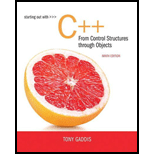
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 32RQE
Program Plan Intro
Linked list:
Linked list is a linear and dynamic data structure which is used to organize data; it contains sequence of elements which are connected together in memory to form a chain. The every element of linked list is called as a node.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
True or False The objects of a class can be stored in an array, but not in a List.
LABEL: 120
The class MyList has three dynamic member variables.
Given the code segment below, which function will be invaked at line 5, assuming that the Big Three were implemented?
The type of element in the list is irrelevant.
1 Mylist nums;
2 nums.append(10);
3 nums. append(20);
4 nums. append( 30);
5 MyList result;
O The copy constructor
The overloaded assignment operator
O The default assignment operator
The default constructor
The destructor
Scores Class
Write a class named Scores which should have the following attribute:
• _scores (a list to hold test scores, 0 - 100. Initially empty. )
The Scores class should have an _init__ method that creates the _scores attribute. It should also have the following methods:
• add_score: this method adds a score to the __scores list. It accepts a single score as it's' only argument.
• get_average: this method accepts no arguments. If _scores is empty, this method returns -1. Otherwise, it returns the average of all scores in _scores.
• get_high_score: this method accepts no arguments. if scores is empty, this method returns -1. Otherwise, it returns the highest score in __scores.
• get_low_score: this method accepts no arguments. if scores is empty, this method returns -1. Otherwise, it returns the lowest score in scores.
• _str_: This method returns a string similar to the following (assuming the _scores contains 89, 77, 93):
Once you have written the class, write a program that…
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - Prob. 18.2CPCh. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.2 - Prob. 18.5CPCh. 18.2 - Prob. 18.6CPCh. 18.2 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - Prob. 3RQECh. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - Prob. 6RQECh. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - T F The programmer must know in advance how many...Ch. 18 - T F It is not necessary for each node in a linked...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 33RQECh. 18 - Prob. 34RQECh. 18 - Prob. 35RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - List Template Create a list class template based...Ch. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- urgent helparrow_forward#ifndef H_StackType#define H_StackType#include <iostream>#include <cassert>using namespace std;template <class Type>struct nodeType{ Type info; nodeType<Type> *link;};template <class Type>class linkedStackType: public stackADT<Type>class linkedStackType{public: const linkedStackType<Type>& operator= (const linkedStackType<Type>&); bool isEmptyStack() const; bool isFullStack() const; void initializeStack(); //Function to initialize the stack to an empty state. void push(const Type& newItem); Type top() const; //Function to return the top element of the stack. //Precondition: The stack exists and is not empty. //Postcondition: If the stack is empty, the program // terminates; otherwise, the top element of // the stack is returned. void pop(); linkedStackType(); //Postcondition: stackTop = NULL; linkedStackType(const…arrow_forwardFill in the blanksarrow_forward
- TRUE OR FALSE A method that uses a generic class parameter can be static or dynamic.arrow_forwardData structure & Algorithum java program 1) Add a constructor to the class "AList" that creates a list from a given array of objects.2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject)3) Write a program that thoroughly tests all the methods in the class "AList".4) Include comments to all source code.arrow_forwardStatic vs. non-static class data members: What real-world circumstance might benefit from a static data member?arrow_forward
- Circular Queue: A circular queue is the extended version of a regular queue where the last element is connected to the first element. Thus forming a circle-like structure. Create a C++ generic abstract class named as CircularQueue with the following: Attributes: Type*arr; int front; int rear; int maxSize; Functions: virtual void enqueue(Type) = 0; Adds the element of type Type at the end of the circular queue. virtual Type dequeue() = 0; Deletes the first most element of the circular queue and returns it. PLEASE USE C++arrow_forwarda) Create a TreeNode class with the following methods: default constructor, two overloaded constructors, copy constructor, getValue, getLeft, getRight, setValue, setLeft, setRight b) Create a BTInterface with the following abstract methods: getRoot, setRoot, isEmpty, swapSubtrees, singleParent, preorder, postOrder, inOrder, insert c) Create an abstract Binary Tree class that implements the interface. Include the default constructor, a private helper method called checkNode for singleParent() and toString. Make insert an abstract method. d) Derive a Binary Search Tree class from Binary Tree with the following methods: default constructor, overloaded constructor (use a variable length parameter), insert, e) Create a TreeDemo class that creates two BinarySearch Tree objects. Use default and overloaded constructors. For tree one: call the methods makeTree (makes a complete tree), print Tree, swap Subtrees, print Tree. Simliar for tree two. Also, print out the number of single parents in…arrow_forwardclass Polynomial: class TermNode:def __init__(self, coefficient: int, exponent: int) -> None:"""Initialize this node to represent a polynomial term with thegiven coefficient and exponent. Raise ValueError if the coefficent is 0 or if the exponentis negative."""if coefficient == 0:raise ValueError("TermNode: zero coefficient")if exponent < 0:raise ValueError("TermNode: negative exponent") self.coeff = coefficientself.exp = exponentself.next = None def __init__(self, coefficient: int = None, exponent: int = 0) -> None:"""Initialize this Polynomial with a single term constructed from thecoefficient and exponent. If one argument is given, the term is a constant coefficient(the exponent is 0).If no arguments are given, the Polynomial has no terms. # Polynomial with no terms:>>> p = Polynomial()>>> print(p._head)None>>> print(p._tail)None # Polynomial with one term (a constant):>>> p = Polynomial(12)>>> p._head.coeff12>>>…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
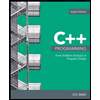
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning