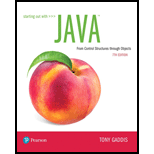
Heads or TaiLs Game
This game is meant for two or more players. In this game, the players take turns flipping a coin. Before the coin is flipped, players should guess if the coin will land face up or face down. If a player guesses correctly, then that player is awarded a point. If a player guesses incorrectly, then that player will lose a point. The first player to score five points is the winner.
Write a program that simulates the game being played by two players. Use the Coin class that you wrote as an assignment in Chapter 6 (

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Degarmo's Materials And Processes In Manufacturing
Java: An Introduction to Problem Solving and Programming (8th Edition)
Modern Database Management
Web Development and Design Foundations with HTML5 (8th Edition)
Database Concepts (8th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Refer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward
- 6.3A-3. Multiple Access protocols (3). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access wireless nodes at times t=0.1, 1.4, 1.8, 3.2, 3.3, 4.1. Each transmission requires exactly one time unit. 1 t=0.0 2 3 45 t=1.0 t-2.0 t-3.0 6 t=4.0 t-5.0 For the CSMA protocol (without collision detection), indicate which packets are successfully transmitted. You should assume that it takes .2 time units for a signal to propagate from one node to each of the other nodes. You can assume that if a packet experiences a collision or senses the channel busy, then that node will not attempt a retransmission of that packet until sometime after t=5. Hint: consider propagation times carefully here. (Note: You can find more examples of problems similar to this here B.] ☐ U ப 5 - 3 1 4 6 2arrow_forwardJust wanted to know, if you had a scene graph, how do you get multiple components from a specific scene node within a scene graph? Like if I wanted to get a component from wheel from the scene graph, does that require traversing still? Like if a physics component requires a transform component and these two component are part of the same scene node. How does the physics component knows how to get the scene object's transform it is attached to, this being in a scene graph?arrow_forwardHow to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward
- 9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward
- 5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward15. The below figure (sequence of moves) shows several stages of the process for a simple initial configuration. 90 a a 90 b a 90 91 b b b b Represent the action of the Turing machine (a) move from one configuration to another, and also (b) represent in the form of arbitrary number of moves.arrow_forward12. Eliminate useless productions from Sa aA BC, AaBλ, B→ Aa, C CCD, D→ ddd Cd. Also, eliminate all unit-productions from the grammar. 13. Construct an npda that accepts the language L = {a"b":n≥0,n‡m}.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
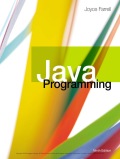
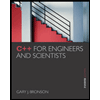
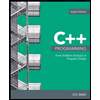