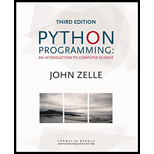
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 9PE
Program Plan Intro
Sphere
Program Plan:
Sphere.py
- Import the required packages.
- Definition of main method
- Get the radius of the sphere
- Call the method “Sphere()” by passing the parameter “rad”.
- Call the method “surfaceArea()”.
- Call the method “volume()”.
- Print the volume of the sphere
- Print the area of the sphere.
- Call the main function
Main.py:
- Import the required packages
- Definition of class sphere
- Definition of method “init()”.
- Assign the value of radius.
- Definition of method “getRadius()”.
- Return the value of radius.
- Definition of method “surfaceArea()”.
- Calculate surface area
- Definition of method “volume()”.
- Calculate the volume.
- Return the volume.
- Definition of method “init()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Create a rectangle class that can compute the area and the sum of its sides. Create a specialized method that will allow the class to compare other rectangle objects. The comparison should identify the larger rectangle according to the object’s area. You should be able to implement the class where the user provides rectangle dimensions. The program should be able to display which of the rectangle inputs is larger using the Rectangle class method that you defined.
Sample output:
Enter Length R1: 1
Enter Width R1: 1
Enter Length R2: 2
Enter Width R2: 2
Results:
R1 Area: 1
R1 Sum: 2
R2 Area: 4
R2 Sum: 4
R2 is the bigger Rectangle!
Note: All characters in boldface are user inputs.
Design an application that does the following: A Solid can be a FlatSurfaceSolid or a CurvedSurfaceSolid. Every solid will have a volume. The method volume will be polymorphic. A solid has faces, vertices and edges. A flat-surface solid also has a variable called side which represents the length of the side. A curved-surface solid also has a radius. A Cube is a FlatSurfaceSolid. A cube has 6 faces, 8 vertices and 12 edges. It will have a side. A Sphere is a CurvedSurfaceSolid. It has 1 face, 0 vertices and 0 edges. It will have a radius. Create an ArrayList of solids (driver file named YourLastNameCISC231Q1.java). The arraylist will have 4 elements – 2 cubes (one with side length 3 and another will side length 4) and 2 spheres (one with radius 3 and another with radius 4). Create a driver class to instantiate an array of solids and display the details of each solid along with the volume of each solid.
Implement the critter class.
Each critter C has attributes species, size, and age.
• The constructor accepts arguments for the attributes above, in the order above.
You can expect size and age to be numeric values.
Each critter C has a can_eat () method, which:
Receives one argument, prey, which you can expect to be another Critter object.
Compares prey 's size against C's.
o If C is larger than prey, return True. Otherwise, return False.
Each critter C also has a survive_year () method, which:
• Increases C's size and age by 1, respectively, and returns C's current size.
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 10 - Prob. 1TFCh. 10 - Prob. 2TFCh. 10 - Prob. 3TFCh. 10 - Prob. 4TFCh. 10 - Prob. 5TFCh. 10 - Prob. 6TFCh. 10 - Prob. 7TFCh. 10 - Prob. 8TFCh. 10 - Prob. 9TFCh. 10 - Prob. 10TF
Ch. 10 - Prob. 1MCCh. 10 - Prob. 2MCCh. 10 - Prob. 3MCCh. 10 - Prob. 4MCCh. 10 - Prob. 5MCCh. 10 - Prob. 6MCCh. 10 - Prob. 7MCCh. 10 - Prob. 8MCCh. 10 - Prob. 9MCCh. 10 - Prob. 10MCCh. 10 - Prob. 1DCh. 10 - Prob. 3DCh. 10 - Prob. 1PECh. 10 - Prob. 2PECh. 10 - Prob. 3PECh. 10 - Prob. 4PECh. 10 - Prob. 5PECh. 10 - Prob. 6PECh. 10 - Prob. 7PECh. 10 - Prob. 8PECh. 10 - Prob. 9PECh. 10 - Prob. 10PECh. 10 - Prob. 11PECh. 10 - Prob. 13PECh. 10 - Prob. 15PECh. 10 - Prob. 16PE
Knowledge Booster
Similar questions
- Implement the inventory manager class. This class must have methods that do the following: Add a new item to the inventory manager. Remove an item from the inventory manager. Re-stock an item in the inventory manager. Display the items in the inventory manager. Search for an item in the inventory manager by at least two criteria (name, price, quantity, etc.). Part 3: Test the inventory manager class with a driver program or unit tests. This may be a console application.arrow_forwardIn this lab you will write three classes: Die, PairOfDice, and Player.The Die class mimics the rolling of a die. Specifically, this class will implement the following methods:• A default constructor initializing the number of sides of a die to 6.• An overloaded constructor that takes an integer number of sides (assume greater than 1).• roll which generates and returns a random number between 1 and the number of sides(inclusive).• An accessor method to read the value of the face on the die.• A toString method returning the string representation of the face value.The maximum number of sides should be stored as a private constant in the Die class. Also use theRandom class for the random number generator.The PairOfDice class mimics the rolling of two dice. Specifically, this class will implement thefollowing methods:• A default constructor that creates and initializes the number of sides of each die to 6.• An overloaded constructor that creates and takes two integer number of sides, one…arrow_forwardLoad your bag with some initial values, but do not fill it. Your client code should give the user the option to: display the contents of the bag using the class method “toVector” add values to the bag remove values from the bag sort the bag search for a value using their choice of either the iterative search or the recursive search - both need to be tested Allow the user to keep doing these things until they are done. Do not automatically sort the bag if the user chooses to search. Your program should give the user a message telling them they must first sort the bag before they can search.You decide what the user interface will be. Make it clear and easy to use. It can be very basic; nothing fancy. A menu driven program works well. Each value in your array should be unique - no value will appear in the array multiple times. Your array elements can be any data type – use a template class put in place in the code provided. You can write your client code to process a bag holding any…arrow_forward
