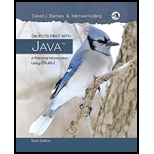
Concept explainers
Rewrite the printTicket method so that it declares a local variable, amountLeftloPay. This should then be initialized to contain the difference between price and balance. Rewrite the test in the conditional statement to check the value of amountLeftloPay. If its value is less than or equal to zero, a ticket should be printed; otherwise, an error message should be printed stating the amount left to pay. Test your version to ensure that it behaves in exactly the same way as the original version. Make sure that you call the method more than once, when the machine is in different states, so that both parts of the conditional statement will be executed on separate occasions.

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Introduction To Programming Using Visual Basic (11th Edition)
Degarmo's Materials And Processes In Manufacturing
Management Information Systems: Managing The Digital Firm (16th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- This method simulates the playing of one round of “Rock Paper Scissors”. In this strange version of the game, if both players have the same hand gestures, the return value is 1. If they have different hand gestures, the return value is a 0. If at least one player, provides a string argument that is anything other than rock, paper, or scissors, the return value is -1. The method should not be case-sensitive. For example, rock, ROCK, Rock, and RocK are all valid. The output is in the image I addedarrow_forwardPlease do it with comments.arrow_forwardYour code should support the following: By default, the car's wheels should start with a pressure of 30. Every time the car drives the wheel pressure should drop by 5. The fillTires method should fill the wheels back to a pressure of 30. The Wheel class should not allow the pressure to go below 0. Any an attempt to set the pressure to a negative value should set the pressure to 0 instead. The toString methods should be written so they match the expected output of the provide Main class. The output produced by the Main class should be: Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30) Car (FR: Pressure = 20, FL: Pressure = 20, BR: Pressure = 20, BL: Pressure = 20) Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30) Car (FR: Pressure = 15, FL: Pressure = 15, BR: Pressure = 15, BL: Pressure = 15) Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30) Car (FR: Pressure = 0, FL: Pressure = 0, BR:…arrow_forward
- You are given a Conversion class and need to complete it by adding two static methods. The first method should be called gallonsToPints and take a double gallons and return the number of pints as a double (1 gallon = 8 pints). The second method should be called feetToInches, also taking a double number of feet and returning the number of inches as a double (1 foot = 12 inches). Test your program in the ConversionTester class by printing out two conversions. In javaarrow_forwardWrite a main method, in which you should have an Arralylist of students, in which you should include every student. You should have a PhD student named Joe Smith. In addition, you should create an Undergraduate student object for each of your team members (setting their names). If you’re only one person doing the assignment, then you should just create one undergraduate object. For each undergraduate student, you should add add two courses (COIS 2240 and COIS 1020). Loop over the arraylist of students, print their names, if the student is an undergraduate student, print their courses.arrow_forwardWrite a method lastNameFirst that takes a string containing a name such as "Harry Smith" or "Mary Jane Lee", and that returns the string with the last name first, such as "Smith, Harry" or "Lee, Mary Jane".arrow_forward
- Write an expression that will cause the following code to print "Equal" if the value of sensorReading is "close enough" to targetValue. Otherwise, print "Not equal". Hint: Use epsilon value 0.0001. Ex: If targetValue is 0.3333 and sensorReading is (1.0/3.0), output is:arrow_forwardProblem: To write the JUnit test cases for our methods, you need to create two test methods: testLeapYear: This method should test if the isLeapYear method returns true for a given leap year. For example, you can choose the year 2020 and verify if the method returns true for it. If it does, the test will pass. If it doesn't, the test will fail. testNotLeapYear: This method should test if the isLeapYear method returns false for a given non-leap year. For example, you can choose the year 1900 and verify if the method returns false for it. If it does, the test will pass. If it doesn't, the test will fail. --------------------------------------------------------------------------------------------------------------------------------------------------------- import static org.junit.jupiter.api.Assertions.assertTrue; import org.junit.jupiter.api.Test; public class LeapYearTest { // Test case to check if a year is a leap year @Test public void testLeapYear() { //create…arrow_forwardThe L&L Bank can handle up to 30 customers who have savings accounts. Design and implement a program that manages the accounts. Keep track of key information, and allow each customer to make deposits and withdrawals. Produce appropriate error messages for invalid transactions. Hint: You may want to baseyour accounts on the Account class from Chapter 5. Also provide a method to add 3 percent interest to all accounts whenever the method is invoked.arrow_forward
- There is a children’s game called FizzBuzz where players take turns counting but replace the numbers that are multiples of 3 with the word ‘fizz’, numbers that are multiples of 5 with ‘buzz’, and numbers that are multiples of both 3 and 5 with ‘fizzbuzz’. Write the code you’d include in the main method to print out the numbers between 1 and 100 according to the rules of this game. For example, the output would look something like this: 1 2 fizz 4 buzz fizz 7 8 fizz buzz 11 fizz 13 14 fizzbuzz 16 17 fizz 19 buzz fizz 22 23 fizz buzz 26 fizz 28 29 fizzbuzz 31 32 fizz 34 buzz fizz 37 38 fizz buzz 41 fizz 43 44 fizzbuzz 46 47 fizz 49 buzz fizz 52 53 fizz buzz 56 fizz 58 59 fizzbuzz 61 62 fizz 64 buzz fizz 67 68 fizz buzz 71 fizz 73 74 fizzbuzz 76 77 fizz 79 buzz fizz 82 83 fizz buzz 86 fizz 88 89 fizzbuzz 91 92 fizz 94 buzz fizz 97 98 fizz buzz in javaarrow_forwardAdd a new Java class to your project by right clicking on the src folder in the Project pane and select New > Java Class.Enter Hours as the name for this class, and IntelliJ will add it to the files in the src folder for your project. Remember tocopy and insert the standard heading for the main method as you did for Program 2.Write a program that prompts the user to enter a number of hours, and produces the equivalent number of days as a wholenumber along with the number of hours left over. Assume that a day has exactly 24 hours. The following example showsthe format to use for your output, where the input entered by the user is underlined.Enter the number of hours: 100100 hours is 4 days, plus 4 hours.Define Variables: The number of hours will be a whole number, and we would like to obtain results as whole numbers ofdays and hours, so use the int data type to declare the variables to hold the numbers of hours input, as well as the results(the number of days and number of hours left…arrow_forwardWrite a method using the following header that determines whether the day of the month is a working day and will return "Working Day" and "Non-working Day" accordingly. The method accepts two parameters: day of week for the 1" day of the month and day of the month (you can assume each month has exactly 30 days). If the given day of the month is not a valid number, the method should return "Incorrect Day of Month". If the given initDayOfWeek is not a valid number, the method should return "Incorrect Initial Day of Week" If both numbers are incorrect, only return “Incorrect Initial Day of Week". public static String isWorkingDay (int initDayOfWeek, int dayNum) (To make the day of week easier to be represented in the program, we use int number 0 to represent Sunday, 1 to represent Monday, 2 to represent Tuesday ... and 6 to represent Saturday) Examples: isWorkingDay (0, 1) returns "Non-working Day". (As 1" day of the month is Sunday) isWorkingDay (1, 2) returns "Working Day" . (As 1ª day…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
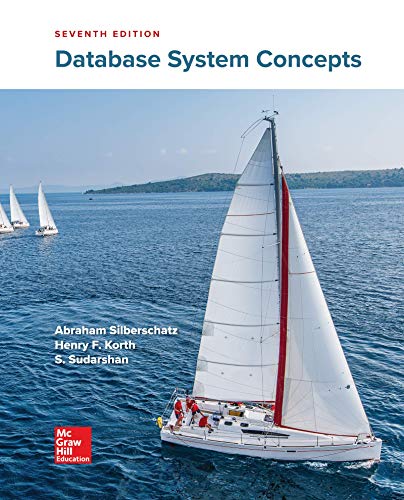
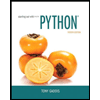
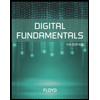
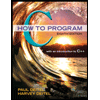
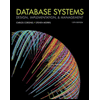
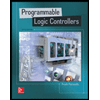