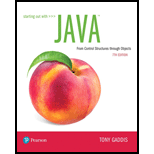
Concept explainers
Geometry Calculator
Design a Geometry class with the following methods:
- A static method that accepts the radius of a circle and returns the area of the circle. Use the following formula:
Area = πr2
Use Math.PI for π and the radius of the circle for r.
- A static method that accepts the length and width of a rectangle and returns the area of the rectangle. Use the following formula:
Area = Length × Width
- A static method that accepts the length of a triangle’s base and the triangle’s height. The method should return the area of the triangle. Use the following formula:
Area = Base × Height × 0.5
The methods should display an error message if negative values are used for the circle’s radius, the rectangle’s length or width, or the triangle’s base or height.
Next, write a program to test the class, which displays the following menu and responds to the user’s selection:
Geometry Calculator
- 1. Calculate the Area of a Circle
- 2. Calculate the Area of a Rectangle
- 3. Calculate the Area of a Triangle
- 4. Quit
Enter your choice (1-4):
Display an error message if the user enters a number outside the range of 1 through 4 when selecting an item from the menu.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Starting Out With Visual Basic (8th Edition)
Problem Solving with C++ (10th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
C++ How to Program (10th Edition)
C Programming Language
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- C# LANGUAGE Create a method named getCircleDiameter that takes a radius of double type as the parameter. The method should return the diameter of a circle. To compute the diameter of a circle, multiply the radius by 2.arrow_forwardPython Class and Objects Create a class named "Account" and “Bank” The class “Account” should have a parameter of id - integer type name - string type balance - float type # (Optional) You may add more parameters for your convenience 3. Create a method for “Account” class: checkBalance(self) - this will show the Account's remaining balance or money withdraw(self, amount) - this will withdraw an amount from the Account deposit(self, amount) - this will deposit an amount from the Account # (Optional) You may add more methods like sendMoney() etc. 4. Create a method for “Bank” class: addAccount(self, account) – used to register an account to the bank # (Optional) You may add more methods for your convenience 4. Create 3 Account objects with the following attributes in the main method account1 - id=(any number), name=(Any Name You Want), balance=7000 (strictly use this value) account2 - id=(any number), name=(Any Name You Want), balance= (any amount) account3 -…arrow_forwardTrue or False: When passing multiple arguments to a method, the order in which the arguments are passed is not important.arrow_forward
- Java Programming Travel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. This process is illustrated in the following example: Step 1. Enter the ticket number; for example, 123454. Step 2. Remove the last digit, leaving 12345. Step 3. Determine the remainder when the ticket number is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4. Assign the Boolean value of the comparison between the remainder and the digit dropped from the ticket number. Step 5. Display the result—true or false—in a message box. Accept the…arrow_forwardpython Cylinder class: Instance variables: Make instance variables appropriately to accomplish the tasks you need. Methods: ?_init_ :: constructor, initializes instance variables o Additional Parameters: diameter and height (in that order) o Assumption: diameter and height will always be numbers which represent measurements in millimeters o Temporary Assumption (we will fix this later): diameter will never be negative and height wilI always be positive number above o ? get_volume : returns the volume of the cylinder based on its height o Additional Parameters: No additional parameters (just self). .0 Note: Use pi from the math module ?_str__ :: returns string representation with this format: "Cylinder (radius: 20.25mm, thickness: 6.10mm, volume: 7858.32mm^3)" o Additional Parameters: No additional parameters. o Notes: ? The quotes in the example are just our string boundaries! The first character in the above example is C. ? This method reports the radius (NOT the diameter). ? All…arrow_forwardCode the following: Language : Java Create a method called triangle that will take in three angles and tell you if you have a triangle Create an overloaded version of the triangle method that only takes in one angle and tells you if it could be a triangle create an overloaded version of the triangle method that only takes in two angles. You must check if it's possible for it to be an isosceles triangle. Don't forget to check both angles : example: angle1*2 + angle2 == 180 or angle1 +angle2*2 == 180arrow_forward
