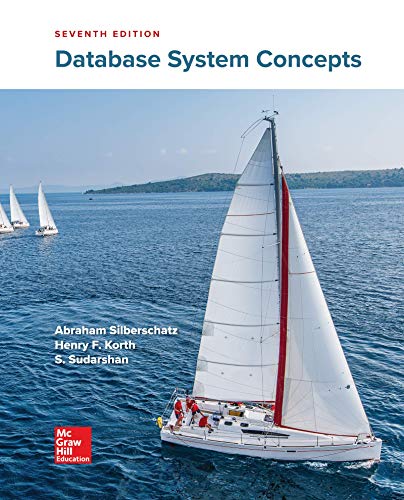
Change the following code so that it checks the following 3 conditions:
1. there is no space between each cells (imgs)
2. even if it is resized, the components wouldn't disappear
3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game.
Main():
Labyrinth labyrinth = new Labyrinth(10);
Player player = new Player(9, 0);
Dragon dragon = new Dragon(9, 9);
JFrame frame = new JFrame("Labyrinth Game");
GameGUI gui = new GameGUI(labyrinth, player, dragon);
frame.add(gui);
frame.setSize(600, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
public class GameGUI extends JPanel {
private final Labyrinth labyrinth;
private final Player player;
private final Dragon dragon; //labyrinth, player, dragon are just public classes
private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");
private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png");
private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png");
private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png");
public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) {
this.labyrinth = labyrinth;
this.player = player;
this.dragon = dragon;
setFocusable(true);
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
char move = switch (e.getKeyCode()) {
case KeyEvent.VK_W -> 'W';
case KeyEvent.VK_S -> 'S';
case KeyEvent.VK_A -> 'A';
case KeyEvent.VK_D -> 'D';
default -> ' ';
};
if (move != ' ') {
player.move(move, labyrinth);
dragon.move(labyrinth);
repaint();
checkGameState();
}
}
});
}
private void checkGameState() {
if (player.getX() == labyrinth.getSize() - 1 && player.getY() == labyrinth.getSize() - 1) {
JOptionPane.showMessageDialog(this, "You escaped! Congratulations!");
System.exit(0);
}
if (Math.abs(player.getX() - dragon.getX()) <= 1 &&
Math.abs(player.getY() - dragon.getY()) <= 1) {
JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over.");
System.exit(0);
}
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int cellSize = 50;
for (int i = 0; i < labyrinth.getSize(); i++) {
for (int j = 0; j < labyrinth.getSize(); j++) {
if (labyrinth.getCell(i, j).isWall()) {
wallIcon.paintIcon(this, g, j * cellSize, i * cellSize);
} else if(!labyrinth.getCell(i, j).isWall()){
emptyIcon.paintIcon(this, g, j * cellSize, i * cellSize);
}
}
}
playerIcon.paintIcon(this, g, player.getY() * cellSize, player.getX() * cellSize);
dragonIcon.paintIcon(this, g, dragon.getY() * cellSize, dragon.getX() * cellSize);
}
}
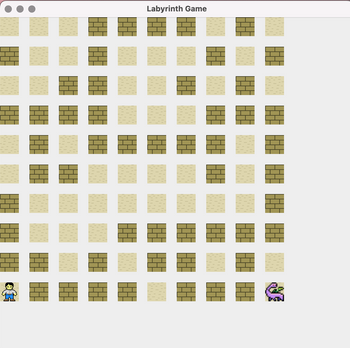
to generate a solution
a solution
- World computer language allows logic to be run in a browser? Java JavaScript HTML SQLarrow_forwardOpenGL - computer grahicarrow_forwardYour computer science class assignment required you to make connections between theoretical ideas and the world beyond the classroom. What did you do about it?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
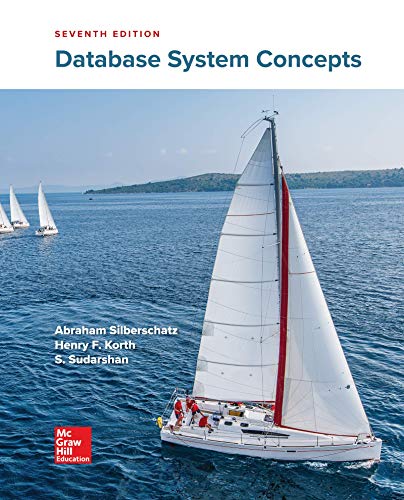
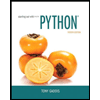
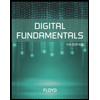
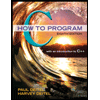
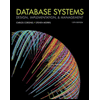
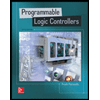