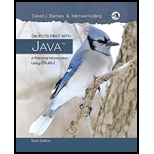
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
6th Edition
ISBN: 9780134477367
Author: David J. Barnes, Michael Kolling
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 2, Problem 22E
Challenge exercise The following object creation will result in the constructor of the Date class being called. Can you write the constructor's header?
new Date ("March", 23, 1861)
Try to give meaningful names to the parameters.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Complete method __init__ in the following class. (It's only missing an assignment to a radius instance variable.)
Finish the TestPlane class that contains a main method that instantiates at least two Planes. Add instructions to instantiate your favorite plane and invoke each of the methods with a variety of parameter values to test each option within each method. To be able to test the functionality of each phase, you will add instructions to the main method in each phase.
Can you call a constructor from another constructor? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first.
Problem Statement#
A class can have multiple parameterized constructors which can call each other.
You are given a partially completed code of a Car class in the editor. Modify the parametrized constructor which assigns parameters to the required fields.
Input#
carName, carModel, carCapacity
Output#
carName, carModel, carCapacity
Sample Input#
"Ferrari", "F8", "100"
Sample Output#
"Ferrari", "F8", "100"
Part of solution
// Car class
class Car {
// Private Fields
private String carName;
private String carModel;
private String carCapacity;
// Default Constructor
public Car() {
this.carName = "";
this.carModel = "";
this.carCapacity = "";
}
// Parameterized Constructor 1
public Car(String name, String model) {
this.carName = name;
this.carModel = model;
}
// Parameterized Constructor 2
public…
Chapter 2 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Ch. 2 - Create a TicketMachine object on the object bench...Ch. 2 - What value is returned if you get the machine's...Ch. 2 - Prob. 3ECh. 2 - Prob. 4ECh. 2 - Create another ticket machine for tickets of a...Ch. 2 - Prob. 6ECh. 2 - Does it matter whether we write...Ch. 2 - Prob. 8ECh. 2 - Put back the word public, and then check whether...Ch. 2 - From your earlier experimentation with the ticket...
Ch. 2 - Prob. 11ECh. 2 - Prob. 12ECh. 2 - Prob. 13ECh. 2 - Prob. 14ECh. 2 - In the following field declaration from the...Ch. 2 - Prob. 16ECh. 2 - Prob. 17ECh. 2 - To what class does the following constructor...Ch. 2 - How many parameters does the following constructor...Ch. 2 - Prob. 20ECh. 2 - Suppose that the class Pet has a field called name...Ch. 2 - Challenge exercise The following object creation...Ch. 2 - Compare the header and body of the getBalance...Ch. 2 - If a call to getPrice can be characterized as...Ch. 2 - If the name of getBalance is changed to getAmount,...Ch. 2 - Prob. 26ECh. 2 - Try removing the return statement from the body of...Ch. 2 - Compare the method headers of getPrice and...Ch. 2 - Do the insertMoney and printTicket methods have...Ch. 2 - Create a ticket machine with a ticket price of...Ch. 2 - How can we tell from just its header that setPrice...Ch. 2 - Complete the body of the setPrice method so that...Ch. 2 - Prob. 33ECh. 2 - Is the increase method a mutator? If so, how could...Ch. 2 - Prob. 35ECh. 2 - Write down exactly what will be printed by the...Ch. 2 - Add a method called prompt to the TicketMachine...Ch. 2 - Prob. 38ECh. 2 - What about the following version?
