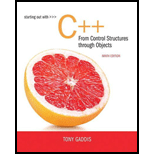
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 13PC
Program Plan Intro
Rainfall Statistics Modification #2
Program Plan:
“IntList.h”:
- Include the required specifications into the program.
- Define a class template named “IntList”.
- Declare the member variables “value” and “*next” in structure named “ListNode”.
- Declare the constructor, copy constructor, destructor, and member functions in the class.
- Declare a class template and define a function named “appendNode()” to insert the node at end of the list.
- Declare the structure pointer variables “newNode” and “dataPtr” for the structure named “ListNode”.
- Assign the value “num” to the variable “newNode” and assign null to the variable “newNode”.
- Using “if…else” condition check whether the list to be empty or not, if the “head” is empty and make a new node into “head” pointer. Otherwise, make a loop find last node in the loop.
- Assign the value of “dataPtr” into the variable “newNode”.
- Declare a class template and define a function named “print()” to print the values in the list.
- Declare the structure pointer “dataPtr” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Make a loop “while” to display the values of the list.
- Declare a class template and define a function named “insertNode()” used to insert a value into the list.
- Declare the structure pointer variables “newNode”, “dataPtr”, and “prev” for the structure named “ListNode”.
- Make a “newNode” value into the received variable value “num”.
- Using “if…else” condition to check whether the list is empty or not.
- If the list is empty then initialize “head” pointer with the value of “newNode” variable.
- Otherwise, make a “while” loop to test whether the “num” value is less than the list values or not.
- Use “if…else” condition to initialize the value into list.
- Declare a class template and define a function named “deleteNode()”to delete a value from the list.
- Declare the pointer variables “dataPtr”, and “prev” for the structure named “ListNode”.
- Using “if…else” condition to check whether the “head” value is equal to “num” or not.
- Initialize the variable “dataPtr” with the value of the variable “head”.
- Remove the value using “delete” operator and reassign the “head” value into the “dataPtr”.
- If the “num” value not equal to the “head” value, then define the “while” loop to assign the “dataPtr” into “prev”.
- Use “if” condition to delete the “prev” pointer.
- Declare a class template and define a function named “getTotal()” to calculate total value in a list.
- Define a variable named “total” and initialize it to “0” in type of template.
- Define a pointer variable “nodePtr” for the structure “ListNode” and initialize it to be “NULL”.
- Assign the value of “head” pointer into “nodePtr”.
- Define a “while” loop to calculate “total” value of the list.
- Return a value of “total” to the called function.
- Declare a class template and define a function named “numNodes()” to find the number of values that are presented in the list.
- Declare a variable named “count” in type of “integer”.
- Define a pointer variable “nodePtr” and initialize it to be “NULL”.
- Assign a pointer variable “head” to the “nodePtr”.
- Define a “while” loop to traverse and count the number of elements in the list.
- Declare a class template and define a function named “getAverage()”to find an average value of elements that are presented in list.
- Declare a class template and define a function named “getLargest()”to find largest element in the list.
- Declare a template variable “largest” and pointer variable “nodePtr” for the structure.
- Using “if” condition, assign the value of “head” into “largest” variable.
- Using “while” loop, traverse the list until list will be empty.
- Using “if” condition, check whether the value of “nodePtr” is greater than the value of “largest” or not.
- Assign address of “nodePtr” into “nodePtr”.
- Return a value of “largest” variable to the called function.
- Declare a class template and define a function named “getSmallest()” to find smallest element in the list.
- Declare a template variable “smallest” and pointer variable “nodePtr” for the structure.
- Using “if” condition, assign the value of “head” into “smallest” variable.
- Using “while” loop, traverse the list until list will be empty.
- Using “if” condition, check the value of “nodePtr” is smaller than the value of “smallest”.
- Assign address of “nodePtr” into “nodePtr”.
- Return a value of “smallest” variable to the called function.
- Declare a class template and define a function named “getSmallestPosition()” to find the position of smallest value in the list.
- Declare a template variable “smallest” and pointer variable “nodePtr” for the structure.
- Using “while” loop traverses the list until the list will be empty.
- Using “if” condition, find the position of “smallest” value in the list.
- Return the value of “position” to the called function.
- Declare a class template and define a function named “getLargestPosition()” to find the position of largest value in the list.
