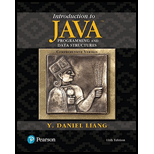
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13.6, Problem 13.6.1CP
Program Plan Intro
Interface:
Interface is a reference type in Java, which contains a collection of abstract methods.
An interface needs to the follow certain conditions such as:
- One cannot instantiate an interface.
- Interfaces do not contain any constructors.
- All methods present in the interface are abstracts.
- An interface does not contain any instance field.
- An interface cannot be extended by a class.
Comparable Interface:
- A comparable interface is a generic interface that defines the compareTo() method that is used for comparing objects.
- Using comparable interface, one can choose the properties of the objects, rather than the reference location of the objects.
Example:
//java langauage package for comaprable interface
package java.lang
//comparable interface definition
public interface Comparable <E>
{
//use of compareTo to compare objects
public integer compareTo(E o);
}
Explanation:
The compareTo() method determines the order of this object with the specified object “o” and returns a negative integer, zero or positive integer, if this object is less than, equal to or greater than object “o”.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Define a class method (static) named compare to the Fraction class. The compare method
accepts two Fraction objects f1 and f2. The method returns
-1 if f1 is less than f2
O if f1 is equal to f2
+1 if f1 is greater than f2
In this assignment, you are implementing a class from a model shown here.
PlayingCard
suit char
value int
+ PlayingCard (s:char, v:int):
+ getSuit(): char
+ getValue(): int
+ setSuit (s:char):void
+ setValue(v:int):void
+ toString(): String
+isMatch (p:PlayingCard): boolean
PlayingCard ADT
1) The constructor takes 2 char values and
initializes the value and suit of the PlayingCard.
2) Accessor functions for the suit and value (getters)
3) Mutator functions for the suit and value (setters)
4) A toString method that displays the PlayingCard
in the format (value, suit). For example, a 10 of
hearts would display as (10,H). Face cards should
display K, Q, J, or A, for the values 13, 12, 11, 14,
respectively.
5) An isMatch method that takes a PlayingCard p as an
argument and returns true if p's suit or value match
that of the calling object
After writing the class, test each function in a main method before proceeding to the next
steps.
Once your class is fully tested, write a driver method…
What can be said about a class that implements the Comparable interface but does not override the compareTo method?
Select one:
O The class is invalid.
O The class must be abstract.
O Objects of the class are not allowed to call compareTo.
None of these options
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 13.2 - Prob. 13.2.1CPCh. 13.2 - The getArea() and getPerimeter() methods may be...Ch. 13.2 - True or false? a.An abstract class can be used...Ch. 13.3 - Prob. 13.3.1CPCh. 13.3 - Prob. 13.3.2CPCh. 13.3 - Prob. 13.3.3CPCh. 13.3 - What is wrong in the following code? (Note the...Ch. 13.3 - What is wrong in the following code? public class...Ch. 13.4 - Can you create a Calendar object using the...Ch. 13.4 - Prob. 13.4.2CP
Ch. 13.4 - How do you create a Calendar object for the...Ch. 13.4 - For a Calendar object c, how do you get its year,...Ch. 13.5 - Prob. 13.5.1CPCh. 13.5 - Prob. 13.5.2CPCh. 13.5 - Prob. 13.5.3CPCh. 13.5 - Prob. 13.5.4CPCh. 13.6 - Prob. 13.6.1CPCh. 13.6 - Prob. 13.6.2CPCh. 13.6 - Can the following code be compiled? Why? Integer...Ch. 13.6 - Prob. 13.6.4CPCh. 13.6 - What is wrong in the following code? public class...Ch. 13.6 - Prob. 13.6.6CPCh. 13.6 - Listing 13.5 has an error. If you add list.add...Ch. 13.7 - Can a class invoke the super.clone() when...Ch. 13.7 - Prob. 13.7.2CPCh. 13.7 - Show the output of the following code:...Ch. 13.7 - Prob. 13.7.4CPCh. 13.7 - What is wrong in the following code? public class...Ch. 13.7 - Show the output of the following code: public...Ch. 13.8 - Prob. 13.8.1CPCh. 13.8 - Prob. 13.8.2CPCh. 13.8 - Prob. 13.8.3CPCh. 13.9 - Show the output of the following code: Rational r1...Ch. 13.9 - Prob. 13.9.2CPCh. 13.9 - Prob. 13.9.3CPCh. 13.9 - Simplify