Ch. 2 - Prob. 40ECh. 2 - Add a showPrice method to the TicketMachine class....Ch. 2 - Create two ticket machines with differently priced...Ch. 2 - Modify the constructor of TicketMachine so that it...Ch. 2 - Give the class two constructors. One should take a...Ch. 2 - Implement a method, empty, that simulates the...Ch. 2 - Prob. 46ECh. 2 - Predict what you think will happen if you change...Ch. 2 - Rewrite the if-else statement so that the method...Ch. 2 - Prob. 49ECh. 2 - In this version of printTicket, we also do...Ch. 2 - Is it possible to remove the else part of the...Ch. 2 - After a ticket has been printed, could the value...Ch. 2 - So far, we have introduced you to two arithmetic...Ch. 2 - Write an assignment statement that will store the...Ch. 2 - Write an assignment statement that will divide the...Ch. 2 - Prob. 56ECh. 2 - Modify your answer to the previous exercise so...Ch. 2 - Why does the following version of refundBalance...Ch. 2 - What happens if you try to compile the...Ch. 2 - What is wrong with the following version of the...Ch. 2 - Add a new method, emptyMachine, that is designed...Ch. 2 - Rewrite the printTicket method so that it declares...Ch. 2 - Challenge exercise Suppose we wished a single...Ch. 2 - List the name and return type of this method:
Ch. 2 - Prob. 65ECh. 2 - Write out the outer wrapping of a class called...Ch. 2 - Write out definitions for the following fields:
Ch. 2 - Write out a constructor for a class called Module....Ch. 2 - Prob. 69ECh. 2 - Correct the error in this method:...Ch. 2 - Write an accessor method called getName that...Ch. 2 - Write a mutator method called setAge that takes a...Ch. 2 - Write a method called printDetails for a class...Ch. 2 - Draw a picture of the form shown in Figure 2.3,...Ch. 2 - Prob. 75ECh. 2 - Create a Student with name "djb" and id "859012"....Ch. 2 - Prob. 77ECh. 2 - Challenge exercise Modify the getLoginName method...Ch. 2 - Consider the following expressions. Try to predict...Ch. 2 - Open the Code Pad in the better-ticket-machine...Ch. 2 - Now add the following in the Code Pad:...Ch. 2 - Add the following: t1.InsertMoney500; What would...Ch. 2 - Prob. 83ECh. 2 - Prob. 84ECh. 2 - Add a field, pages, to the Book class to store the...Ch. 2 - Are the Book objects you have implemented...Ch. 2 - Add a method, printDetails, to the Book class....Ch. 2 - Add a further field, refNumber, to the Book class....Ch. 2 - Modify your printDetai 1 s method to include...Ch. 2 - Prob. 90ECh. 2 - Add a further integer field, borrowed, to the Book...Ch. 2 - Prob. 92ECh. 2 - Prob. 93ECh. 2 - Prob. 94E
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Given that y=ax3+7, which of the following are correct Java statements for this equations? int y = a x x x +...
Java How To Program (Early Objects)
Why doesn't the following pseudocode module work as indicated in the comments? // The readFile method accepts a...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
What is the difference between main memory and secondary storage?
Starting Out With Visual Basic (8th Edition)
Employee and ProductionWorker Classes Create an Employee class that has properties for the following data: Empl...
Starting out with Visual C# (4th Edition)
Using pseudocode similar to the Java class syntax of Figure 8.27, sketch a definition of an abstract data type ...
Computer Science: An Overview (12th Edition)
Demonstrate each of the anomaly types with an example.
Modern Database Management (12th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Why is it important for a class to have a destructor implemented? With your comment, fill in the spaces.arrow_forwardAdd a list of procedures in your patient class, fill them and print them with the patient data. This is called composition which is a "has a" relationship. A patient has procedures. Write a class named Patient that has attributes for the following data: First name, middle name, and last name Address, city, state, and ZIP code Phone number Name and phone number of emergency contact The Patient class’s _ _init_ _ method should accept an argument for each attribute. The Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: Name of the procedure Date of the procedure Name of the practitioner who performed the procedure Charges for the procedure The Procedure class’s _ _init_ _ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator…arrow_forward1) Set numberofseats to 40 and use Seat(id:int) constructor to create 40 seats with differentids. Id should be encapsulated but only getter method should be obtained. User will notbe able to change the id number, will be just able to see it.2) At the initilization stage, all seats will have null Passenger and false isReserved values.These values should be obtained in case of creation of new reservation to the seat.3) Name, surname, phonenumber, mail values in Passenger class should be encapsulatedand getter and setter methods should be obtained.4) User will be able to make reservation to any seat but when user tries to make reservation,isReserved value will be searched and if the seat is already reserved then and errormessage should be shown to the user. Otherwise passenger information will be requiredfrom the user and passenger object will be created and assigned to the seat andisReserved value will be true.5) When a reservation of cancel of reservation has happened,…arrow_forward
- Create a Student class, where attributes associated with each student are name, registration number, father name, degree and department. All attributes should not be accessed directly. One can view the details of all students. Note student attributes can not be changed by any means after initialization.In student class maintaining student count means your program could be able to tell the count of student objects created. (User static counter variable)arrow_forwardHow do I add an object reference to an instance of an object. It seems that is the major issue here and not the code itself:arrow_forwardDefine the Circle2DYourLastName class that contains: Two double data fields names x and y that specify the center of the circle withgetter methods. A double data field radius with getter method. A constructor that creates a circle with specified x, y, and radius. A method findTypeOfIntersection to determine and return types ofintersection as string (contained, overlap, or separate).Design a user class that prompts the user to enter the coordinates of the center andradius of two circles and displays the type of intersection. Allow the user to repeat yourprogram using a ConfirmDialog Box.arrow_forward
- Create a code the follow way: Each student object should also contain thenumber of earned credits. Provide a constructor that sets this value based on parameter value. Overload theconstructor such that the number of earned credits is initially zero. Provide setter and getter for this newfield. Modify the toString method such that the new information is included in the description of thestudent. Modify the driver class main method to exercise the new Student methods.arrow_forwardCreate one more method in the Starship class: encounter(self, enemy_ship) This method takes in self, along with another Starship object. The method should simulate a battle between the two ships. How the method is implemented is almost entirely up to you. You could take in user input for each crew member, or have them each act randomly from the available options, or specify exactly what a given crew member of each type should do. The only requirements are as follows: Each crew member on each ship should get at least one “turn” before the battle is complete. If the crew member is Injured on their turn, they should do nothing. You may assume that each ship will have at least one crew member uninjured when the encounter begins. You shouldn’t make any assumptions about how many crew members there will be on a given ship, where they will start, or about which subclasses will be present - it’s possible that you’ll have test cases including a ship with no captain, or no doctor.…arrow_forwardTHE BELOW IS IMPORTANT Rewrite the above code so that It has all of the correct access modifiers for good encapsulation. Your code obeys the principle of encapsulation that obliges you to put each method in the proper class. Your code uses NO getters and NO additional classes or methods. You are welcome to modify any existing methods (including signatures and bodies) and/or move them between the existing classes. Your code DOES NOT modify any of the constructors. Important notes: You DO NOT have to write a main method. You DO NOT have to write tests. You DO NOT have to write Javadocs. You DO NOT have to write interfaces to encapsulate data structures. There should be no incorrect syntax or other compile-time errors.arrow_forward
- Modified Circle class and test file: Add a new attribute for color (or any other new attribute) Add get/set methods for this new instance variable, including data validation in set method (assuming background is black, i.e. circle color can't be black, reset to any non-black color if black is entered ). Add a new constructor to pass the color attribute as an argument. Add an instance method to display all properties of a Circle object. Create 2 objects using different constructors in test file, with invalid data. Use this test java file test java file: class CircleTest{ public static void main(String[] args){ int num = 10; Circle c1 = new Circle(0.001); Circle c2 = new Circle("black", -9); c1.display(); c2.display(); c1.setRadius( -1); c1.setColor("black"); c1.display(); }}arrow_forwardThe class "Car" has the following attributes: plate (String), mark (String), model (String), year (int), km (int).Write a constructor method for the "Car" class that takes values as parameters for all these attributes.arrow_forwardWrite a shoe class with the following attributes: color (e.g., "blue", "green", "orange") displayName (e.g., "nikes, adidas", "puma") price (e.g., 100, 200,60) Include only one constructor. It should have parameters for each of the attributes and set their values. Additionally, include getters and setters for each of the attributes. Add a driver, name it Purchases, and create 2 objects. Finally, print out some information about both objects (i.e., print the information from some or all of the getters). For example, if you created a shoe object whose color was blue, whose display name was shoes , for a price of 230, you could use the getters to print something like this:These work trousers are blue and cost $230arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
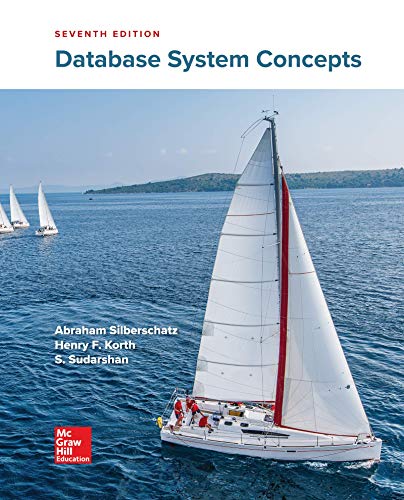
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
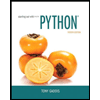
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
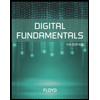
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
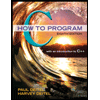
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
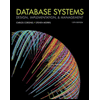
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
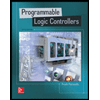
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY