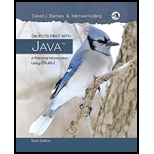
Program plan:
The variables used in the program are given below:
- Player: a class representing the player in the game
- Room: a class representing a room in the game
- Item: a class representing an item in the game
- Command: a class representing a command that can be executed by the player in the game.
The methods used in the program are as follows:
- execute(): executes the take command, which allows the player to pick up an item from the current room and add it to their inventory
- hasSecondWord(): a method inherited from the Command class, which checks whether the player provided a second word (i.e. an item name) with the command
- getRoom(): a method in the Player class, which retrieves the current room that the player is in
- getItem(): a method in the Room class, which retrieves an item from the room by name
- pickUpItem(): a method in the Player class, which attempts to add an item to the player's inventory. It returns true if the item is successfully added, and false if it is too heavy to carry.
- removeItem(): a method in the Room class, which removes an item from the room.
- LookCommand(): a constructor in the LookCommand class, which creates a new LookCommand object with a reference to the current player. This command is used to display the room description and contents after an item has been dropped.
addItem(): a method in the Room class, which adds an item to the room
Program Description:
To implement an extension that allows the player to pick only 1 item.
Program
TakeCommand.java
public class TakeCommand extends Command { private Player player; /& * Constructor for objects of class TakeCommand */ public TakeCommand(Player player) { this.player = player; } public void execute() { if (!hasSecondWord()) { System.out.println("Take what"); return; } Room room = player.getRoom(); Item item = room.getItem(getSecondWord()); if (item != null) { if (player.pickUpItem(item)) { // the item is no longer in the room room.removeItem(item); Command command = new ItemsCommand(player); command.execute(); } else { System.out.println("You cannot possibly pick this up!"); } } else { System.out.println("Take what?"); } } }
DropCommand.java
/& * The player gets an item out of his bag and drops it in to the room. */ public class DropCommand extends Command { private Player player; /& * Constructor for objects of class DropCommand */ public DropCommand(Player player) { this.player = player; } public void execute() { if (!hasSecondWord()) { System.out.println("Drop what"); return; } Item item = player.dropItem(getSecondWord()); if (item != null) { Room room = player.getRoom(); room.addItem(item); Command command = new LookCommand(player); command.execute(); } else { System.out.println("Drop what?"); } } }
Explanation:
The modified "Zuul" game is a text-based adventure game in which the player navigates through a series of rooms, interacting with items and solving puzzles to progress through the game. The game includes two new commands, "take" and "drop," which allow the player to pick up and carry one item at a time. The game is implemented using object-oriented
TakeCommand and DropCommand are two classes in the Zuul project that extend the Command class and allow the player to pick up and drop items in the game. TakeCommand is executed when the player types the "take" command followed by the name of an item in the current room. The command checks if the item is in the room and if it is not too heavy for the player to carry. If both conditions are met, the item is removed from the room and added to the player's inventory. DropCommand is executed when the player types the "drop" command followed by the name of an item in their inventory. The command checks if the item is in the inventory and if so, removes it and adds it to the current room. Both commands use methods in the Player, Room, and Item classes to interact with the game world and modify the state of the player and the game.
OUTPUT:
You are in the living room. Exits: north, east, west Items: couch, lamp, remote control > take lamp You took the lamp. > take remote You took the remote control. > take book Take what? > go north You are in the kitchen. Exits: south Items: fridge, stove, knife > take fridge You cannot possibly pick this up! > take knife You took the knife. > go south You are in the living room. Exits: north, east, west Items: couch, lamp, remote control, knife > drop remote You dropped the remote control. > look You are in the living room. Exits: north, east, west Items: couch, lamp, knife > drop lamp You dropped the lamp. > go east You are in the dining room. Exits: west Items: table, chair > drop chair You cannot drop something that you don't have! > drop knife You dropped the knife. > look You are in the dining room. Exits: west Items: table, chair, knife

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
- when coding a chess game, implement the following method: isInCheck(Side s): Returns true if the king of side s is attacked by any of the opponent’s pieces, i.e., if in the current board state, any of the opponents pieces can move to where the king is. Otherwise, it returns false. Note that this method is only used to warn the player when they are in check. You can use the GUI to test if this is working. public boolean isInCheck(Side side) { // TODO write this method return false; } public enum Side { BLACK, WHITE; public static Side negate(Side s) { return s == Side.BLACK ? Side.WHITE : Side.BLACK; } }arrow_forwardPython: Write a code snippet that imports the tkinter library and creates a new window object root. Add a label widget to the window object root with the text "Hello, World!". Write a code snippet that adds a button widget to the window object root with