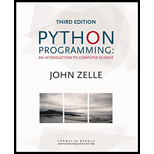
Concept explainers
Validation of date input
Program plan:
- Define the “leap_Year()” function,
- Check whether the year is not divisible by "4",
- If the condition is “True”, return “False”.
- Otherwise, check whether the year is divisible by "100",
- If it is “True”, check whether the year is divisible by "400",
- If it is “True”, return “True”.
- Otherwise, return “False”.
- Otherwise, return “True”.
- If it is “True”, check whether the year is divisible by "400",
- Check whether the year is not divisible by "4",
- Define the “main()” function,
- Execute the “try” statement to raise the exception if it is occurred.
- Get the input from the user.
- Split the user input based on "/" using “split()”.
- Convert the string type inputs into integer type.
- Check whether either the month is greater than “12” or day is greater than “12”,
- If it is “True”, print the string “This date is invalid”
- Otherwise, check whether the day is less than or equal to “28”,
- If it is “True”, print the string as “This date is valid”.
- Otherwise, check whether the month is "2" and "29",
-
- If it is “True”, check whether the Boolean value return from “leap_Year()” is “False”.
- If it is “True”, print “This date is invalid”.
- Otherwise, print “This date is valid”.
- Otherwise, check whether the day is “31”,
- If it “True”, check whether the month is either "2" or "4" or "6" or "11",
- If the above condition is “True”, print invalid.
- Otherwise, print valid.
- Otherwise, print “This date is valid”.
- If it is “True”, check whether the Boolean value return from “leap_Year()” is “False”.
- Catch the exception using “except ValueError” statement, if the exception of type “ValueError” occurs.
- Handle the exception.
- Catch the exception using “except” statement, if any type of exception occurs.
- Call the function “main()”.
- Execute the “try” statement to raise the exception if it is occurred.

This Python program is to add decisions and exception handling as required making it truly robust.
Explanation of Solution
Program:
File name: “year.py”
#Define the function
def leap_Year(yr):
#Check whether the year is not divisible by "4"
if (yr % 4) != 0:
#Return the boolean value
return False
#Otherwise
else:
#Check whether the year is divisible by "100"
if (yr % 100) == 0:
#Check whether the year is divisible by "400"
if (yr % 400) ==0:
#Return boolean value
return True
#Otherwise
else:
#Return boolean value
return False
#Otherwise
else:
#Return boolean value
return True
#Define the function
def main():
#Make a try
try:
#Get the input from the user
date_Str = input("Enter a date in the form MM/DD/YYYY: ")
#Split the user input based on "/" using split()
month_Str, day_Str, year_Str = date_Str.split("/")
#Convert the string into integer
mon = int(month_Str)
#Convert the string into integer
d = int(day_Str)
#Convert the string into integer
yr = int(year_Str)
#Execute the condition
if mon > 12 or d > 31:
#Print the string
print("This date is invalid.")
#Otherwise
else:
#Check whether the day is less than or equal to 28
if d <= 28:
#Print the string
print("This date is valid.")
#Check whether the month is "2" and "29"
elif mon == 2 and d == 29:
#Check whether the boolean value return from "leap_Year()" is "False"
if leap_Year(yr) == False:
#Print the string
print("This date is invalid.")
#Otherwise
else:
#Print the string
print("This date is valid.")
#Check whether the day is "31"
elif d == 31:
'''Check whether the month is either "2" or "4" or "6" or "11"'''
if mon == 2 or 4 or 6 or 11:
#Print the string
print("This date is invalid")
#Otherwise
else:
#Print the string
print("This date is valid")
#Otherwise
else:
#Print the string
print("The date is valid.")
#Catch the exception
except ValueError:
#Handle the exception
print("Your input was not in the correct form.")
#Catch the exception
except:
#Handle the exception
print("Something went wrong!")
#Call the function
main()
Output:
Enter a date in the form MM/DD/YYYY: 02/30/2000
The date is valid.
>>>
Additional output:
Enter a date in the form MM/DD/YYYY: 02-05-2000
Your input was not in the correct form.
>>>
Additional output:
Enter a date in the form MM/DD/YYYY: 06/31/2000
This date is invalid
>>>
Want to see more full solutions like this?
Chapter 7 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- What is exception propagation? Give an example of a class that contains at least two methods, in which one method calls another. Ensure that the subordinate method will call a predefined Java method that can throw a checked exception. The subordinate method should not catch the exception. Explain how exception propagation will occur in your example.arrow_forwardThere are three clauses in Java’s Exception Handling. What are they? What are they for? Is it possible to create your own exception? Why would you think you might need it?arrow_forwardUse inheritance in JAVA to create an exception superclass(called ExceptionA) and exception subclasses ExceptionB and ExceptionC, where ExceptionB inherits from ExceptionA and ExceptionC inherits from ExceptionB. Write a program to demonstrate that the catch block for type ExceptionA catches exceptions of types ExceptionB and ExceptionC.arrow_forward
- hi it's been 6 days now I still haven't got an answer to the previous question which is: ("Hi, can you include all OOP concepts (Encapsulation, Inheritance, Abstraction, Polymorphism) and also include Exception handling?")arrow_forwardWhat exactly does it imply when someone overloads an operator or a function? What are the positive aspects of it? How can the user be safeguarded from making errors via the usage of various exception handling strategies that may be programmed?arrow_forwardWhat is meant by operator and function overloading? What are the advantages What exception handling techniques can be programmed to protect the user from making mistakes?arrow_forward
- please answer in java How do exceptions and their accompanying try/catch statements occur in the real world? Let's take some time to discuss how what we have learned in this module is represented in our everyday lives. Try to separate yourself from the actual programmatic code and look for the high-level concepts in your day to day life or in your discipline. Please write a response on how exceptions and their accompanying try/catch statements are represented in a real-world setting. Feel free to use industry-specific examples and be creative.arrow_forwardWhat is the importance of exception handling? Explain the use of try, catch and finally in exception handling with the help of example.arrow_forwardIn regard to developing appropriate exceptions in Java, what are some best practices to follow?arrow_forward
- b. Suppose you are a professional java freelancer in Upwork where you completed several client projects. Most of the projects are on basic java programming. Since you learned the OOP feature in the java language so you are bidding for advanced java project. After a few days later you got a project which has OOP feature but it is very simple. Your task is to identify the workflow of the java program. This java program is on exception handling and there have multiple catch blocks match the type of thrown object. Your client can't understand what happen or what will be running order of the java program. Now discuss your client project based on a java program as a client program.arrow_forward1. Write a program to demonstrate exception propagation in java?arrow_forwardIf a programming language does not provide exception-handling facilities, it is common to have subprograms indicate an error either through return value or through an error out parameter. What are some disadvantages of this approach comapared to exception-handling facilities provided by programming languages such as C++ and Java?arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage