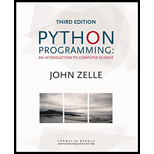
Concept explainers
Calculate whether a date is valid
Program plan:
- Define the function “isLeapYear()”,
- Check whether the year is not divisible by "4",
- If it is true, return “False”.
- Otherwise, check whether the year is divisible by "100",
- If it is true, check whether the year is divisible by "400",
- If it is true, return “True”.
- Otherwise, return “False”.
- Otherwise, return “True”.
- If it is true, check whether the year is divisible by "400",
- Check whether the year is not divisible by "4",
- Define the function “main()”,
- Get the date from the user.
- Assign month, day, and year by splitting the date by "/" using “split()” method
- Typecast month, day, and year to “int” type.
- Check whether either a month is greater than "12" or day is greater than "31",
- If it is true, prints date as invalid.
- Otherwise, Check whether day is less than or equal to "28",
- If the condition is “True”, print as valid.
- Otherwise, check whether month is "2" and day is "29",
- If it is “True” Check whether the return value from “isLeapYear()” is “False”,
- If “True” print invalid.
- Otherwise, print valid.
- Otherwise, check whether day is equal to “31”,
- If “True”, Check whether month is either "2" or "4" or "6" or "11",
- If “True” print invalid.
- Otherwise, print valid.
- Otherwise, print valid.
- If “True”, Check whether month is either "2" or "4" or "6" or "11",
- Call the function “main()”.

This Python program accepts a date in the form month / day / year and prints whether the date is valid or not.
Explanation of Solution
Program:
File name: “Leap.py”
#Define the function isLeapYear()
def isLeapYear(y):
#Check whether the year is not divisible by "4"
if (y % 4) != 0:
#Return false
return False
#Otherwise
else:
#Check whether the year is divisible by "100"
if (y % 100) == 0:
#Check whether the year is divisible by "400"
if (y % 400) ==0:
#Return true
return True
#Otherwise
else:
#Return false
return False
#Otherwise
else:
#Return true
return True
#Define the function main()
def main():
#Get the date from the user
date=eval(input("Enter date"))
'''Assign month, day, and year by splitting the date by "/" using split() method'''
month_Str, day_Str, year_Str = date.split("/")
#Typecast month to int type
mon = int(month_Str)
#Typecast day to int type
d = int(day_Str)
#Typecast year to int type
yr = int(year_Str)
'''Check whether either a month is greater than "12" or day is greater than "31"'''
if mon > 12 or d > 31:
#Print a message
print("This date is invalid.")
#Otherwise
else:
#Check whether day is less than or equal to "28"
if d <= 28:
#Print a message
print("This date is valid.")
#check whether month is "2" and day is "29"
elif mon == 2 and d == 29:
#Check whether the return value is false
if isLeapYear(yr) == False:
#Print a message
print("This date is invalid.")
#Otherwise
else:
#Print a message
print("This date is valid.")
#Check whether day is "31"
elif d == 31:
'''Check whether month is either "2" or "4" or "6" or "11"'''
if mon == 2 or 4 or 6 or 11:
#Print date is invalid
print("This date is invalid")
#Otherwise
else:
#Print date is valid
print("This date is valid")
#Otherwise
else:
#Print dte is valid
print("The date is valid.")
#Call the function main()
main()
Output:
Enter date'6/29/1200'
The date is valid.
>>>
Additional Output:
Enter date'12/32/1500'
This date is invalid.
>>>
Additional Output:
Enter date'13/28/1999'
This date is invalid.
>>>
Want to see more full solutions like this?
Chapter 7 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Write a program that outputs all possibilities to put + or - or nothing between the numbers 1,2,…,9 (in this order) such that the result is 100. For example 1 + 2 + 3 - 4 + 5 + 6 + 78 + 9 = 100.arrow_forwardWrite a program that takes in a line of text as input, and outputs that line of text in reverse. The program repeats, ending when the user enters "Quit", "quit", or "q" for the line of text. Ex: If the input is: Hello there Hey quit then the output is: ereht olleH yeHarrow_forwardWrite a program that reads an integer age of kids, finds the maximum of them, and counts its occurances, finds the minimum of them, and counts its occurances. And Find the average ages. Assum that the input ends with number -1.arrow_forward
- Write a program that takes in a line of text as input, and outputs that line of text in reverse. The program repeats, ending when the user enters "Quit", "quit", or "q" for the line of text.arrow_forwardWrite a program that reads in hours, minutes, as input, and outputs the time in minutes only.arrow_forwardWrite a program that asks the user how many credit units they have taken. If they have taken 23 or less, print that the student is a freshman. If they have taken between 24 and 53, print that they are a sophomore. The range for juniors is 54 to 83, and for seniors it is 84 and over.arrow_forward
- Write a program that inputs a number and checks whether it is a perfect number or not. A perfect number is the number that is numerically equal to the sum of its divisors. For example, 6 is a perfect number because the divisors of 6 are 1, 2, 3 and 1+2+3=6arrow_forwardUsing Paython Write a program that asks a student for his grade (out of 100) in 3 exams and then print out his final grade (out of 100), given that the weight of the first exam is 30%, the second 30%, and the third 40%.Example Input:Exam1: 70Exam2: 80Exam3: 90Example Output:Total Grade = 81Explanation: 0.3 * 70 + 0.3 * 80 + 0.4 * 90 = 81arrow_forwardWrite a program that prompts for and reads the radius and the height of a cylinder (in centimeters). It then computes its volume by using pass by reference and pass by valuearrow_forward
- Write a program that will Input the name , position of the employee , Input the rate per hour, hours worked. Compute the Gross pay for an employee.arrow_forwardWrite a program that takes a date as input and outputs the date's season. The input is a string to represent the month and an int to represent the day. Ex: If the input is: April 11 the output is: Springarrow_forwardwrite a program that takes in an integer in the range 20 to 98 is input the output is a countdown starting from the integer and stopping when both output digits are identicalarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
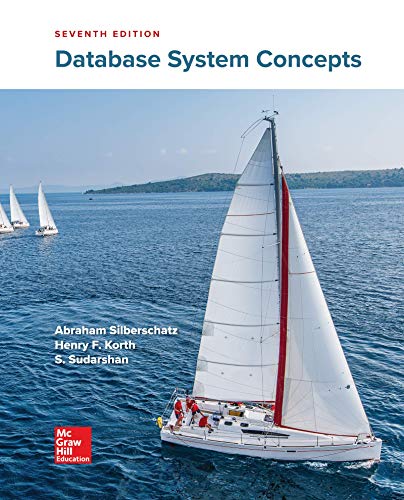
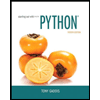
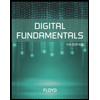
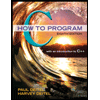
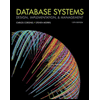
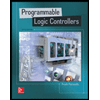