Point out errors in the following codes and correct them publicclass CheckingAccount { // Create attributes account Num, Balance privateint accountNum; // Account Number privatedouble balance; // Account balance // Constructor public void CheckingAccount(int aNum, double balance) { // Call setters to initialize attribute values setAccountNumber (aNum); setBalance; } // Setters public void setAccountNumber(int aNum) { if (accountNum >= 100) { accountNum = aNum; } else {System.out.println("This account number is invalid (Account number must be at least 3 digits)!"); this.accountNum = 100; // assign a default value } } public void setBalance(double aBalance) { // make sure the value is positive if (aBalance > 0) { aBalance = balance; } else { System.out.println("This balance value is invalid (input must be positive)!"); aBalance = 0; // assign a default value } } // Getters publicvoid getAccountNumber() { return accountNum; } public void getBalance() { return balance; } /*================================= * Create a method for depositing* Parameter to indicate the amount to be to be deposited (must be* positive)====================================*/ public void deposit(double dAmount) { double balance = 0; // make sure the value is positive if (dAmount > 0) { balance += dAmount; } else { // The balance does not change System.out.println("This deposit value is invalid (input must be positive)!"); } } /*====================================* Create a method for withdrawing* Parameter to indicate the amount to be withdrawn* Must be positive and never more than the balance( i.e. no overdrawing) ====================================*/ public void withdraw(double wAmount) { // make sure the value is positive and less than the balance if (wAmount > 0) { // if (this.balance >= wAmount) { this.balance = wAmount; } else { System.out.println("Withdraw exceeds balance, operation cancelled!"); } } else{ //negative value System.out.println("This withdraw value is invalid, withdrawmust be positive!"); } } } //end of class definition
Point out errors in the following codes and correct them publicclass CheckingAccount { // Create attributes account Num, Balance privateint accountNum; // Account Number privatedouble balance; // Account balance // Constructor public void CheckingAccount(int aNum, double balance) { // Call setters to initialize attribute values setAccountNumber (aNum); setBalance; } // Setters public void setAccountNumber(int aNum) { if (accountNum >= 100) { accountNum = aNum; } else {System.out.println("This account number is invalid (Account number must be at least 3 digits)!"); this.accountNum = 100; // assign a default value } } public void setBalance(double aBalance) { // make sure the value is positive if (aBalance > 0) { aBalance = balance; } else { System.out.println("This balance value is invalid (input must be positive)!"); aBalance = 0; // assign a default value } } // Getters publicvoid getAccountNumber() { return accountNum; } public void getBalance() { return balance; } /*================================= * Create a method for depositing* Parameter to indicate the amount to be to be deposited (must be* positive)====================================*/ public void deposit(double dAmount) { double balance = 0; // make sure the value is positive if (dAmount > 0) { balance += dAmount; } else { // The balance does not change System.out.println("This deposit value is invalid (input must be positive)!"); } } /*====================================* Create a method for withdrawing* Parameter to indicate the amount to be withdrawn* Must be positive and never more than the balance( i.e. no overdrawing) ====================================*/ public void withdraw(double wAmount) { // make sure the value is positive and less than the balance if (wAmount > 0) { // if (this.balance >= wAmount) { this.balance = wAmount; } else { System.out.println("Withdraw exceeds balance, operation cancelled!"); } } else{ //negative value System.out.println("This withdraw value is invalid, withdrawmust be positive!"); } } } //end of class definition
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Point out errors in the following codes and correct them
publicclass CheckingAccount {
// Create attributes account
Num, Balance
privateint accountNum; // Account Number
privatedouble balance; // Account balance
// Constructor
public void CheckingAccount(int aNum, double balance) {
// Call setters to initialize attribute values
setAccountNumber (aNum);
setBalance;
}
// Setters
public void setAccountNumber(int aNum) {
if (accountNum >= 100) {
accountNum = aNum;
}
else
{System.out.println("This account number is invalid (Account number must be at least 3 digits)!");
this.accountNum = 100;
// assign a default value
}
}
public void setBalance(double aBalance) {
// make sure the value is positive
if (aBalance > 0) {
aBalance = balance;
}
else {
System.out.println("This balance value is invalid (input must be positive)!");
aBalance = 0;
// assign a default value
}
}
// Getters
publicvoid getAccountNumber() {
return accountNum;
}
public void getBalance() {
return balance;
}
/*================================= * Create a method for depositing* Parameter to indicate the amount to be to be deposited (must be* positive)====================================*/
public void deposit(double dAmount) {
double balance = 0;
// make sure the value is positive
if (dAmount > 0) {
balance += dAmount;
}
else {
// The balance does not change
System.out.println("This deposit value is invalid (input must be positive)!");
}
}
/*====================================* Create a method for withdrawing* Parameter to indicate the amount to be withdrawn* Must be positive and never more than the balance( i.e. no overdrawing) ====================================*/
public void withdraw(double wAmount) {
// make sure the value is positive and less than the balance
if (wAmount > 0) { //
if (this.balance >= wAmount) {
this.balance = wAmount;
}
else {
System.out.println("Withdraw exceeds balance, operation cancelled!");
}
}
else{
//negative value
System.out.println("This withdraw value is invalid, withdrawmust be positive!");
}
}
}
//end of class definition
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
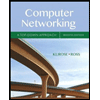
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
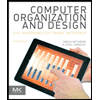
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
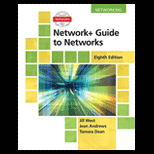
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
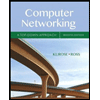
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
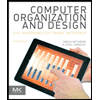
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
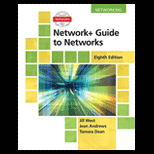
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
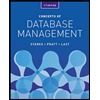
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
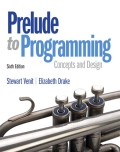
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
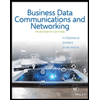
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY