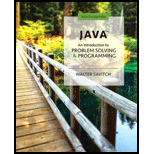
Explanation of Solution
Exception classes:
The two classes that derives “InvalidFormattingException” are “InvalidHourException” and “InvalidMinuteException”.
InvalidHourException.java:
//Create a class that extends Exception
public class InvalidHourException extends InvalidFormattingException
{
//Define a parameterized constructor
public InvalidHourException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidHourException” extends “InvalidFormattingException” and this exception occurs when the hour entered by the user in invalid.
InvalidMinuteException.java:
//Create a class that extends Exception
public class InvalidMinuteException extends InvalidFormattingException
{
//Define a parameterized constructor
public InvalidMinuteException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidMinuteException” extends “InvalidFormattingException” and this exception occurs when the minute entered by the user in invalid.
Complete program:
Main.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class Main
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public TimeOfDay()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException, InvalidHourException, InvalidMinuteException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Check the condition
if(hourFound<1 || hourFound>12)
//Throw the exception with a message
throw new InvalidHourException("Hour not in the range of 1 to 12");
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check the condition
if(minuteFound<0 || minuteFound>59)
//Throw the exception with a message
throw new InvalidMinuteException("Minute not in the range of 0 to 59");
//Check condition
if(!amString.equals("am") && !amString.equals("pm"))
//Throw the exception with a message
throw new InvalidFormattingException("Did not have am/pm");
//Assign the value
hour = hourFound;
//Assign the value
minute = minuteFound;
//Check if the string is am
if(amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Modify the MilTime class given under Final exam module. The class should implement the following exceptions: BadHour Throw when an invalid hour (< 0 or > 2359) is passed to the class. BadSeconds Throw when an invalid number of seconds (<0 or > 59) is passed to the class. Demonstrate the class in a driver program. Demo in the main function. the file: // MillTIme.cpp//#include "stdafx.h"#include <iostream>using namespace std;class Time{protected: int hour; int min; int sec;public: Time(int h, int m, int s) { hour = h; min = m; sec = s; } int getHour() { return hour; } int getMin() { return min; } int getSec() { return sec; }}; class MilTime : public Time{private: int milHours; int milSeconds;public: MilTime(int h = 0, int s = 0) : Time(0, 0, s) { if (h < 0 || h > 2359) { cout << "Hours must be in the range 0 - 2359.\n"; milHours = h;…arrow_forwardWrite a program that prompts the user to enter a person's date of birth in numeric form such as 8-27-1980. The program then outputs the date of birth in the form: August 27, 1980. Your program must contain at least two exception classes: invalidDay and invalidMonth. If the user enters an invalid value for day, then the program should throw and catch an invalidDay object. Similar conventions for the invalid values of month and year. (Note that your program must handle a leap year.)arrow_forwardJAVA PROGRAM Probloem: Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. First Example:Input:The quick brown fox jumped over the lazy dogs.Output:The quick brown fox jumped over the lazy dogs. Second Example:Input: The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown…arrow_forward
- in C# i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. For example, an error message: For Goodnight Moon, ratio is invalid. ...Price is $12.99 for 25 pages. Next, create a Book class that contains fields for title, author, price, and number of pages. Include properties for each field. Throw a BookException if a client program tries to construct a Book object for which the price is more than 10 cents per page. Finally, using the Book class, create an array of five Books. Prompt the user for values for each Book. To handle any exceptions that are thrown because of improper or invalid data entered by the user, set the Book’s…arrow_forwardComplete the following tasks: Write an exception handler to catch ValueError and output 'float(): Input is not a float.' Write an exception handler to catch OverflowError and output 'math.pow(): result of 5 raised to the power of ', followed by x_value and ' is too large.'arrow_forwarda) Write a method in java that searches a numeric array for a specified value. The method should return the subscript of the element containing the value if it is found in the array. If the value is not found , the method should throw an exceptionof the Exception class with the error message “Element not found”. b) Write an exception class that can be thrown when a negative number is passed to a method.arrow_forward
- Exception - Java Write a program that will compute the factorial of a number. Your program must catch an exception if the input is not a number. For example: Input Result 5 120 w InputMismatchException -5 Invalid Inputarrow_forwardJava Foundations: Please provide a basic exception program. Do not use input, output. Write the Java code for the following: Write the InvalidGradeException class that will be used below Write the Java program that reads an integer grade from a user and checks that it is valid (between 0 and 100). If it is invalid an InvalidGradeException will be thrown, stating what the invalid grade is. This will be caught in the main( ) method which will write a message about the invalid grade. If the grade is valid, it is written to the monitor.arrow_forwardJava Exception method withdraw throws an exception if amount is greater than balance. For example: Test Result Account account = new Account("Acct-001","Juan dela Cruz", 5000.0); account.withdraw(5500.0); System.out.println("Balance: "+account.getBalance()); Insufficient: Insufficient funds. Balance: 5000.0 Account account = new Account("Acct-001","Juan dela Cruz", 5000.0); account.withdraw(500.0); System.out.println("Balance: "+account.getBalance()); Balance: 4500.0arrow_forward
- JAVA EXCEPTION HANDLING:Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. For example: Input Result The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped…arrow_forwardPlease do this in Java! 3. RetailItem Exceptions Programming Challenge 4 of Chapter 6 required you to write a RetailItem class that holds data pertaining to a retail item. Write an exception class that can be instantiated and thrown when a negative number is given for the price. Write another exception class that can be instantiated and thrown when a negative number is given for the units on hand. Demonstrate the exception classes in a program. /** *Description: This program will displays a string without any user interaction *Class: Fall - COSC 1437.81002 *Assignment1: Hello World *Date: 08/15/2011 *@author Zoltan Szabo *@version 0.0.0 */ For each method, you will also be required to create docstring as follows: /** * @param String as args * @return Termination code as int, 0 for normal, anything else is error condition * @throws Nothing is implemented */ Flowcharts/UML and Pseudo code All assignment questions must show design flowchart/UML and/or pseudo code unless otherwise…arrow_forwardPLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
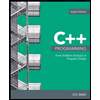
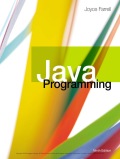
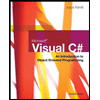