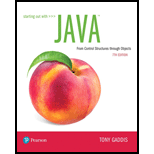
Car Instrument Simulator
For this assignment, you will design a set of classes that work together to simulate a car’s fuel gauge and odometer. The classes you will design are the following:
- The Fuel Gauge Class: This class will simulate a fuel gauge. Its responsibilities are as follows:
- To know the car’s current amount of fuel, in gallons.
- To report the car’s current amount of fuel, in gallons.
- To be able to increment the amount of fuel by 1 gallon. This simulates putting fuel in the car. (The car can hold a maximum of 15 gallons.)
- To be able to decrement the amount of fuel by 1 gallon, if the amount of fuel is greater than 0 gallons. This simulates burning fuel as the car runs.
- The Odometer Class: This class will simulate the car’s odometer. Its responsibilities are as follows:
- To know the car’s current mileage.
- To report the car’s current mileage.
- To be able to increment the current mileage by 1 mile. The maximum mileage the odometer can store is 999,999 miles. When this amount is exceeded, the odometer resets the current mileage to 0.
- To be able to work with a FuelGauge object. It should decrease the FuelGauge object’s current amount of fuel by 1 gallon for every 24 miles traveled. (The car’s fuel economy is 24 miles per gallon.)
Demonstrate the classes by creating instances of each. Simulate filling the car up with fuel, and then run a loop that increments the odometer until the car runs out of fuel. During each loop iteration, print the car’s current mileage and amount of fuel.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Problem Solving with C++ (10th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
C++ How to Program (10th Edition)
Java How To Program (Early Objects)
- The Spider Game Introduction: In this assignment you will be implementing a game that simulates a spider hunting for food using python. The game is played on a varying size grid board. The player controls a spider. The spider, being a fast creature, moves in the pattern that emulates a knight from the game of chess. There is also an ant that slowly moves across the board, taking steps of one square in one of the eight directions. The spider's goal is to eat the ant by entering the square it currently occupies, at which point another ant begins moving across the board from a random starting location. Game Definition: The above Figure illustrates the game. The yellow box shows the location of the spider. The green box is the current location of the ant. The blue boxes are the possible moves the spider could make. The red arrow shows the direction that the ant is moving - which, in this case, is the horizontal X-direction. When the ant is eaten, a new ant is randomly placed on one of the…arrow_forwardExercises: PolymorphismProblem 1. VehiclesWrite a program that models 2 vehicles (Car and Truck) and will be able to simulate driving and refueling them inthe summer. Car and truck both have fuel quantity, fuel consumption in liters per km and can be driven givendistance and refueled with given liters. But in the summer both vehicles use air conditioner and their fuelconsumption per km is increased by 0.9 liters for the car and with 1.6 liters for the truck. Also the truck has a tinyhole in his tank and when it gets refueled it gets only 95% of given fuel. The car has no problems when refueling andadds all given fuel to its tank. If vehicle cannot travel given distance its fuel does not change.Input On the first line - information about the car in format {Car {fuel quantity} {liters per km}} On the second line – info about the truck in format {Truck {fuel quantity} {liters per km}} On third line - number of commands N that will be given on the next N lines On the next N lines –…arrow_forwardjava programming language You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is tested – create…arrow_forward
- Parking Ticket SimulatorFor this assignment you will design a set of classes that work together to simulate apolice officer issuing a parking ticket. The classes you should design are:• The Parkedcar Class: This class should simulate a parked car. The class's respon-sibilities are:To know the car's make, model, color, license number, and the number of min-utes that the car has been parkedThe BarkingMeter Class: This class should simulate a parking meter. The class'sonly responsibility is:- To know the number of minutes of parking time that has been purchased• The ParkingTicket Class: This class should simulate a parking ticket. The class'sresponsibilities areTo report the make, model, color, and license number of the illegally parked carTo report the amount of the fine, which is $2S for the first hour or part of anhour that the car is illegally parked, plus $10 for every additional hour or part ofan hour that the car is illegally parkedTo report the name and badge number of the police…arrow_forwardExercise 6 - Loaded Coin Simulation Make a new class in the Lab2 project called LoadedCoinSim that simulates the toss of a loaded coin. A coin is loaded when it is constructed such that it is more likely to land on one value than another. Assume the probability the coin will land head is HEAD_PROB, and declare that as a constant in your program. Initially, use a value of 0.75, which means 75% chance the coin will show heads. Given this probability, simulate ten coin tosses and displaying the result. At the end, display the number of times the coin showed heads. The output should appear like this: Toss 1: HEADS Toss 2: HEADS Toss 3: HEADS Toss 4: TAILS Toss 5: HEADS Toss 6: HEADS Toss 7: HEADS Toss 8: HEADS Toss 9: TAILS Toss 10: HEADS Number of heads = 8arrow_forwardDescription: The game is a single player scenario, in which the player’s army needs to defeat the