- Instructions: IMPORTANT: This is a continuation of the previous part of the project and assumes that you are starting with code that fulfills all requirements from that part of the project. Modify the your code from the previous part of the project to make it modular. In addition to the main method, your code must include the following static methods: Method 1 - displayTitle A method that creates a String object in memory to hold the text “Computer Hardware Graphics Quality Recommendation Tool” and displays it Method 2 – getResolutionString A method that accepts an integer value (1, 2, 3, or 4) that denotes the monitor resolution. The method should return the appropriate String representation of the monitor resolution. For example, if the method is passed an integer value of 1, it should return a String with a value of “1280 x 720”. (See Step 4 of Project 1) Method 3 – getMultiplierValue A method that accepts an integer value (1, 2, 3, or 4) that denotes the monitor resolution and…arrow_forwardAssignment: Dice Rolling Program Objective: Create a Java program that rolls two dice and displays the results. The program should have two Java classes: one for a single die and another for a pair of dice. Assignment Details: User Input: Ask the user to specify the number of sides they want on each die. Ensure that the user's input is within a reasonable range. Dice Rolling: Simulate rolling the dice using Math.random() based on the user's chosen number of sides. Display the sum of the values rolled, e.g., "5 + 3 = 8." Special Combinations: If the dice roll results in combinations of 2, 7, or 12, print special messages: "1 + 1 = 2 snake eyes!" "3 + 4 = 7 craps!" "6 + 6 = 12 box cars!" Main Method: In the main method, create a pair of dice, roll them, and display the results. Allow the user to decide whether to continue rolling the dice or exit the program. Additional Features: You are welcome to add more features or enhancements to the program if desired. In…arrow_forwardIn PYTHON Design a class named Car that has the following fields: yearModel: The yearModel field is an integer that holds the car's year model (e.g, 1959). speed: The speed field is an integer that holds the car's current speed. In addition, the class should have the following constructor and other methods: Constructor: The constructor should accept the car's year model and speed as arguments. These values should be assigned to the object's yearModel and speed fields.The constructor should also assign 0 to the speed field. Accessors: Design appropriate accessor methods to get the values stored in an object's yearModel and speed fields. accelerate: The accelerate method should add 5 to the speed field each time is called. brake: The brake method should subtract 5 from the speed field each time it is called. Next, design a program that creates a Car object, and then calls the accelerate method five times. After each call to the accelerate method, get the current speed ofthe car and…arrow_forward
- Retail StoreSuppose you have been consulted by a retail shop around your area to build a simple calculator. The calculator must be able to perform arithmetic operations (+, -, *, /) on integers. The program receives an expression from the user. The expression is structured as follows: integer input followed by an operator and another integer input from user prior to displaying the final result. Write a Java application that can perform the above expression. Create a method that takes two parameters and performs an arithmetic operation on the passed values. Your final output should consist of the expression and the result.arrow_forwardMath: pentagonal numbers) A pentagonal number is defined as n(3n-1)/2 , for n = 1,2, . . ., and so on. Therefore, the first few numbers are 1, 5 ,12, 22, ... Write a Java program with an object class called Pentagon and a test class called testPentagon. The Pentagon object class must contain a method with the following header that calculates and return a pentagonal number: public int calcPentagonalNumber(int n) Use the formula: n(3n-1)/2 In the test class: Make use of the calcPentagonalNumber(n) method in the Pentagon object class and display the first 100 pentagonal numbers with 10 numbers on each line. NOTE: NO marks if the pentagonal number is calculated in the test class. Your solution must be an object-oriented solutionarrow_forwardsolve eith java :- Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
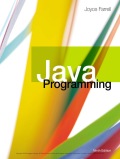
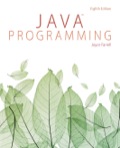