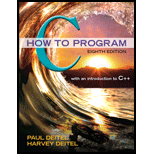
Concept explainers
(Character Testing) Write a

Program Plan:
- mainfunction used to test all the functions.
- Declare character type of variable character.
- printf function used to print the formatted output on console.
- scanf function used to takea formatted inputfrom console.
Program Description:
The following program will input character and test all the character-handling library functions.
Explanation of Solution
Program:
//header file included #include <stdio.h> #include <string.h> #include <stdlib.h> //included main function intmain() { //declare character type variable char character; //display message to user printf("Enter the character:\n"); //input character scanf("%c", &character); //testing each function of character-handling library //isdigit function printf("'%c'%sdigit\n",character, isdigit(character)?"is a ":"is not a "); //isalpha function printf("'%c'%salphabet\n",character, isalpha(character)?"is a ":"is not a "); //isalnum function printf("'%c'%salpha-numeric\n",character, isalnum(character)?"is a ":"is not a "); //isxdigit function printf("'%c'%shexadecimal digit\n",character, isxdigit(character)?"is a ":"is not a "); //islower function printf("'%c'%slowercase letter\n",character, islower(character)?"is a ":"is not a "); //isupper function printf("'%c'%suppercase letter\n",character, isupper(character)?"is a ":"is not a "); //toupper function printf("The uppercase letter of '%c' is '%c'\n",character, toupper(character)); //tolower function printf("The lowercase letter of '%c' is '%c'\n",character, tolower(character)); //isspace function printf("'%c'%sspace\n",character, isspace(character)?"is a ":"is not a "); //iscntrl function printf("'%c'%scontrol character\n",character, iscntrl(character)?"is a ":"is not a "); //ispunct function printf("'%c'%sprinting character of ispunct\n",character, ispunct(character)?"is a ":"is not a "); //isprint function printf("'%c'%sprinting character of isprint\n",character, isprint(character)?"is a ":"is not a "); //isgraph function printf("'%c'%sprinting character of isgraph\n",character, isgraph(character)?"is a ":"is not a "); //exiting from program return 0; }
Explanation:
In the above program, one character is inputted through scanf function. The %c is the format specifier used to input character type value. All the character-handling library function is used to test and print the output. The ternary operator is used inside the printf function to determine if the entered character is a digit, alphanumeric character, hexadecimal digit, lowercase letter or uppercase letter or not.
Sample Output:
Want to see more full solutions like this?
Chapter 8 Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (4th Edition)
Starting Out With Visual Basic (7th Edition)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Computer Science: An Overview (12th Edition)
- (Rounding Numbers) Function floor may be used to round a number to a specific decimalplace. The statementy = floor(x * 10 + .5) / 10;rounds x to the tenths position (the first position to the right of the decimal point). The statementy = floor(x * 100 + .5) / 100;rounds x to the hundredths position (the second position to the right of the decimal point). Writea program that defines four functions to round a number x in various waysa) roundToInteger(number)b) roundToTenths(number)c) roundToHundreths(number)d) roundToThousandths(number)For each value read, your program should print the original value, the number rounded to thenearest integer, the number rounded to the nearest tenth, the number rounded to the nearest hundredth, and the number rounded to the nearest thousandth.arrow_forward(Bar-Chart Printing Program) One interesting application of computers is drawing graphsand bar charts. Write a program that reads five numbers (each between 1 and 30). For each numberread, your program should print a line containing that number of adjacent asterisks. For example,if your program reads the number seven, it should print *******.arrow_forward(Variable-Length Argument List: Calculating Products) Write a program that calculates theproduct of a series of integers that are passed to function product using a variable-length argumentlist. Test your function with several calls, each with a different number of arguments.arrow_forward
