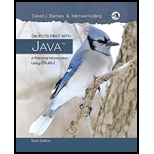
Concept explainers
What is wrong with the following version of the constructor of TIcketMachine?
Try out this version in the better-ticket-machine project. Does this version compile? Create an object and then inspect its fields. Do you notice something wrong about the value of the price field in the inspector with this version? Can you explain why this is?

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Modern Database Management (12th Edition)
Starting Out with C++: Early Objects (9th Edition)
Starting Out with Python (4th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- I need to figure out how to call a method that is located in a different class. For example, I am trying to make a tax calculator that multiplies a payers income by a corporate rate. However, I cannot figure out how to call the income method. Nothing I have tried is working. Is there any chance you can guide me? I am just trying to make this equation work. Is there something else that is stopping this from working?arrow_forwardSELECT ALL the members of the class that are accessible by classes in the same package as Bicycle. Take note: In your response, leave off the constructor(s).arrow_forwardCreate a new project for this program called TestOldMaid and add a class with a main() method. In the project: Copy your Deck and Card class from the earlier project into it. Create a subclass of Deck called OldMaidDeck. It is special because one of the Queens is missing so it only has 51 cards. Create a constructor method that calls the super class constructor, then removes a queen. Override the toString method so it returns the name of the deck and the number of cards in it. Write the test main() method. Create an OldMaidDeck object and deal all the cards to six players. It is ok if not everyone has an equal number of cards. Use arrays or ArrayLists for the players hands. Show the hands of all 6 players. Refer to the web to find out more about the Old Maid card game: https://bicyclecards.com/how-to-play/old-maid/ Fully document all classes with your name, date and description. And each data member and method is documented. Each block that does something is also documented.arrow_forward
- Create a new project for this program called TestOldMaid and add a class with a main() method. In the project: Copy your Deck and Card class from the earlier project into it. Create a subclass of Deck called OldMaidDeck. It is special because one of the Queens is missing so it only has 51 cards. Create a constructor method that calls the super class constructor, then removes a queen. Override the toString method so it returns the name of the deck and the number of cards in it. Write the test main() method. Create an OldMaidDeck object and deal all the cards to six players. It is ok if not everyone has an equal number of cards. Use arrays or ArrayLists for the players hands. Show the hands of all 6 players. Refer to the web to find out more about the Old Maid card game. Fully document all classes with your name, date and description. And each data member and method is documented. Each block that does something is also documented. Without removing the Queensarrow_forwardCan you implement the Student class using the concepts of encapsulation? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first. You are given a Student class in the editor. Your task is to add two fields: ● String name ● String rollNumber and provide getter/setters for these fields: ● getName ● setName ● getRollNumber ● setRollNumber Implement this class according to the rules of encapsulation. Input # Checking all fields and getters/setters Output # Expecting perfectly defined fields and getter/setters. There is no need to add constructors in this class.arrow_forwardHelp please: Write the definition of a class swimmingPool, to implement the properties of a swimming pool. Your class should have the instance variables to store the length (in feet), width (in feet), depth (in feet), the rate (in gallons per minute) at which the water is filling the pool, and the rate (in gallons per minute) at which the water is draining from the pool. Add appropriate constructors to initialize the instance variables. Also, add member functions to do the following: determine the amount of water needed to fill an empty or partially filled pool, determine the time needed to completely or partially fill or empty the pool, and add or drain water for a specific amount of time, if the water in the pool exceeds the total capacity of the pool, output "Pool overflow" to indicate that the water has breached capacity. exisiting code in different file: const double GALLONS_IN_A_CUBIC_FEET = 7.48; class swimmingPool { public: void set(double l = 0, double w = 0, double…arrow_forward
- .If the name of getBalance is changed to getAmount, does the return statement in the body of the method also need to be changed for the code to compile? Try it out within BlueJ. What does this tell you about the name of an accessor method and the name of the field associated with it?arrow_forwardAdd a constructor to the Point class that accepts another Point as a parameter and initializes this new Point to have the same (x, y) values. Use the keyword this in your solution.arrow_forwardPlease help me create a cave class for a Hunt the Wumpus game (in java). You can read the rules in it's entirety of the Hunt the Wumpus game online to get a better idea of the specifications. It's an actual game. INFORMATION: The object of this game is to find and kill the Wumpus within a minimum number of moves, before you get exhausted or run out of arrows. There is only one way to win: you must discover which room the Wumpus is hiding in and kill it by shooting an arrow into that room from an adjacent room. The Cave The Wumpus lives in a cave of 30 rooms. The rooms are hexagonal. Each room has up to 3 tunnels, allowing access to 1, 2 or 3 (out of 6) adjacent rooms. The attached diagram shows the layout of rooms in the cave. The map wraps around such that rooms on the edges (white cells) have neighbors from the opposite edge (blue cells). E.g., the neighbors of room 1 are rooms 25, 26, 2, 7, 6, and 30, and you could choose to connect room 1 to any of these rooms. Observe how…arrow_forward
- You are going to be working with class Rational which defines rational number objects. A rational number is a number that can be written as a fraction. A rational number consists of a numerator and a denominator (which cannot be 0). Rational numbers also have the ability to be displayed in an original form, decimal form or reduced form. You are going to be completing various object methods to perform the tasks previously list. You are to begin with the Rational Class and RationalTest classes provided. Make all modifications outlined in the point values below. Pay close attention to the names of the attributes, methods and return values. You are given starter files and the test files necessary. Only modify class Rational and keep the function headers provided to you for all of Part 1. You will need to add your own methods for Part 2. Part 1a This version requires that you complete the constructor, getOriginal(), and getDecimal() methods of class Rational. Constructor: at this stage…arrow_forwardWrite the code for the timeTick method in ClockDisplay that displays hours, minutes, and seconds, or even implement the whole class if you wish.arrow_forwardWith the addition of electric cars, we have a need to create a subclass of our Car class. In this exercise, we are going to create the Electric Car subclass so that we can override the miles per gallon calculation since electric cars don’t use gallons of gas. The Car class is complete, but you need to complete the ElectricCar class as outlined in the starter code with comments. Once complete, use the CarTester to create both a Car and ElectricCar object and test these per the instructions in the CarTester class. public class CarTester{public static void main(String[] args){// Create a Car object// Print out the model// Print out the MPG// Print the object// Create an ElectricCar object// Print out the model// Print out the MPG// Print the object}} public class Car { //This code is completeprivate String model;private String mpg; public Car(String model, String mpg){this.model = model;this.mpg = mpg;} public String getModel(){return model;} public String getMPG(){return mpg;} public…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
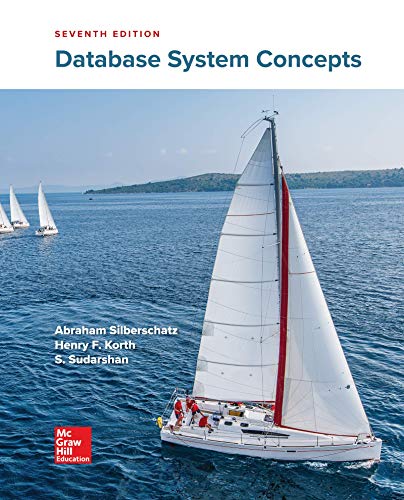
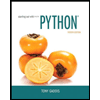
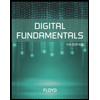
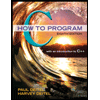
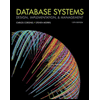
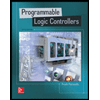