the code in lines 8285 in Listing 13.13...Ch. 13.9 - Prob. 13.9.5CPCh. 13.9 - The preceding question shows a bug in the toString...Ch. 13.10 - Describe class-design guidelines.Ch. 13 - (Triangle class) Design a new Triangle class that...Ch. 13 - (Shuffle ArrayList) Write the following method...Ch. 13 - (Sort ArrayList) Write the following method that...Ch. 13 - (Display calendars) Rewrite the PrintCalendar...Ch. 13 - (Enable GeometricObject comparable) Modify the...Ch. 13 - Prob. 13.6PECh. 13 - (The Colorable interface) Design an interface...Ch. 13 - (Revise the MyStack class) Rewrite the MyStack...Ch. 13 - Prob. 13.9PECh. 13 - Prob. 13.10PECh. 13 - (The Octagon class) Write a class named Octagon...Ch. 13 - Prob. 13.12PECh. 13 - Prob. 13.13PECh. 13 - (Demonstrate the benefits of encapsulation)...Ch. 13 - Prob. 13.15PECh. 13 - (Math: The Complex class) A complex number is a...Ch. 13 - (Use the Rational class) Write a program that...Ch. 13 - (Convert decimals to fractious) Write a program...Ch. 13 - (Algebra: solve quadratic equations) Rewrite...Ch. 13 - (Algebra: vertex form equations) The equation of a...
Knowledge Booster
Similar questions
- Modified Circle class and test file: Add a new attribute for color (or any other new attribute) Add get/set methods for this new instance variable, including data validation in set method (assuming background is black, i.e. circle color can't be black, reset to any non-black color if black is entered ). Add a new constructor to pass the color attribute as an argument. Add an instance method to display all properties of a Circle object. Create 2 objects using different constructors in test file, with invalid data. Use this test java file test java file: class CircleTest{ public static void main(String[] args){ int num = 10; Circle c1 = new Circle(0.001); Circle c2 = new Circle("black", -9); c1.display(); c2.display(); c1.setRadius( -1); c1.setColor("black"); c1.display(); }}arrow_forwardSally and Harry implement two different compareTo methods for a classthey are working on together. Sally’s compareTo method returns −1, 0, or +1,depending on the relationship between two objects. Harry’s compareTo methodreturns −6, 0, or +3. Which method is suitable?arrow_forwardGiven a Student class, and UndergradCourse is a true subtype of Course, there is one method called recommend in the Student class: public class Student { public Course recommend(Course pCourseID); } Please evaluate if EACH of the method in UndergradStudent class violates the Liskov Substitution Principle. public class UndergradStudent extends Student { 1. public Course recommend(UndergradCourse pCourseID); 2. public UndergradCourse recommend(Course pCourseID); 3. public UndergradCourse recommend(Object pCourseID); 4. public Course recommend(Course pCourseID) throw SomeCheckedException;arrow_forward
- Class Library implements the interface Findable and has/should have the following members: - Instance variable Map books (maps the name of the book to the total number of pages in the book). - A constructor to initialize the instance variable. -Override method find (T obj) to search for books with total pages more than or equal to that of obj. Class Test has the main method. main () method accepts inputs to instantiate an object of Library. For each book, the input is accepted in the order-book name followed by the number of pages in the book.arrow_forwardtri1 and tri2 are instances of the Triangle class. Attributes height and base of both tri1 and tri2 are read from input. In the Triangle class, define instance method print_info() with self as the parameter to output the following in one line: 'Height: ' The value of attribute height ', base: ' The value of attribute basearrow_forwardDynamic Apex: Write test class for this apex code(Not required the output) You are working on the project in which you have to create the apex code in which your task is to define the objects dynamically by using the method argument which is api name in string and a map of string, string as a key value pair. Class Code: public static void modifySobjectData(String str , Map mapofString) { //Retrive the type of Sobject by using the getGlobalDescribe Schema.SobjectType targetType = Schema.getGlobalDescribe().get(str); //Initalize the Object Sobject newobject = targetType.newSobject(); for (String s :mapofString.keySet() { newobject.put(s, mapofString.get(s)); } System.debug('newObject' + newobject); //Perform the DML if(targetType != null) insert newobject; } }arrow_forward
- Define the Student class that has two attributes: name and gpa Implement the following instance methods: A constructor that sets name to "Defualt name"(What ever you want to give) and gpa to 1.0 set_name(self, name) - set student's name to parameter name get_name(self) - return student's name set_gpa(self, gpa) - set student's gpa to parameter gpa get_gpa(self) - return student's gpa Ex. If a new Student object is created, the default output is: Default name/1.0 Ex. If the student's name is set to "Frank" and the gpa is set to 3.7, the output becomes: Frank/3.7arrow_forwardDynamic Apex: Write test class for this apex code(Not required the output) You are working on the project in which you have to create the apex code in which your task is to define the objects dynamically by using the method argument which is api name in string and a map of string, string as a key value pair. Class Code: public static void modifySobjectData(String str , Map mapofString) { //Retrive the type of Sobject by using the getGlobalDescribe Schema.SobjectType targetType = Schema.getGlobalDescribe().get(str); //Initalize the Object Sobject newobject = targetType.newSobject(); for(String s :mapofString.keySet(O) { newobject.put(s, mapofString.get(s)); } System.debug('newObject' + newobject); //Perform the DML if(targetType != null) insert newobject; } }arrow_forwardpublic class date { private int day; // from 1 to 31 private int month; // from 1 to 12 private int year; // from 2000 upwards public void advance (); // move to next day }; Implement a constructor that initializes new objects of date class to be set to the 1st of January 2000. Implement setters for day, month, and year. Implement the advance method, which moves to the next day, ensuring that all data members are updated appropriately.arrow_forward
- Point out errors in the following codes and correct them publicclass CheckingAccount { // Create attributes account Num, Balance privateint accountNum; // Account Number privatedouble balance; // Account balance // Constructor public void CheckingAccount(int aNum, double balance) { // Call setters to initialize attribute values setAccountNumber (aNum); setBalance; } // Setters public void setAccountNumber(int aNum) { if (accountNum >= 100) { accountNum = aNum; } else {System.out.println("This account number is invalid (Account number must be at least 3 digits)!"); this.accountNum = 100; // assign a default value } } public void setBalance(double aBalance) { // make sure the value is positive if (aBalance > 0) { aBalance = balance; } else { System.out.println("This balance value is invalid (input must be positive)!"); aBalance = 0; // assign a default value } } // Getters publicvoid getAccountNumber() { return accountNum; } public void getBalance() { return balance; }…arrow_forwardUsing explicit constructor can avoid type conversions. Group of answer choices True Falsearrow_forwardIn Python, #Create a basket class, which has a class/static attribute pricelist (string/float dictionary - you can hardcode this) #The constructor should set the name to the name passed as a parameter and initialise the contents (empty) #You should provide an addItem method taking product,quantity which adds this to the contents #and a getQuantity method taking a product, which returns the relevant quantityarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
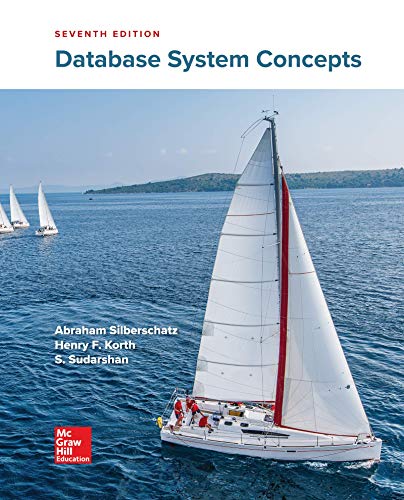
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
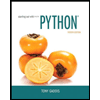
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
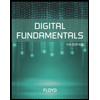
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
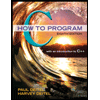
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
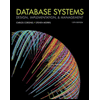
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
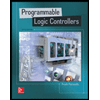
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education