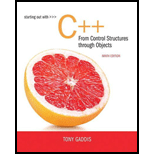
Explanation of Solution
Appending a node:
It is the process of adding a new node at the end of a linked list.
Member function for appending a node:
The following member function is used to append the received value into a list at end:
//Definition of appendNode() function
void IntList::appendNode(int num)
{
//Declare a structure pointer variables
ListNode *newNode, *dataPtr = nullptr;
//Assign a new node
newNode = new ListNode;
//Store the argument value into "newNode"
newNode->value = num;
//Assign the next pointer to be "NULL"
newNode->next = nullptr;
//Condition to check whether the list is empty or not
if (!head)
//If true, make newNode as the first node
head = newNode;
//else part
else
{
//Store head value into dataPtr
dataPtr = head;
//Loop to find the last node in the list
while (dataPtr->next)
//Assign last node to the structure variable
dataPtr = dataPtr->next;
//Insert newNode as the last node
dataPtr->next = newNode;
}
}
Explanation:
Consider the function named “appendNode()” to insert the received value “num” at end of the list.
- Make a new node and assign a received value “num” into the node.
- Using “if…else” condition check whether the list is empty or not.
- If the “head” node is empty, assign a new node into “head” pointer.
- Otherwise, make a loop to find a last node in the list and insert the value at end of the list.
Inserting a node:
It is the process of adding a new node at a specific location in a list.
Member function for inserting a node:
The following member function is used to insert the received value into a list at specific location:
//Definition of insertNode() function
void IntList::insertNode(int num)
{
/*Declare a structure pointer variables*/
ListNode *newNode, *dataPtr, *prev = nullptr;
//Assign a new node
newNode = new ListNode;
//Store the argument value into "newNode"
newNode->value ...

Want to see the full answer?
Check out a sample textbook solution
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
- What is the best way to code?arrow_forwardC# Programming What is the wrong of this code? Can you fix the error on this part? See attached photo for referencearrow_forwardCreate C# (Sharp) code using visual studio: Use Delegate methodology to do the following project: Have a Add method, Subtract method, Multiply method, Divide method. Each method will loop 100 times. With each iteration, generate two random numbers (between 1 - 50), then perform the math operation (add for the Add method, subtract for the Subtract method, etc.). Make sure to display the numbers and the answer! One validation check - for subtraction and division, make sure the higher number generated comes first in the calculation. For example, if 12 and 40 are the numbers generated, the math should be 40 -12 for the subtraction (not 12 - 40) and 40/12 for the division (not 12/40). Again, make sure to use Delegates!arrow_forward
- What is displayed as a result of executing the code segment?arrow_forwardBig O notation format: Determine the runtime of the given code below.arrow_forwardIn PYTHON Print values from the Goal column only Print values only for columns: Team, Yellow Cards and Red Cards Find and print rows for teams that scored more than 6 goals Select the teams whose name start with a G Print values only for columns Team and Shooting Accuracy , and only for rows in which the team is either of the following: England, Italy and Spain Find and print rows for teams that scored more than 6 goals and at least 4000 passes Team Goals Shots on target Shots off target Shooting Accuracy Goals-to-shots (perc) Total shots (inc. Blocked) Hit Woodwork Penalty goals Penalties not scored Headed goals Passes Passes completed Passing Accuracy Touches Crosses Dribbles Corners Taken Tackles Clearances Interceptions Clearances off line Clean Sheets Blocks Goals conceded Saves made Saves-to-shots ratio Fouls Won Fouls Conceded Offsides Yellow Cards Red Cards Subs on Subs off Players Used Croatia 4 13 12 0.5 0.16 32 0 0 0 2 1076 828 0.769 1706 60…arrow_forward
- Explain the code and write comment on each code line.arrow_forwardWhy do programmers insert blank lines and indentations in their code?arrow_forwardPython:Temperature Statistics Learning Objectives In this lab, you will Create a function to match the specifications Use the if/else statements to detect a range Use lists to store the results Instructions Sahara desert explorers call us for help! They want to know some statistics about the temperature in Sahara, but sometimes their thermometer fails to record the proper temperature. Help them to find which temperature is from correct recordings and which is broken and calculate statistics on given data. During the night, the temperature in Sahara varies from -4 to -10 С. During the day, the temperature varies from 20 to 50 C. Create a function check_input(temperature) that will return True if the temperature given as input can be from Sahara and False if it is from a broken thermometer (i.e., outside of the expected range). Steps Write a program to use the check_input to create a list of valid temperatures and compute their statistics: Create a list, where you will store the…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage