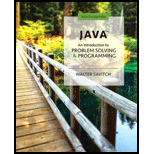
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 9, Problem 3PP
Program Plan Intro
Date format converter
Program plan:
“DateFormatConverter.java”:
- Import required packages.
- Define the class “DateFormatConverter”.
- Declare required variables.
- Define an array and assign it with the months.
- Define the “main()” method.
- Do until the user enters other than “Y” or “y”.
- Inside the “try” block,
- Get the date from the user.
- Trim the input.
- Store the month value in a variable.
- Check if that value is less than 0.
-
- Assign “false” to “validInput”.
- Throw an exception.
- Check if month value is less than 1 or greater than 12.
-
- Assign “false” to “validInput”.
- Throw an exception.
- Otherwise,
-
- Get the date value and store it in a variable.
- Call the function “dayCheck()”.
- Catch “MonthException”.
- Print the error message.
- Check if the function “validInput()” returns true.
- Print the month name and its date.
- Get the response from the user whether the user wants to do it again or not.
- Inside the “try” block,
- Do until the user enters other than “Y” or “y”.
- Give function to convert string to integer for month.
- Get the position of “/”.
- Check if that position is equal to 2.
- Return the value.
- Check if that position is equal to 1.
- Return the integer value.
- Otherwise,
- Return -1.
- Give function to convert string to integer for day.
- Get the number of characters.
- Check if that number is equal to 2.
- Return the value.
- Check if that number is equal to 1.
- Return the integer value.
- Otherwise,
- Return -1.
- Function definition to convert ASCII value to integer.
- Switch to the digit.
- Assign the value according to the character digit.
- Switch to the digit.
- Give function definition to check the day.
- Inside the “try” block,
- Switch to the month number.
- If the month number is 1, 3, 5, 7, 8, 10 and 12,
-
- Check if the day is less than 1 or greater than 31.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 31.
- If the month number is 4, 6, 9 and 11,
-
- Check if the day is less than 1 or greater than 30.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 30.
- If the month number is 2,
-
- Check if the day is less than 1 or greater than 29.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 29.
- Switch to the month number.
- Inside the “catch” block for “DayException”, print the error message.
- Inside the “try” block,
“DayException.java”:
- Define the exception class “DayException”.
- Define a default constructor.
- Call the parent class’s method.
- Define two parameterized constructors.
- Call the parent class’s method by passing a message.
- Define a default constructor.
“MonthException.java”:
- Define the exception class “MonthException”.
- Define a default constructor.
- Call the parent class’s method.
- Define two parameterized constructors.
- Call the parent class’s method by passing a message.
- Define a default constructor.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
in C# i need to
Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. For example, an error message:
For Goodnight Moon, ratio is invalid. ...Price is $12.99 for 25 pages.
Next, create a Book class that contains fields for title, author, price, and number of pages. Include properties for each field. Throw a BookException if a client program tries to construct a Book object for which the price is more than 10 cents per page.
Finally, using the Book class, create an array of five Books. Prompt the user for values for each Book. To handle any exceptions that are thrown because of improper or invalid data entered by the user, set the Book’s…
Write a program that prompts the user to enter a person’s date of birthin numeric form such as 8-27-1980. The program then outputs thedate of birth in the form: August 27, 1980. Your program must containat least two exception classes: invalidDay and invalidMonth.If the user enters an invalid value for day, then the program shouldthrow and catch an invalidDay object. Follow similar conventions forthe invalid values of month and year. (Note that your program musthandle a leap year.)
Write a program that prompts the user to enter a person's date of birth in numeric form such as 8-27-1980. The program then outputs the date of birth in the form: August 27, 1980. Your program must contain at least two exception classes: invalidDay and invalidMonth. If the user enters an invalid value for day, then the program should throw and catch an invalidDay object. Similar conventions for the invalid values of month and year. (Note that your program must handle a leap year.)
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 9.1 - Prob. 1STQCh. 9.1 - What output would the code in the previous...Ch. 9.1 - Prob. 3STQCh. 9.1 - Prob. 4STQCh. 9.1 - Prob. 5STQCh. 9.1 - Prob. 6STQCh. 9.1 - Prob. 7STQCh. 9.1 - Prob. 8STQCh. 9.1 - In the code given in Self-Test Question 1,...Ch. 9.1 - In the code given in Self-Test Question 1,...
Ch. 9.1 - Prob. 11STQCh. 9.1 - Prob. 12STQCh. 9.1 - Prob. 13STQCh. 9.1 - Prob. 14STQCh. 9.2 - Prob. 15STQCh. 9.2 - Prob. 16STQCh. 9.2 - Prob. 17STQCh. 9.2 - Prob. 18STQCh. 9.2 - Prob. 19STQCh. 9.2 - Prob. 20STQCh. 9.2 - Suppose that, in Self-Test Question 19, we change...Ch. 9.2 - Prob. 22STQCh. 9.2 - Prob. 23STQCh. 9.3 - Prob. 24STQCh. 9.3 - Prob. 25STQCh. 9.3 - Prob. 26STQCh. 9.3 - Prob. 27STQCh. 9.3 - Prob. 28STQCh. 9.3 - Repeat Self-Test Question 27, but change the value...Ch. 9.3 - Prob. 30STQCh. 9.3 - Prob. 31STQCh. 9.3 - Prob. 32STQCh. 9.3 - Consider the following program: a. What output...Ch. 9.3 - Write an accessor method called getPrecision that...Ch. 9.3 - Prob. 35STQCh. 9.4 - Prob. 36STQCh. 9.4 - Prob. 37STQCh. 9.4 - Prob. 38STQCh. 9 - Write a program that allows students to schedule...Ch. 9 - Prob. 2ECh. 9 - Prob. 3ECh. 9 - Prob. 4ECh. 9 - Prob. 5ECh. 9 - Write code that reads a string from the keyboard...Ch. 9 - Create a class Rational that represents a rational...Ch. 9 - Prob. 9ECh. 9 - Suppose that you are going to create an object...Ch. 9 - Revise the class RoomCounter described in the...Ch. 9 - Prob. 12ECh. 9 - Write a class LapTimer that can be used to time...Ch. 9 - Prob. 1PCh. 9 - Prob. 2PCh. 9 - Prob. 3PCh. 9 - Write a program that uses the class calculator in...Ch. 9 - Prob. 3PPCh. 9 - Prob. 7PPCh. 9 - Suppose that you are in change of customer service...Ch. 9 - Write an application that implements a trip-time...
Knowledge Booster
Similar questions
- Write a program that converts a time from 24-hour notation to 12-hour notation. To make the solution easier, a requirement is imposed on the input: It must be in xx:xx format, i.e. it must have two digits, a colon, and then another two digits. Define an exception class called TimeException. If the user enters an illegal time, like 10:65, or even gibberish, like 8&*68, your program should throw and handle a TimeException. Test your program with the file "times.txt" as input and store the result in the file "result.txt" File times.txt: 00:00 12:00 12:01 11:59 23:59 24:00 10:65 3:23 1145 8&*68 File result.txt: # 24-hour 12-hour -------------------------------------------- 1 00:00 12:00 AM 2 12:00 12:00 PM 3 12:01 12:01 PM 4 11:59 11:59 AM 5 23:59 11:59 PM 6 24:00 Time Exception 7 10:65…arrow_forwardI need to know how to do this in python: Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.arrow_forwardin c # i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. Next, create a Book class that contains fields for title, author, price, and number of pages. Include properties for each field. Throw a BookException if a client program tries to construct a Book object for which the price is more than 10 cents per page. Finally, using the Book class, create an array of five Books. Prompt the user for values for each Book. To handle any exceptions that are thrown because of improper or invalid data entered by the user, set the Book’s price to the maximum of 10 cents per page. At the end of the program, display all the entered, and…arrow_forward
- Write a Month class that holds information about the month. Write exception classes for the following error conditions:• A number less than 1 or greater than 12 is given for the month number.• An invalid string is given for the name of the month.Modify the Month class so that it throws the appropriate exception when either of these errors occurs. Demonstrate the classes in a program.arrow_forwardWrite a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.arrow_forwardWrite a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info. **This is the output i am having trouble with. on line 20 The print string shows the print(ve, 'could not calculate heart rate info.') And i know that the extra space i see at the begining of that second line of output is due to the comma but I cannot figure out how to make the same line print but with out the space from the comma after…arrow_forward
- Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info. **This is the output i am having trouble with. on line 20 The print string shows the print(ve, 'could not calculate heart rate info.') And i know that the extra space i see at the begining of that second line of output is due to the comma but I cannot figure out how to make the same line print but with out the space from the comma after…arrow_forwardin c # i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. my errors are Test Case IncompleteComplete program walkthrough, test 1 InputBook1Author110.99200Book2Author219.99100Book3Author225.00600Book4Author35.0020Book5Author48.00120OutputEnter information for book #1:Title: Author: Price: Number of pages: Unhandled Exception:System.DivideByZeroException: Value was either too large or too small for a Decimal. at System.Decimal+DecCalc.VarDecDiv (System.Decimal& d1, System.Decimal& d2) [0x0003e] in <7b90a8780ac4414295b539b19eea7eea>:0 at System.Decimal.Divide (System.Decimal d1, System.Decimal d2) [0x00000] in…arrow_forwardin C# i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. my errors are Test Case IncompleteComplete program walkthrough, test 1 InputBook1Author110.99200Book2Author219.99100Book3Author225.00600Book4Author35.0020Book5Author48.00120OutputBook1 by Author1 Price $10.99 200 pages.Book2 by Author2 Price $19.99 100 pages.Book3 by Author2 Price $25.00 600 pages.Book4 by Author3 Price $5.00 20 pages.Book5 by Author4 Price $8.00 120 pages. ResultsFor Book2, ratio is invalid....Price is $19.99 for 100 pages.For Book4, ratio is invalid....Price is $5.00 for 20 pages.Book1 by Author1 Price $10.99 200 pages.Book2 by Author2 Price $10.00 100…arrow_forward
- python Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info. # here is the script I have but its not working def get_age():age = int(input())age=int(input("Enter the age:"))if age<18 or age>75:raise ValueError('Invalid age.')return age # TODO: Complete fat_burning_heart_rate() functiondef fat_burning_heart_rate(age):heart_rate=(220-age)*.70return heart_rate if __name__ == "__main__":#…arrow_forwardWrite a simple program using exception handling. The program allows the payment by credit card if the amount is more than RM50. Otherwise, payment can only be done by casharrow_forwardTake two integers from the user, Write a program in Java to divide a number by another. Point to be NOTED: User may give "string or o“ as divider intentionally or by mistake. You must handle all types of exception, But the program should not stop if there is any kind of error or exception. Your program should provide as many chances as user need to complete the calculation. The program will immediately exit after successfully dividing the 1st number by 2nd number.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
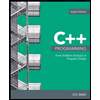
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
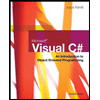
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,