enemy’s (AI’s) army. There are 4 possible troops for an army: Archers, Spearman, Cavaliers, Footman. Each troop has some attributes and some actions. And to avoid excessive programming and calculation, we want to treat these troops as squadrons. The player always starts with 10 squadrons of their choices: they can choose any combinations of the 4 possible troop types. Each squadron should have 100 members of that troop type. Each turn, the player is allowed to choose one of their squadrons and perform an action which is allowed by that troop type. Player and the AI take turns to make actions. The game continues until either the player or the AI has no troops left. Troop Types and Descriptions: Archers: should be able to attack from range with no casualties, meaning the attack action should not cause any damage to themselves. They should be pretty fragile to anything themselves. They…arrow_forward
- An artist's discography includes several albums. Each album includes several songs. We want to model the Discography, Album and Song classes as follows • a discography has a single artist attribute and a total_time method that returns the total minutes corresponding to the artist's entire discography • an album has a single name attribute and a total_time method that returns the total minutes for the songs on the album a song has two attributes: title and duration and a duration method that returns the duration in minutes of the song • we want to take advantage of the composite pattern so that the duration method delivers the total number of minutes of a song, album or the entire discography of the artist depending on the object in question a) Draw a UML class diagram showing the solution b) Write the Ruby code that implements itarrow_forwardAssignment 10: Chapter 10 Complete Programming Challenge 10 at the end of chapter 10 10. Ship, CruiseShip, and CargoShip Classes Design a Ship class that the following members: A field for the name of the ship (a string) - A field for the year that the ship was built (a string). A constructor and appropriate accessors and mutators. A toString method that displays the ship's name and the year it was built. Design a CruiseShip class that extends the Ship class. The CruiseShip class should have the following members: A field for the maximum number of passengers (an int). A constructor and appropriate accessors and mutators. A toString method that overrides the toString method in the base class. The CruiseShip class's toString method should display only the ship's name and the maximum number of passengers. Design a CargoShip class that extends the Ship class. The CargoShip class should have the following members: A field for the cargo capacity in tonnage (an int). A constructor and…arrow_forwardCode the StudentAccount class according to the class diagramMethods explanation:pay(double): doubleThis method is called when a student pays an amount towards outstanding fees. The balance isreduced by the amount received. The updated balance is returned by the method.addFees(double): doubleThis method is called to increase the balance by the amount received as a parameter. The updatedbalance is returned by the method.refund(): voidThis method is called when any monies owned to the student is paid out. This method can onlyrefund a student if the balance is negative. It displays the amount to be refunded and set thebalance to zero. (Use Math.abs(double) in your output message). If there is no refund, display anappropriate message.Test your StudentAccount class by creating objects and calling the methods addFees(), pay() andrefund(). Use toString() to display the object’s data after each method calledarrow_forward
- Java Assignment Outcomes: Student will demonstrate the ability to utilize inheritance in a Java program. Student will demonstrate the ability to apply the IS A and HAS A relationships. Program Specifications: Start by watching Video Segment 16 from Dr. Colin Archibald's video series (found in the module overview). Key in the program shown in the video and make sure it works. Then, add the following: Animals have a Weight. Animals have a Height. Dog is an Animal. Dogs have a Name. Dogs have a Breed. Dogs have a DOB. Cat is an Animal Cats have a Name. Cats have 9 lives, so you need to keep track of the remaining lives once a cat dies. Bird is an Animal Birds have a wing span Birds have a canFly which is true or false (some birds cannot fly) Create a test class that creates one of each type of animal and displays the animal’s toString method. Submission Requirements: You must follow the rules from the prior assignments. UMLs and Design Tools are not required for this one. YOU MAY…arrow_forwardRequirements A vehicle has a certain fuel efficiency (measured in miles/gallon) and a certain amount of fuel in the gas tank. We need to simulate driving the vehicle for a given distance while reducing the amount of gasoline in the fuel tank. We also need to be able to check how much gas is in the tank, and we need to be able to add gasoline to the fuel tank. Build the Vehicle class with the following specifications: Instance data:Variable mpg for fuel efficiency (miles per gallon = mpg)Variable gas to save how many gallons of gas left in the tank Constructors:Default constructor with no parameter. Use 0 as initial values.Overloaded constructor with two parameters Methods:getMPG() & setMPG()(getGas() & setGas()toString() methoddrive() to simulate that the car is driven for certain miles. For example, v1.drive(100) means vehicle v1 is driven 100 miles. You need to calculate the gas cost and update the gas tank: gas = gas - miles/mpg. You also need to check if there…arrow_forwardsolve with java Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
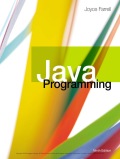