the text "Click me!", and binds the button to a function button_click() that prints "Button clicked!" to the console. Hint: You may find the Label, Button, and command attributes in tkinter useful for completing parts 2 and 3 Note: For each part of the question, make sure to provide clear instructions and examples for the code snippets. Also, make sure to test your code snippets to ensure that they work as intended.arrow_forwardPlease help me create a cave class for a Hunt the Wumpus game. You can read the rules in it's entirety of the Hunt the Wumpus game online to get a better idea of the specifications. It's an actual game. INFORMATION: The object of this game is to find and kill the Wumpus within a minimum number of moves, before you get exhausted or run out of arrows. There is only one way to win: you must discover which room the Wumpus is hiding in and kill it by shooting an arrow into that room from an adjacent room. The Cave The Wumpus lives in a cave of 30 rooms. The rooms are hexagonal. Each room has up to 3 tunnels, allowing access to 1, 2 or 3 (out of 6) adjacent rooms. The attached diagram shows the layout of rooms in the cave. The map wraps around such that rooms on the edges (white cells) have neighbors from the opposite edge (blue cells). E.g., the neighbors of room 1 are rooms 25, 26, 2, 7, 6, and 30, and you could choose to connect room 1 to any of these rooms. Observe how room 1…arrow_forward
- 2. Write an interface for Tossable. We will say that every Tossable item has a way to toss it and we want to be able to give its color. A Tossable item by default is to throw an assist toss to another player.arrow_forwardProject #5 A deck of cards contains 52 cards with four suits: club, diamond, heart and spade ranging in values from 2, ... to 10, Jack, Queen, King and Ace. Ace has the highest value in the same suit. Cards can be compared using the face value. A card with higher face value is bigger than a card with lower face value. If two cards have the same face value, then the suit determines the order. Club is smaller than diamond which is smaller than heart which is smaller than spade. For example: club 2 < diamond 2arrow_forwardimplement these five methods and main function: 1. getSize() // return the total number of items stored in the bag. Note: DlkdBag does not have itemCount member variable. 2. addFH() //add anEntry from head 3. addFT() //add anEntry from tail 4. display() // display bag items from head to tail 5. clear() // get rid of the bag, and release all memory used by the bag 6. main() // driver code to test all the methods you've implemented Submission: Put everything into a single file, name it as LastNameFirstInitial_a3.cpp (e.g., if your name is John Doearrow_forwardOpen the clock-display project and create a ClockDisplay object by selecting the following constructor: new ClockDisplay() Call its getTime method to find out the initial time the clock has been set to. Can you work out why it starts at that particular time?arrow_forwardPlease add a comment (# this is a comment) on each line or block to briefly explain what it does.arrow_forwardImplement the "Add song" menu item. New additions are added to the end of the list. Ex: ADD SONG Enter song's unique ID: SD123 Enter song's name: Peg Enter artist's name: Steely Dan Enter song's length (in seconds): 237arrow_forwardCan you use it in a scanner so i can copy paste please. Part 1 A regular triangle is one for which all sides are congruent and all interior angles. Create a class RegularTriangle with a field side (int) Create a constructor which accepts an int parameter and will assign the parameter to field side Create assessor and mutator for this class Create a method getPerimeter() which should return 3*side; Part 2 in the constructor, add a logic that if the input parameter is less than 5 then set the side to 5, if it is >10 then set the side to 10, otherwise set the side to the input value. Screenshot final outputarrow_forwardQ2 Create MusicOrganizer project using BlueJ . (i) Create a MusicOrganizer class and declare an ArrayList for storing the file names of music files into the String object. (ii) Create a constructor for the music organizer class and assign the field to the ArrayList. (iii) Write a method to add a file to the collection. (iv) Write a method to return the number of files in the collection. (v) Write a method to remove a file from the collection. (vi) Make a screen shot of the coding and output.arrow_forwardPlease help me create a cave class for a Hunt the Wumpus game (in java). You can read the rules in it's entirety of the Hunt the Wumpus game online to get a better idea of the specifications. It's an actual game. INFORMATION: The object of this game is to find and kill the Wumpus within a minimum number of moves, before you get exhausted or run out of arrows. There is only one way to win: you must discover which room the Wumpus is hiding in and kill it by shooting an arrow into that room from an adjacent room. The Cave The Wumpus lives in a cave of 30 rooms. The rooms are hexagonal. Each room has up to 3 tunnels, allowing access to 1, 2 or 3 (out of 6) adjacent rooms. The attached diagram shows the layout of rooms in the cave. The map wraps around such that rooms on the edges (white cells) have neighbors from the opposite edge (blue cells). E.g., the neighbors of room 1 are rooms 25, 26, 2, 7, 6, and 30, and you could choose to connect room 1 to any of these rooms. Observe how…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
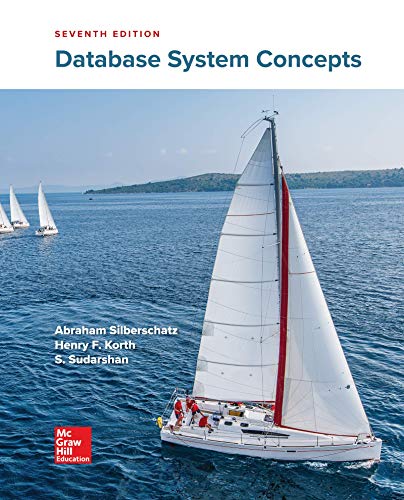
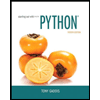
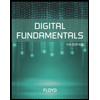
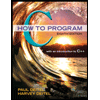
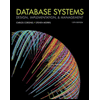
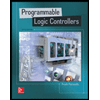