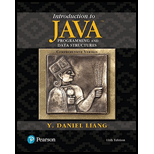
Interface:
Interface is a reference type in Java, which contains a collection of abstract methods.
An interface needs to the follow certain conditions such as:
- One cannot instantiate an interface.
- Interfaces do not contain any constructors.
- All methods present in the interface are abstracts.
- An interface does not contain any instance field.
- An interface cannot be extended by a class.
Comparable Interface:
A comparable interface is a generic interface that defines the compareTo() method that is used for comparing objects.
Example:
//java langauage package for comaprable interface
package java.lang
//comparable interface definition
public interface Comparable <E>
{
//use of compareTo to compare objects
public integer compareTo(E o);
}
The compareTo() method determines the order of this object with the specified object “o” and returns a negative integer, zero or positive integer, if this object is less than, equal to or greater than object “o”.

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- Explore scenarios where you might need to implement a custom Comparator for a class that already implements the Comparable interface. Provide real-world examples for context.arrow_forward1. Why do you need to implement all the methods of an interface in class which implements an interface?arrow_forwardAnswer the following questions based on knowledge of inner class: Please answer according to question please please.arrow_forward
- If you're only implementing an interface, why do you need to implement all its methods?arrow_forwardImplement the following parking permit class using java It has a dependency on the car class, no need to implement the car class. Use the diagram for refrencearrow_forwardDescribe how you can use an interface as an alternative to a class to specify the type for a parameter.arrow_forward
- Create a deck of cards class. Internally, the deck of cards should use another class, a card class. Your requirements are: The Deck class should have a deal method to deal a single card from the deck After a card is dealt, it is removed from the deck. There should be a shuffle method which makes sure the deck of cards has all 52 cards and then rearranges them randomly. The Card class should have a suit (Hearts, Diamonds, Clubs, Spades) and a value (A,2,3,4,5,6,7,8,9,10,J,Q,K)arrow_forwardT/F1. Interface classes cannot be extended but classes that implement interfaces can be extended.arrow_forwardIdentify the steps need to be taken in the correct orderarrow_forward
- Why is it important for a class to have a destructor implemented? With your comment, fill in the spaces.arrow_forwardExplain the advantages of passing this data to a method rather than the current class object directly.arrow_forwardThe class "Car" has the following attributes: plate (String), mark (String), model (String), year (int), km (int).Write a constructor method for the "Car" class that takes values as parameters for all these attributes.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
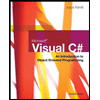