- Declare a template variable “largest” and pointer variable “nodePtr” for the structure.
- Using “while” loop traverses the list until the list will be empty.
- Using “if” condition, find the position of “largest” value in the list.
- Return the value of “position” to the called function.
- Define the destructor to destroy the values in the list.
- Declare the structure pointer variables “dataPtr”, and “nextNode” for the structure named “ListNode”.
- Initialize the “head” value into the “dataPtr”.
- Define a “while” loop to make the links of node into “nextNode” and remove the node using “delete” operator.
- Declare a class template and define a function named “storeToFile()” to save the list values into file.
- Declare a pointer variable “nodePtr” for a structure “ListNode” and assign it’s to be null.
- Make a call to create a file object “outFile()” and initialize the value of variable “head” pointer into “nodePtr”.
- Using “while” loop save the values of list into file and assign the next node into “nodePtr”.
- Close the file using “close()” method.
- Declare a class template and define a function named “getFromFile()” to get the data from file into list.
- Declare a pointer variable “nodePtr” for a structure “ListNode” and assign it’s to be null.
- Declare a template variable “newValue” and create an object for file.
- Using “while” loop, get the values from file into list.
- Close the file using “close()” method.
Program #1 “Main.cpp”:
- Include the required header files into the program.
- Declare a variable “n” in type of integer.
- Read the value of “n” from user and using “while” loop to validate the data entered by user.
- Declare an object named “rainfall” for the class “IntList”.
- Using “for” loop, read an input for every month from user.
- Append the value entered from user into the list.
- Make a call to the function “storeToFile()” to write the list values into file.
Program #2 “Main.cpp”:
- Include the required header files into the program.
- Declare an object named “rainfall” for the class “IntList”.
- Make a call to the function “getFromFile()” to read a file into list using “rainfall” object.
- Make a call to “getTotal()”, “getAverage()”, “getLargest()”, “getSmallest()”, “getLargestPosition()”, and “getSmallestPosition()” function and display the values on the screen.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Program Specification
For this assignment you will write a program to help people record the events of their day by supplying prompts and then saving their responses along with the question and the date to a file.
Functional Requirements
This program must contain the following features:
Write a new entry - Show the user a random prompt (from a list that you create), and save their response, the prompt, and the date as an Entry.
Display the journal - Iterate through all entries in the journal and display them to the screen.
Save the journal to a file - Prompt the user for a filename and then save the current journal (the complete list of entries) to that file location.
Load the journal from a file - Prompt the user for a filename and then load the journal (a complete list of entries) from that file. This should replace any entries currently stored the journal.
Provide a menu that allows the user choose these options
Your list of prompts must contain at least five different prompts.…
Music Player – Songs in music player are linked to previous and next song. You can play songs from either starting or ending of the list. Write an algorithm for the above problem and analyse the efficiency of the algorithm.
Lottery number generator: Write a program that generates aseven-digit lottery number. The program should have a loopto generate seven random numbers, each in the range 0through 9 and assign each number to a list element.2. Write another loop to display the contents of the list.3. Tip: You will need to create/initialize your list before you canassign numbers to it.4. Use program 7-1 sales_list as an example. You will start witha seven-digit lottery number that contains all zeros. Then inyour loop, you will assign a random number instead ofgetting the data from the user.Turn in your program to the practice assignment link in coursecontent.
