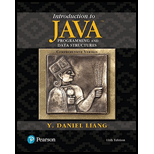
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 13.6, Problem 13.6.3CP
Can the following code be compiled? Why?
Integer n1 = new Integer(3);
Object n2 = new Integer(4);
System.out.println(n1.compareTo(n2));
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
a) FindMinIterative
public int FindMin(int[] arr) { int x = arr[0]; for(int i = 1; i < arr.Length; i++) { if(arr[i]< x) x = arr[i];
} return x; }
b) FindMinRecursive
public int FindMin(int[] arr, int length) { if(length == 1) return arr[0];
return Math.Min(arr[length - 1], Find(arr, length - 1));
}
What is the Big-O for this functions. Could you explain the recurisive more in details ?
// MichiganCities.java - This program prints a message for invalid cities in Michigan.
// Input: Interactive.
// Output: Error message or nothing.
import java.util.Scanner;
public class MichiganCities
{
public static void main(String args[]) throws Exception
{
// Declare variables.
String inCity; // name of city to look up in array.
// Initialized array of cities in Michigan.
String citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"};
boolean foundIt = false; // Flag variable.
int x; // Loop control variable.
// Get user input.
Scanner input = new Scanner(System.in);
System.out.println("Enter the name of the city: ");
inCity = input.nextLine();
// Write your loop here.
for (x=0;x<= citiesInMichigan.length;x++){
if(citiesInMichigan[x]==inCity){
foundIt = true;…
Basic Java Help
Each year, the Social Security Administration provides a list of baby names for boy and girls by state. You will write a program to read a file containing a list of baby names and display the top names.
To start, implement the following class: baby names util = printTopNames(File, int): void
printTopNames
Will be a static method. It will accept two parameters, one is a File containing the list of names. The other is an integer that indicates how many names to display (ie, top 10 names, or top 50 names, etc). The integer argument could be any valid integer.
You do not have to worry about an integer value large than 5 for the boy/girl names.
This method should open the file and parse the file and print the names as shown below:
Your program will use the Scanner class to read the data from the file (see your text for examples). You will also need to use a delimiter with the scanner so that the scanner will break the line apart based on the commas. For this file, set…
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 13.2 - Prob. 13.2.1CPCh. 13.2 - The getArea() and getPerimeter() methods may be...Ch. 13.2 - True or false? a.An abstract class can be used...Ch. 13.3 - Prob. 13.3.1CPCh. 13.3 - Prob. 13.3.2CPCh. 13.3 - Prob. 13.3.3CPCh. 13.3 - What is wrong in the following code? (Note the...Ch. 13.3 - What is wrong in the following code? public class...Ch. 13.4 - Can you create a Calendar object using the...Ch. 13.4 - Prob. 13.4.2CP
Ch. 13.4 - How do you create a Calendar object for the...Ch. 13.4 - For a Calendar object c, how do you get its year,...Ch. 13.5 - Prob. 13.5.1CPCh. 13.5 - Prob. 13.5.2CPCh. 13.5 - Prob. 13.5.3CPCh. 13.5 - Prob. 13.5.4CPCh. 13.6 - Prob. 13.6.1CPCh. 13.6 - Prob. 13.6.2CPCh. 13.6 - Can the following code be compiled? Why? Integer...Ch. 13.6 - Prob. 13.6.4CPCh. 13.6 - What is wrong in the following code? public class...Ch. 13.6 - Prob. 13.6.6CPCh. 13.6 - Listing 13.5 has an error. If you add list.add...Ch. 13.7 - Can a class invoke the super.clone() when...Ch. 13.7 - Prob. 13.7.2CPCh. 13.7 - Show the output of the following code:...Ch. 13.7 - Prob. 13.7.4CPCh. 13.7 - What is wrong in the following code? public class...Ch. 13.7 - Show the output of the following code: public...Ch. 13.8 - Prob. 13.8.1CPCh. 13.8 - Prob. 13.8.2CPCh. 13.8 - Prob. 13.8.3CPCh. 13.9 - Show the output of the following code: Rational r1...Ch. 13.9 - Prob. 13.9.2CPCh. 13.9 - Prob. 13.9.3CPCh. 13.9 - Simplify the code in lines 8285 in Listing 13.13...Ch. 13.9 - Prob. 13.9.5CPCh. 13.9 - The preceding question shows a bug in the toString...Ch. 13.10 - Describe class-design guidelines.Ch. 13 - (Triangle class) Design a new Triangle class that...Ch. 13 - (Shuffle ArrayList) Write the following method...Ch. 13 - (Sort ArrayList) Write the following method that...Ch. 13 - (Display calendars) Rewrite the PrintCalendar...Ch. 13 - (Enable GeometricObject comparable) Modify the...Ch. 13 - Prob. 13.6PECh. 13 - (The Colorable interface) Design an interface...Ch. 13 - (Revise the MyStack class) Rewrite the MyStack...Ch. 13 - Prob. 13.9PECh. 13 - Prob. 13.10PECh. 13 - (The Octagon class) Write a class named Octagon...Ch. 13 - Prob. 13.12PECh. 13 - Prob. 13.13PECh. 13 - (Demonstrate the benefits of encapsulation)...Ch. 13 - Prob. 13.15PECh. 13 - (Math: The Complex class) A complex number is a...Ch. 13 - (Use the Rational class) Write a program that...Ch. 13 - (Convert decimals to fractious) Write a program...Ch. 13 - (Algebra: solve quadratic equations) Rewrite...Ch. 13 - (Algebra: vertex form equations) The equation of a...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Ingredient Adjuster A cookie recipe calls for the following ingredients: 1.5 cups of sugar 1 cup of butter 2...
Starting Out with Python (4th Edition)
The ____________ is always transparent.
Web Development and Design Foundations with HTML5 (8th Edition)
T F A variable must be defined before it can be used.
Starting Out with C++ from Control Structures to Objects (9th Edition)
What is the difference between the names defined in an ML let construct from the variables declared in a C bloc...
Concepts Of Programming Languages
Complete and fully test the class Characteristic that Exercise 5 describes. Include the following methods: getD...
Java: An Introduction to Problem Solving and Programming (8th Edition)
What is an object?
Starting Out With Visual Basic (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- How do I code: public static int maxValue(int[] arr) public static int maxValue(int[] arr, int firstIndex, int lastIndex) public static int indexOfFirstMaxValue(int[] arr) in java?arrow_forwardWhat does the following code do? In [1]: def mystery (a, b) : if b == 1: return a else: return a + mystery (a, b - 1) In [2]: mystery (2, 10) Out [2]: ?????arrow_forward6arrow_forward
- How do I change the code so if numMinutes is zero then the number cast members is also zero? Code: import java.util.*; import java.util.Arrays; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; // default constructor public Movie() { this.movieName = "Flick"; this.numMinutes = 0; this.isKidFriendly = false; this.numCastMembers = 0; this.castMembers = new String[10]; } // overloaded parameterized constructor public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = new String[numCastMembers]; for (int i = 0; i < castMembers.length; i++) { this.castMembers[i] = castMembers[i]; } } // set the number of minutes public void setNumMinutes(int numMinutes) { this.numMinutes = numMinutes; } //…arrow_forwardWhen an array is passed as a parameter to a method, modifying the elements of the array from inside the function will result in a change to those array elements as seen after the method call is complete. O True O Falsearrow_forwardUse the following code without changing anything to to produce the output that is attached. public class GradeBookTest { public static void main( String[] args ) { // two-dimensional array of student grades int[][] gradesArray = { { 87, 96, 70 }, { 68, 87, 90 }, { 94, 100, 90 }, { 100, 81, 82 }, { 83, 65, 85 }, { 78, 87, 65 }, { 85, 75, 83 }, { 91, 94, 100 }, { 76, 72, 84 }, { 87, 93, 73 } }; processGrades(gradesArray);rowAverage(row)lowestGrade(gradesArray);maxGrade(gradesArray); } // end main } // end class GradeBookTestarrow_forward
- for the class ArrayAverage write a code that calculates the average of the listed numbers with a double result for the class ArrayAverageTester write a for each loop and print out the resultarrow_forwardMovie. Java Codearrow_forward3. Factorials/MathUtils File Factorials.java contains a program that calls the factorial method of the MathUtils class to compute the factorials of integers entered by the user. Save these files to your directory and study the code in both, then compile and run Factorials to see how it works. Try several positive integers, then try a negative number. You should find that it works for small positive integers (values < 17), but that it returns a large negative value for larger integers and that it always returns 1 for negative integers.Returning 1 as the factorial of any negative integer is not correct—mathematically, the factorial function is not defined for negative integers. To correct this, you could modify your factorial method to check if the argument is negative, but then what? The method must return a value, and even if it prints an error message, whatever value is returned could be misconstrued. Instead it should throw an exception indicating that something went wrong so it…arrow_forward
- 7. Compute set of number. Waiting for codearrow_forwardANSWER IN JAVA: Write an application that inputs five numbers, each between 10 and 100, inclusive. As each number is read, display it only if it’s not a duplicate of a number already read. Provide for the “worst case,” in which all five numbers are different. Use the smallest possible array to solve this problem. Display the complete set of unique values input after the user enters each new value. NOTE: looking for the output to appear on three lines as shown in the results STANDARD OUTPUT: Enter·an·integer·between·10·and·100:This·is·the·first·time·100·has·been·entered↵ Enter·an·integer·between·10·and·100:Enter·an·integer·between·10·and·100:This·is·the·first·time·10·has·been·entered↵ Enter·an·integer·between·10·and·100:This·is·the·first·time·20·has·been·entered↵ Enter·an·integer·between·10·and·100:The·complete·set·of·unique·values·entered·is:↵ Unique·Value·1:·is·100↵ Unique·Value·2:·is·10↵ Unique·Value·3:·is·20↵arrow_forwarddef getDimension(): row = int(input("Enter number of rows (between 4 and 10): ")) while row < 4 or row > 10: row = int(input("Invalid input! Enter number of rows (between 4 and 10): ")) column = int(input("Enter number of columns (between 4 and 10): ")) while column < 4 or column > 10: column = int(input("Invalid input! Enter number of columns (between 4 and 10): ")) return row, column def printBoard(board): for row in board: print("|", end="") for cell in row: if cell == "": print(" ", end="") else: print(cell, end="") print("|", end="") print() print("-" * (len(board[0]) * 2 + 1)) def dropToken(board, col, token): row = len(board) - 1 while row >= 0: if board[row][col] == "": board[row][col] = token return row row -= 1 return -1 def isValidLocation(board, col): return board[0][col] == "" def isTie(board):…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
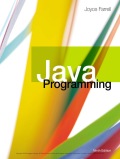
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
9.1: What is an Array? - Processing Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=NptnmWvkbTw;License: Standard Youtube License