- ( MindTap - Cenage )Example 5-6 implements the Number Guessing Game program. If the guessed number is not correct, the program outputs a message indicating whether the guess is low or high. Modify the program as follows: Suppose that the variables num and guess are as declared in Example 5-6 and diff is an int variable. Let diff = the absolute value of (num - guess). If diff is 0, then guess is correct and the program outputs a message indicating that the user guessed the correct number. Suppose diff is not 0. Then the program outputs the message as follows: If diff is greater than or equal to 50, the program outputs the message indicating that the guess is very high (if guess is greater than num) or very low (if guess is less than num). If diff is greater than or equal to 30 and less than 50, the program outputs the message indicating that the guess is high (if guess is greater than num) or low (if guess is less than num). If diff is greater than or equal to 15 and less than 30, the…arrow_forward(Converting Strings to Integers for Calculations ) Write a program that inputs six strings that represent integers , converts the ngs to integers , and calculates the sum and average of the six values .arrow_forward(Yes/No): Can an inline statement refer to code labels outside the __asm block?arrow_forward
- (IN C LANGUAGE) Binary-Decimal / Decimal Binary . Between 0 and 255 a number will be decided randomly by computer. Then asks to user 3 times a random digit of binary value of the that number. If user enters wrong number for a digit program will select another random number and ask random times random digit. For example: Computer selected number as 163 ( Which is 10100011) What is the digit 2 (question 1/3) :User enter 1 CorrectWhat is the digit 4 (question 2/3) : User enter 0 CorrectWhat is the digit 7 (question 3/3) : User enter 0 CorrectUser finished the quest with 3 input .arrow_forward(True/False): A function ending with the letter W (such as WriteConsoleW) is designed towork with a wide (16-bit) character set such as Unicodearrow_forward(Cost of driving) Write a program that prompts the user to enter the distance to drive, the fuel efficiency of the car in miles per gallon, and the price per gallon, and dis- plays the cost of the trip.arrow_forward
- (Computer-Assisted Instruction) The use of computers in education is referred to as computer-assisted instruction (CAI). Write a program that will help an elementary school student learn multiplication. Use a Random object to produce two positive one-digit integers. The program should then prompt the user with a question, such as How much is 6 times 7? The student then inputs the answer. Next, the program checks the student’s answer. If it’s correct, display the message "Very good!" and ask another multiplication question. If the answer is wrong, display the message "No. Please try again." and let the student try the same question repeatedly until the student finally gets it right. A separate method should be used to generate each new question. This method should be called once when the application begins execution and each time the user answers the question correctly.arrow_forward(Financials: currency exchange) Write a program that prompts the user to enter the exchange rate from currency in U.S. dollars to Rupees PKR. Prompt the user to enter 0 to convert from U.S. dollars to Rupees PKR and 1 to convert from Rupees PKR and U.S. dollars. Prompt the user to enter the amount in U.S. dollars or Rupees PKR to convert it to Rupees PKR or U.S. dollars, respectively. Use c++ program.arrow_forward(Calculating the Product of Odd Integers) Write a program that calculates and prints theproduct of the odd integers from 1 to 15.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
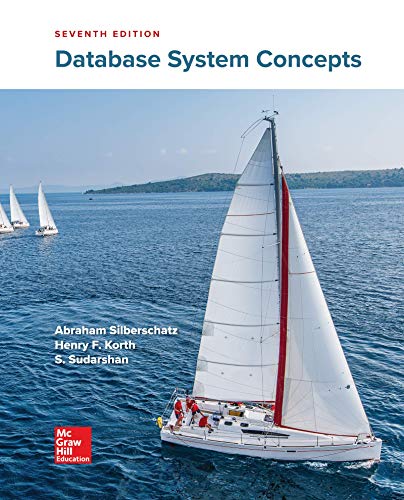
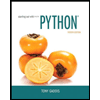
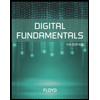
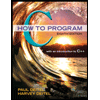
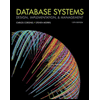
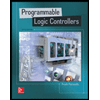