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - Prob. 18.2CPCh. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.2 - Prob. 18.5CPCh. 18.2 - Prob. 18.6CPCh. 18.2 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - Prob. 3RQECh. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - Prob. 6RQECh. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - T F The programmer must know in advance how many...Ch. 18 - T F It is not necessary for each node in a linked...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 33RQECh. 18 - Prob. 34RQECh. 18 - Prob. 35RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - List Template Create a list class template based...Ch. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C# Gas Station Program: Write a program that allows the users to select items that are for sale in a gas station. Candy, Soda, Pizza, Muffins and the list goes on. Keep a running total of the items ordered (loop until user is done ordering) when they are done ordering items, output the total salessales.arrow_forwardC++ You should create a loop which will ask user if they want to insert, delete, display and exit. Then call the corresponding method based on the user's input. The loop will only stop once user entered "Exit" Topic: LinkedListarrow_forwardIf you have downloaded the source code from this book’s companion Web site, you will find a file named USPopulation.txt in the Chapter 07 folder. The file contains the midyear population of the United States, in thousands, during the years 1950 through 1990. The first line in the file contains the population for 1950, the second line contains the population for 1951, and so forth.Write a program that reads the file’s contents into a list. The program should display the following data:• The average annual change in population during the time period• The year with the greatest increase in population during the time period• The year with the smallest increase in population during the time period(You can access the book’s companion Web site at www.pearsonglobaleditions.com/gaddis.)arrow_forward
- Python write a program in python that plays the game of Hangman. When the user plays Hangman, the computer first selects a secret word at random from a list built into the program. The program then prints out a row of dashes asks the user to guess a letter. If the user guesses a letter that is in the word, the word is redisplayed with all instances of that letter shown in the correct positions, along with any letters correctly guessed on previous turns. If the letter does not appear in the word, the user is charged with an incorrect guess. The user keeps guessing letters until either: * the user has correctly guessed all the letters in the word or * the user has made eight incorrect guesses. one for each letter in the secret word and Hangman comes from the fact that incorrect guesses are recorded by drawing an evolving picture of the user being hanged at a scaffold. For each incorrect guess, a new part of a stick-figure body the head, then the body, then each arm, each leg, and finally…arrow_forwardTrue/False 7. Items can be removed from a list with the del operatorarrow_forwardTrue or False When you create a List object, you do not have to know the number of items that you intend to store in it.arrow_forward
- phyton Topics: list and list processing You will write a program to read grade data from a text file, displays the grades as a 5-column table, and then print the statistics (min, max, median, and median). You can assume that the input file only one number per line. You can assume the user always enter a file that exists. The median is the value in the middle of a sorted list. To sort a list, use list.sort() function. It’s computed as below. For a list of odd length, the middle number is just the length divide by 2. For the list, [1,2,3], the median is 2 since 2 is in the middle of the list. The middle index is 3//2, which is 1. Remember that // is the integer division operator. When the length of the list is even, there are two middle numbers. The median is the average of the two middle numbers. For example, [1,2,3,4], the median is (2+3)/2 = 2.5. The two middle indexes for this example are (4//2)-1 = 1 and (4//2) = 2. Functions you need to write: read_grades() Prompts…arrow_forwardThis term refers to an individual item in a list.a. elementb. binc. cubbyholed. slotarrow_forwardLab Goal : This lab was designed to teach you more about list processing and algorithms.Lab Description : Write a program that will search through a list to find the smallest number and the largest number. The program will return the average the largest and smallest numbers. You must combine variables, ifs, and a loop to create a working method. There will always be at least one item in the list.arrow_forward
- A list called parts has already been created and filled with data. It has a length of 5. Write a for loop that prints each value in this list.arrow_forward2. ID: A Name: 2. A list of numbers is considered increasing if each value after the first is greater than or equal to the preceding value. The following procedure is intended to return true if numberList is increasing and return false otherwise. Assume that numberList contains at least two elements. Line 1: PROCEDURE isIncreasing (numberList) Line 2: { Line 3: count 2 Line 4: REPEAT UNTIL(count > LENGTH(numberList)) Line 5: Line 6: IF(numberList[count] =. YA198ICarrow_forwardThe program will take in a year as an input from the user and output the popular dance and popular slang from that decade. Years, slang, and dances are stored in parallel lists in the starter code. The decade 1920 is located at index 0 and accompanies the 1920’s slang and dance also located at index 0, the decade 1930 is located at index 1 and accompanies the 1930’s slang and dance also located at index 1, etc. year_list = list(range(1920, 2030, 10)) slang_list = ["bee's knees", "hip", "cool", "nifty", "groovy", "far out", "gnarly", "phat", "sweet", "lit", "fire"] dance_list = ["The Charleston", "The Jitterbug", "The Lindy Hop", "The Hand Jive", "The LocoMotion", "The Electric Slide", "The Moonwalk", "The Macarena", "Single Ladies", "Juju on that Beat", "The Renegade"] year = int(input("Year: ")) decade_index = (year - 1920) // 10 if decade_index == 11: print(f"In the {year_list[decade_index]}'s, The {dance_list[decade_index]} is the hip dance craze!") else:…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
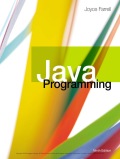
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT