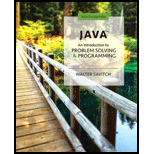
Concept explainers
Explanation of Solution
Modified code:
“Account.java”:
//Class Account
public class Account
{
//Private variable
private double balance;
//Define a constructor
public Account()
{
//Initialize the amount value
balance = 0;
}
//Parameterized constructor
public Account(double initialDeposit)
{
//Assign the value
balance = initialDeposit;
}
//Function to get balance
public double getBalance()
{
//Return the amount
return balance;
}
//Function to deposit the amount
public double deposit(double amount) throws NegativeAmountException
{
//Check if amount is greater than 0
if (amount > 0)
//Add the value to the balance
balance += amount;
//Else
Else
//return -1;
//Throw an exception
throw new NegativeAmountException();
//Return the balance
return balance;
}
//Function definition to withdraw the amount
public double withdraw(double amount) throws InsufficentBalanceException, NegativeAmountException
{
//Check if amount is greater than balance
if (amount > balance)
//throw an exception
throw new InsufficentBalanceException();
//Check if amount is less than 0
else if (amount < 0)
//Throw an exception
throw new NegativeAmountException();
//Else
else
//Calculate the balance
balance -= amount;
//Return the balance amount
return balance;
}
//Main method
public static void main(String[] args)
{
//Create an object for the class
Account a = new Account(10);
//Try block
try
{
//Deposit the value
a...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- JAVA HELP please Method Overloading is a feature that allows a class to have more than one method having the same name, if their argument lists are different. Design a simple class and define a maximum method that finds the maximum of two integer values? Design an application to test that your new method is working properly. Now overload the maximum method to accept three double values and return the maximum of these three values? Finally overload the maximum method again such that it accept four double or four integer values and return the average of these four values? Modify your main( ) application to call and test these new methods. In each case the maximum overloaded methods should return the correct maximum values. Attach you java source codes and a screenshot of the output(s).arrow_forwardIf two methods in the same class have the same name, which is true? (choose one) They must have a different number of parameters They must have different return types They must have different parameter type lists The compiler must generate an error messagearrow_forwardPROGRAMMING LANGUAGE: C++ ALSO PUT SCREENSHOTS WITH EVERY TASK. TASK 1 : A class of ten students took a quiz .The grades (integers are in range 0-100) for this quiz are available to you .Determine class average on quiz . TASK 2: Write c++ code that print summery of exam result and decide either student should have makeup class or not .If more then 30% of class fails in exam it’s mean they need a makeup class otherwise they don’t need any makeup class. For class strength take input from user (Hint: take two variables pass and fail) TASK 3: write a c++ that will determine whether a department-store customer has exceeded the credit limit on the charge account .For each customer , following facts are available : Account number (an integer) Balance at beginning of month Total of all items charged by this customer this month Total of all credit applied to this customer’s account this month. Allowed credit limit You are required to use a while structure to input each of these facts ,…arrow_forward
- Java Overview In this task, you should try using the Scanner class to read data from the keyboard. This data should be stored in variables in your program and then printed on screen. Task You should write a class called Story that should include a main method. This programs should create a fairy tale for a child. The program is based on a completed story, but some words must be replaced with such as the user may enter. The fairy tale you are going to based on are the following: The little ant (1) There was once a small, small ant that lived with its (2) and its (3) siblings in an anthill, by a large (4) in the forest. The little ant is called (1). For the most part, (1) and his siblings used to work on carrying (5) and (6) to the stack, but today it was (7), so (1) was free. The words that are crossed out are the words that your program should ask the user for. In total, there are seven entries the user must make. The program must save these entries in separate variables and then…arrow_forwardtrue of false: a) The first step in any programming task is to start coding. b) The only places we would ever want to put a comment in our code are above a method and above a class.arrow_forwardThe following class maintains an account balance and returns aspecial error code.public class Account{private double balance;// returns new balance or -1 if errorpublic double deposit(double amount){if (amount > 0)balance += amount;elsereturn -1; // Code indicating errorreturn balance;}}Rewrite the class so that it throws appropriate exception instead ofreturning -1 as an error code. Write test code that attempts to depositinvalid amounts and catches the exceptions that are thrownarrow_forward
- Overloading a method and overriding a method are two separate but related concepts.arrow_forwardpublic static class Lab2{public static void Main(){int idNum;double payRate, hours, grossPay;string firstName, lastName;// prompt the user to enter employee's first nameConsole.Write("Enter employee's first name => ");firstName = Console.ReadLine();// prompt the user to enter employee's last nameConsole.Write("Enter employee's last name => ");lastName = Console.ReadLine()// prompt the user to enter a six digit employee numberConsole.Write("Enter a six digit employee's ID => ");idNum = Convert.ToInt32(Console.ReadLine());// prompt the user to enter the number of hours employeeworkedConsole.Write("Enter the number of hours employee worked =>");// prompt the user to enter the employee's hourly pay rateconsole.Write("Enter employee's hourly pay rate: ");payRate = Convert.ToDouble(Console.ReadLine());// calculate gross paygrossPay = hours * payRate;// output resultsConsole.WriteLine("Employee {0} {1}, (ID: {2}) earned {3}",firstName, lastName, idNum,…arrow_forwardMethod overloading is a word that refers to the practise of using an excessive number of methods.arrow_forward
- True or False A static method can reference an instance variable.arrow_forwardMicrosoft Visual C#, 7th edition. chapter 11, Programming exercise 11-3 Instructions: Write the program FindSquareRoot that finds the square root of a user’s input value. The Math class contains a static method named Sqrt() that accepts a double and returns the parameter’s square root. If the user’s entry cannot be converted to a double, display an appropriate message, and set the square root value to 0. Otherwise, test the input number’s value. If it is negative, throw a new ApplicationException to which you pass the message “Number can’t be negative.” and again set sqrt to 0. If the input value is a double and not negative, pass it to the Math.Sqrt() method, and display the returned value. An example of the program is shown below: Enter a number 9 Square root is 3 or Enter a number -9 Error: Number can't be negative. Square root is 0arrow_forwardPython Class and Objects Create a class named "Account" and “Bank” The class “Account” should have a parameter of id - integer type name - string type balance - float type # (Optional) You may add more parameters for your convenience 3. Create a method for “Account” class: checkBalance(self) - this will show the Account's remaining balance or money withdraw(self, amount) - this will withdraw an amount from the Account deposit(self, amount) - this will deposit an amount from the Account # (Optional) You may add more methods like sendMoney() etc. 4. Create a method for “Bank” class: addAccount(self, account) – used to register an account to the bank # (Optional) You may add more methods for your convenience 4. Create 3 Account objects with the following attributes in the main method account1 - id=(any number), name=(Any Name You Want), balance=7000 (strictly use this value) account2 - id=(any number), name=(Any Name You Want), balance= (any amount) account3 -…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
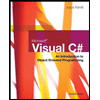
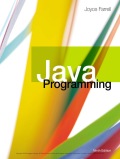
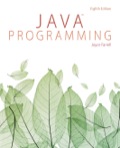