of the square.Create a Driver class with a main method to test your classes. Your program should prompt the user to enter a value for the side length, and then create a Square object with that side length. If the side length is valid, the program should print the area of the square. Otherwise, it should catch the InvalidExceptionError, print "Side length must be greater than 0.", and terminate the program. Standard output: Enter·side·length·of·square:16↵ 256↵arrow_forwardJava Define the class InvalidSideException, which inherits from the Exception class. Also define a Square class, which has one method variable -- an int describing the side length. The constructor of the Square class should take one argument, an int meant to initialize the side length; however, if the argument is not greater than 0, the constructor should throw an InvalidSideError. The Square class should also have a method getArea(), which returns the area of the square.Create a Driver class with a main method to test your classes. Your program should prompt the user to enter a value for the side length, and then create a Square object with that side length. If the side length is valid, the program should print the area of the square. Otherwise, it should catch the InvalidExceptionError, print "Side length must be greater than 0.", and terminate the program.arrow_forward
- Write a Java operator/keyword, which can be used to check the validity of reference before attempting down-casting from superclass object to subclass reference. Given an example of it usage. (Write in maximum 5 lines) Assume you have a method expecting the superclass's object to be passed as an argument. In this case, if you give it an object of subclass rather than superclass. Is it acceptable? Why or why not? (Write in maximum 5 lines)arrow_forwardIn c++ ( without empty constructor)Create a lecturer class with member-data name (name) and department (department). For the Lecturer class, write constructors, get & set methods, and the toString () method, which returns the textual description of the object. Write a Lecture class with data member:- subject (discipline)- weekday (day of the week when it takes place)- hour (start time, integer)- online (boolean value, whether remote or not)- lecturer (object of class Lecturer)For the Lecture class, write:- constructors- get & set methods- method isItMorning (), returning a Boolean value, the result of checking whether the lecture starts at 7-11- give a new implementation of the toString () method so that it returns the text description of the Lecture objectIn the test function main ()1. Create objects of both classes and illustrate the use of all possible methods for them.2. Create an array of Lecture objects and display it on the screen and in a file.arrow_forwardPrompt We have learned how to use accessor and mutator methods to access private class member data. It is possible to instead make the class members public, which would allow other programs to directly retrieve and modify the class member data without needing to write the accessor and mutator methods. This is usually considered bad practice. Why do you think that is? What do you think the benefits are to writing accessor and mutator methods instead of just leaving the variables public? Below is the definition for a class called Counter. Define a new method for this class called "findDifference". This method should take another Counter object as an argument and return the difference in the counts between the counter being called and the one passed as an argument. The difference should be given as an absolute value (not returned as a negative). See below the class definition for examples of this method being used. public class Counter {private int count;public Counter() {count =…arrow_forward
- Answer with True or False for the following. Explain your answers.-It is legal to write a method in a class which overloads another method declared in the same.-It is legal to write a method in a superclass which overrides a method declared in a sub-class.arrow_forwardPls answer part a and b accordingly. Read this first: SHOW ALL YOUR WORK. PROGRAM SEGMENTS ARE TO BE WRITTEN IN JAVA. Assume that the classes listed in the Java Quick Reference have been imported where appropriate. Unless otherwise noted in the question, assume that parameters in method calls are not null and that methods are called only when their preconditions are satisfied. In writing solutions for each question, you may use any of the accessible methods that are listed in classes defined in that question. You shouldn't write significant amounts of code that can be replaced by a call to one of these methods . A manufacturer wants to keep track of the average of the ratings that have been submitted for an item using a running average. The algorithm for calculating a running average differs from the standard algorithm for calculating an average, as described in part (a). A partial declaration of the RunningAverage class is shown below. You will write two methods of the…arrow_forwardObserve the following Java statement and the answer the question. Choose ONE that is true. System.out.println(circleArea = super.calArea()); a. This statement may exist in classes that are not related by inheritance b. The use of super will cause Java to raise exception. It should be replaced with an object. c. The returned value from the superclass method named calArea() is displayed d. None of the abovearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
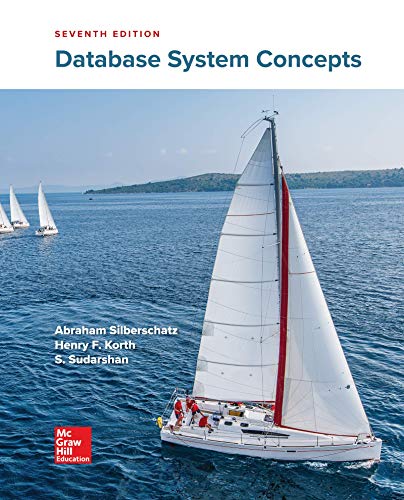
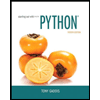
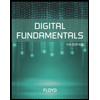
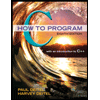
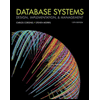
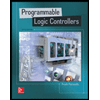