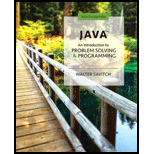
Write a class LapTimer that can be used to time the laps in a race. The class should have the following private attributes:
- running — a boolean indication of whether the timer is running
- startTime — the time when the timer started
- lapStart — the timer’s value when the current lap started
- lapTime — the elapsed time for the last lap
- totalTime — the total time from the start of the race through the last completed lap
- lapsCompleted — the number of laps completed so far
- lapsInRace — the number of laps in the race
The class should have the following methods:
- LapTimer (n) — a constructor for a race having n laps.
- start — starts the timer. Throws an exception if the race has already started.
- markLap — marks the end of the current lap and the start of a new lap. Throws an exception if the race is finished.
- getLapTime — returns the time of the last lap. Throws an exception if the first lap has not yet been completed.
- getTotalTime — returns the total time from the start of the race through the last completed lap. Throws an exception if the first lap has not yet been completed.
- getLapsRemaining — returns the number of laps yet to be completed, including the current one.
Express all times in seconds.
To get the current time in milliseconds from some baseline date, invoke
Calendar.getInstance ().getTimeInMillis ()
This invocation returns a primitive value of type long. By taking the difference between the returned values of two invocations at two different times, you will know the elapsed time in milliseconds between the invocations. Note that the class Calendar is in the package java.util.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Problem Solving with C++ (10th Edition)
Programming in C
Starting Out With Visual Basic (7th Edition)
Modern Database Management (12th Edition)
- class Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forwardsolve with java Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having…arrow_forwardEvery attribute and method declared in a class has an access modifier such as private, public and protected. Differentiate each of them.arrow_forward
- A small airline company is developing software to control its operations. You should design and develop a class that will be used to create objects that hold information about flights. The information kept about each flight includes: ● Flight number • Departure time • Seat capacity Seats sold Flight destination Flight departure point (i.e. Dublin, Cork, etc.) When creating a flight object the flight number, departure time, seat capacity, flight destination and departure point are given. Behaviour Functions of this class: • Default Constructor - initialises all data members. Constructor that takes the flight no as input parameter and request user input, departure time, seat capacity, flight destination, flight departure point, flight time. ● • A check_available_seats function that returns the number of unsold (still available) seats on a flight; • A sellseats function that takes as a parameter the number of seats a customer requires on a flight. If there are sufficient seats available…arrow_forwardVariables declared globally in an instantiable class are also known as all of these terms except: O fields O class variables O properties O instance variablesarrow_forwardUsing JAVASCRIPT write an object prototype for a Person that has a name and age, has a printInfo method, and also has a method that increments the persons age by 1 each time the method is called. Create two people using the 'new' keyword, and print both of their infos and increment one persons age by 3 years. Use an arrow function for both methods */ // Create our Person Prototype // Use an arrow to create the printInfo method // Create another arrow function for the addAge method that takes a single parameter // Adding to the agearrow_forward
- Assignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date createdarrow_forwardWrite the Circle class:• A Circle has a radius – Add instance variable – 2 constructors – one should be the default or no args – Add accessor and mutator method for each instance variable – Add methods to calculate Area, Perimeter, and Diameter TestCircle Create a Circle with the radius Print the radius using the getters Print the Circle’s area, circumference, and diameter Change the circle’s radius Reprint the informationarrow_forwardClass: Square Create a class called "Square", which is inherited from“TwoDimensionShape” class. The "Square" class is used to calculate theArea and the Perimeter of square shapes when their side-length is given, or in contrast,finding the side-length of the square when the Area or the Perimeter of the square is given.The specifications of this class are below. Data Members (Attributes): All are private. sideLength: the sidelight (L) of the square. Methods/Operations/Getters/Setters: Default Constructor: when creating an object this constructor must set the side-length of the square by calling the method setSideLength(). Also, this constructor willcall the findArea() and the findPerimeter() in addition to summaryPrint() to calculateand print all needed information. User-Defined Constructor: when creating an object this constructor shouldfind the side-length of the square by calling the method findSquareSideLength()and pass the Area or the…arrow_forward
- Library Information System Design and Testing Library Item Class Design and TestingDesign a class that holds the Library Item Information, with item name, author, publisher. Write appropriate accessor and mutator methods. Also, write a tester program(Console and GUI Program) that creates three instances/objects of the Library Items class. Extending Library Item Class Library and Book Classes: Extend the Library Item class in (1) with a Book class with data attributes for a book’s title, author, publisher and an additional attributes as number of pages, and a Boolean data attribute indicating whether there is both hard copy as well as eBook version of the book. Demonstrate Book Class in a Tester Program (Console and GUI Program) with an object of Book class.arrow_forwardThe Point2D should store an x and y coordinate pair, and will be used to build a new class via class composition. A Point2D has a x and a y, while a LineSegment has a start point and an end point (both of which are represented as Point2Ds). class Invariants The start and end points of a line segment should never be null Initialize these to the origin instead. Data A LineSegment has a start point This is a Point2D object All data will be private A LineSegment also has an end point. Also a Point2D object Methods Create getters and setters for your start and end points public Point2D getStartPoint() { public void setStartPoint(Point2D start) { Create a toString() function to build a string composed of the startPoint’s toString() and endPoint’s toString() Should look like “Line start(0,0) and end(1,1)” Create an equals method that determines if two LineSegments are equal public boolean equals(Object other) { if(other == null || !(other instanceof LineSegment)) return…arrow_forwardObject adapters and class adapters each provide a unique function. These concepts are also significant due to the significance that you attach to them.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
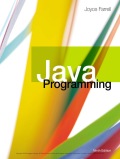
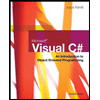