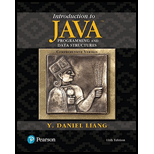
a)
“abstract” class:
An abstract class is a class which may or may not include abstract methods and cannot be instantiated but rather it can be sub classed.
Legal “abstract” class:
- The conditions for a class to be considered as legal abstract class are mentioned below:
- If a class includes abstract methods, then the class must be declared as abstract.
- The abstract method that is declared within the abstract class must be declared without braces and followed by a semicolon.
Example of a Legal “abstract” class:
The example of a legal abstract class is mentioned below:
public abstract class GraphicRectangle {
//declare fields
//declare methods
abstract void drawRectangle();
}
Given code:
//class definition
class A {
//declaration of an abstract method
abstract void unfinished() {
}
//end of class
}
b)
“abstract” class:
An abstract class is a class which may or may not include abstract methods and cannot be instantiated but rather can be sub classed.
Legal “abstract” class:
The conditions for a class to be considered as legal abstract class are mentioned below:
- If a class includes abstract methods, then the class must be declared as abstract.
- The abstract method that is declared within the abstract class must be declared without braces and followed by a semicolon.
Example of a Legal “abstract” class:
The example of a legal abstract class is mentioned below:
public abstract class GraphicRectangle {
//declare fields
//declare methods
abstract void drawRectangle();
}
Given code:
//class definition
public class abstract A {
//declaration of an abstract method
abstract void unfinished();
//end of class
}
c)
“abstract” class:
An abstract class is a class which may or may not include abstract methods and cannot be instantiated but rather can be sub classed.
Legal “abstract” class:
The conditions for a class to be considered as legal abstract class are mentioned below:
- If a class includes abstract methods, then the class must be declared as abstract.
- The abstract method that is declared within the abstract class must be declared without braces and followed by a semicolon.
Example of a Legal “abstract” class:
The example of a legal abstract class is mentioned below:
public abstract class GraphicRectangle {
//declare fields
//declare methods
abstract void drawRectangle();
}
Given code:
//class definition
class A {
//declaration of an abstract method
abstract void unfinished();
//end of class
}
d)
“abstract” class:
An abstract class is a class which may or may not include abstract methods and cannot be instantiated but rather can be sub classed.
Legal “abstract” class:
The conditions for a class to be considered as legal abstract class are mentioned below:
- If a class includes abstract methods, then the class must be declared as abstract.
- The abstract method that is declared within the abstract class must be declared without braces and followed by a semicolon.
Example of a Legal “abstract” class:
The example of a legal abstract class is mentioned below:
public abstract class GraphicRectangle {
//declare fields
//declare methods
abstract void drawRectangle();
}
Given code:
//class definition
abstract class A {
//declaration of a protected method
protected void unfinished();
//end of class
}
e)
“abstract” class:
An abstract class is a class which may or may not include abstract methods and cannot be instantiated but rather can be sub classed.
Legal “abstract” class:
The conditions for a class to be considered as legal abstract class are mentioned below:
- If a class includes abstract methods, then the class must be declared as abstract.
- The abstract method that is declared within the abstract class must be declared without braces and followed by a semicolon.
Example of a Legal “abstract” class:
The example of a legal abstract class is mentioned below:
public abstract class GraphicRectangle {
//declare fields
//declare methods
abstract void drawRectangle();
}
Given code:
//class definition
abstract class A {
//declaration of an abstract method
abstract void unfinished();
//end of class
}
f)
“abstract” class:
An abstract class is a class which may or may not include abstract methods and cannot be instantiated but rather can be sub classed.
Legal “abstract” class:
The conditions for a class to be considered as legal abstract class are mentioned below:
- If a class includes abstract methods, then the class must be declared as abstract.
- The abstract method that is declared within the abstract class must be declared without braces and followed by a semicolon.
Example of a Legal “abstract” class:
The example of a legal abstract class is mentioned below:
public abstract class GraphicRectangle {
//declare fields
//declare methods
abstract void drawRectangle();
}
Given code:
//class definition
abstract class A {
//declaration of an abstract method
abstract int unfinished();
//end of class
}

Trending nowThis is a popular solution!

Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- If a member is declared private in a class, can it be accessed from other classes? If a member is declared protected in a class, can it be accessed from other classes? If a member is declared public in a class, can it be accessed from other classes?arrow_forwardTo overload the pre-increment operator for a class as a member function, how many arguments are required? b.When overloading the pre-increment operator for a class as a friend function, how many arguments are required?arrow_forwardWhich of the following is true of abstract classes? a.An abstract class cannot have child classes b.An abstract class is a parent class with more than one child class. c.An abstract class cannot be instantiated. d.An abstract class is another name for a base class. Which of the following is true of abstract methods? Select one: a.An abstract method is a method of the child class which overrides a parent method. b.An abstract method is a method without a body which is declared using the keyword abstract. c.An abstract method is any method of an abstract class d.An abstract method is a method which cannot be inherited. Can an abstract class define both abstract and non abstract methods. a.No. All methods must be one or the other. b.No. All methods must be abstract. c.Yes, but the child classes do not inherit the abstract methods. d.Yes. The child classes inherit both.arrow_forward
- Please read the main function and define a class CStudent. (object array, static member, string) Please do not change the main function and complete the program given. 【Description】 Please read the main function and define a class CStudent. In the main funciton, two arrays of the ojbect of CStudent are define. Please analyze the call of constructor and destructor in the process of creating and releasing objects. 1. CStudent has two private member variables:stirng name, int age. 2. CStudent has a static member count. It will be incremented by 1 when the object is created and decremented by 1 when the object is destroyed. 3. The constructor function should output "*** is contructing". The destructor function should output “*** is destructing”. "***" indicates the name of the current CStudent object. 【Input】 No input 【Output sample】 There are 0 students. noname is contructing noname is contructing noname is contructing noname is contructing noname is contructing name:noname…arrow_forwarda. How many parameters are required to overload the pre-increment operator for a class as a member function? b. How many parameters are required to overload the post-increment operator for a class as a friend function?arrow_forwardWrite a class declaration for a class named Circle, which has the data member radius, a double, and member functions setRadius and getArea. Write the code for these as inline functions.arrow_forward
- Can a derived class directly access by name a private instance variable ofthe base class?arrow_forwarda. How many parameters are required to overload the pre-increment operator for a class as a member function? b. How many parameters are required to overload the pre-increment operator for a class as a friend function?arrow_forwardWhich statement of the following is the most appropriate? Group of answer choices When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the invariant of the class, and should be written at the botttom of the implementation file for easy reference. When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the variant of the class, and should be written at the botttom of the implementation file for easy reference. When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the invariant of the class, and should be written at the top of the implementation file for easy reference. When you design a class, always make an explicit statement of the rules that dictate how the member variables are used. These rules are called the…arrow_forward
- People in School Objective: At the end of the activity, the students should be able to: Create a program that exhibits inheritance. Procedure: Write a simple information system that will store and display the complete information of a student, faculty, or employee. Create four (4) no-modifier classes named Person, Student, Faculty, and Employee. Create a public class named CollegeList. This class shall contain the main method. Refer to the UML Class Diagram for the names of the variables and methods. The (-) symbol represents private variables, while (+) represents public method. This should be the sequence of the program upon execution: Prompt the user to select among Employee, Faculty, or Student, by pressing E, F, or S. Ask the user to type the name and contact For Employee, ask the user to type the employee's monthly salary and the department where he/she belongs to (Ex. Registrar). Then, display name, contact number, salary, and For Faculty, ask the user to press Y if…arrow_forwardComplete the following table by filling in private, protected, public, or inaccessible in the right-hand column: In a private base class, this base class MEMBER access specification... ..becomes this access specification in the derived class. private protected publicarrow_forwardHow do you define a friend function to access a class’s private members?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
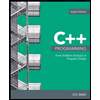