The Cat and Dog class below implement the Animal interface. All animals have names, but only dogs do tricks. Implement the describe method, using the instanceof operator to test whether the given animal is a dog. If so, return the name, a space, and the trick that the dog can do, such as "Helmut barks at the moon". If it is any other kind of animal, return the name, a space, and "has no tricks". public class Animals { /** Describes the given animal. @param pet an animal @return a string with the animal's name, a space, and either the trick that the animal knows (if it is a dog) or a string "has no tricks" */ public static String describe(Animal pet) { // TODO: Complete this method } // This method is used to check your work public static String check(String name, String trick) { Animal pet; if (trick == null) pet = new Cat(name); else pet = new Dog(name, trick); return describe(pet); }
The Cat and Dog class below implement the Animal interface. All animals have names, but only dogs do tricks. Implement the describe method, using the instanceof operator to test whether the given animal is a dog. If so, return the name, a space, and the trick that the dog can do, such as "Helmut barks at the moon". If it is any other kind of animal, return the name, a space, and "has no tricks".
public class Animals
{
/**
Describes the given animal.
@param pet an animal
@return a string with the animal's name, a space, and
either the trick that the animal knows (if it is a dog) or a
string "has no tricks"
*/
public static String describe(Animal pet)
{
// TODO: Complete this method
}
// This method is used to check your work
public static String check(String name, String trick)
{
Animal pet;
if (trick == null) pet = new Cat(name);
else pet = new Dog(name, trick);
return describe(pet);
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

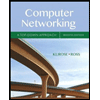
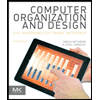
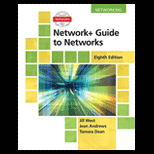
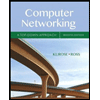
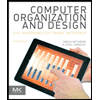
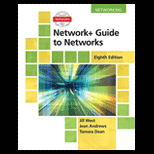
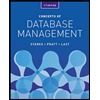
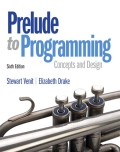
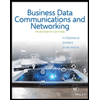