- JAVA HELP PLEASE! Write a class Rectangle that has only the following public methods (you can add other non-public methods if you think you need them): Write a constructor that creates a rectangle using the x, y coordinates of its lower left corner, its width and its height (the parameters must be in that order). Creating a rectangle with non-positive width or height should not be allowed; throw an IllegalArgumentException for those cases. The values of x and y are allowed to be negative. Write a method overlap(Rectangle other). This method should return true if this rectangle overlaps with other, false otherwise. Rectangles that touch each other are not considered to be overlapping. Write a method toString that returns a String. The string should be formatted exactly as:“x:2, y:3, w:4, h:5”without the quotation marks and replacing the numbers with the actual attribute values of the object. A class called Rectangle exists in Java already. You are not allowed to use this class in any…arrow_forwardJava - Access Specifiers Create a class named Circle that has attributes radius, area, and circumference and make the attributes private. Make a public method that sets the radius and a method that prints all attributes. Ask the user input for radius. Note: Use the PI from the math functions Inputs A line containing an integer 10 Sample Output Radius: 20 Area: 1256.64 Circumference: 125.66arrow_forwardDirectionsThe total cost of a group of items at a grocery store is based on the sum of the individual product prices and the tax (which is 5.75%). Products that are considered “necessities” are not taxed, whereas products that are considered “luxuries” are. For this practice problem you will need to download Shopping Trip Starting Code.zip The Product class is abstract, and it has a method called getTotalPrice. Your task is to create two subclasses of Product: NecessaryProduct and LuxuryProduct and implement the getTotalPrice method in each of these classes appropriately.Then modify the driver program to instantiate four products (two necessary and two luxury) and store them in the product array, print out each item in the array, and display the total cost of the items. You should not make any change at all to Product.java, and you should only add to ShoppingTripStartingCode.java. Do not change any code that is already present. Make sure that it is for anygiven…arrow_forward
- Write a class for the following object. Fraction - an object that represents parts of a whole number. A fraction is created by supplying a numerator and a denominator. Instance related actions 1. add - mutates this fraction by adding the other fraction value 2. subtract - mutates this fraction by subtracting the other fraction value 3. multiply - mutates this fraction by multiplying with the other fraction value 4. divide - mutates this fraction by dividing by the other fraction value 5. tostring - represents the fraction in a form of 'numerator/denominator" 6. compareTo - returns the following values 1. O when this fraction is equal with the other fraction 2. 1 when this fraction is greater than the other fraction 3. -1 when this fraction is less than the other fraction Example fraction1 = 1/2 fraction2 = 3/4 When fraction1.add(fraction2] is called, it will make the value of fraction 1 as 5/4. When fraction1.subtract(fraction2) is called, it will make the value of fraction 1 as -1/4.…arrow_forwardIn java language Write a Rectangle class. A Rectangle has properties of width and length. You construct a Rectangle object by providing the width and length in that order. If no width and length are provided to the constructor, construct a Rectangle with width 0.0 and length 0.0. We want to be able to get and set both the width and the length independently. We also want to be able to ask for the area of the rectangle and the perimeter of the rectangle. What will the object need to remember? width and length - those are the instance variables. Rectangle class has these constructors : public Rectangle() - Constructs a new rectangle with width and length of 0.0. public Rectangle(double width, double length) - Constructs a new rectangle with the given width and length. Remember that the job of the constructor is to initialize the instance variables. It has these methods. public double getWidth() - Gets the width of this Rectangle public double getLength() - Gets the length of this…arrow_forwardIn java language Write a Rectangle class. A Rectangle has properties of width and length. You construct a Rectangle object by providing the width and length in that order. If no width and length are provided to the constructor, construct a Rectangle with width 0.0 and length 0.0. We want to be able to get and set both the width and the length independently. We also want to be able to ask for the area of the rectangle and the perimeter of the rectangle. What will the object need to remember? width and length - those are the instance variables. Rectangle class has these constructors : public Rectangle() - Constructs a new rectangle with width and length of 0.0. public Rectangle(double width, double length) - Constructs a new rectangle with the given width and length. Remember that the job of the constructor is to initialize the instance variables. It has these methods. public double getWidth() - Gets the width of this Rectangle public double getLength() - Gets the length of this…arrow_forward
- Create a class Rectangle with parameters length, breadth, and color. Use appropriate data types andaccess specifiers. Write overloaded constructors to create objects of rectangle. First constructor takesall the three parameters. Second constructer takes two parameters length and breadth. If only oneparameter is given then user creates square of red color. Write methods to set and get the values oflength, breadth and color. Write methods to find length of the diagonal of rectangle and to display thecomplete details of rectangle with area. Create another class TestRectangle which uses allconstructors to create the different objects, uses appropriate set and get methods wherever required.Display the details of every rectangle. (IN JAVA LANGUAGE)arrow_forwardWrite a method toString() for the Cow class which displays information about a Cow object. The method should consider the following: • If the Cow's stomach value is 100, the output should say that the Cow is full • If the Cow's stomach value is below 100 but above 50, no information about the Cow's stomach should be given • If the Cow's stomach value is below 50 but above 10, the output should mention that the cow is hungry • If the Cow's stomach value is below 10, the output should mention that the cow is starving. Example output: First Cow is full. Second Cow Third Cow is hungry. Fourth Cow is starving!arrow_forwardCreate a Book class with attributes such as book title, author, and price. Implement a parameterized constructor to initialize these attributes. Now, extend the functionality of the Bookstore class by adding a new method called 'addBook.' This method should allow the addition of custom books to the bookstore's inventory. The method should take three parameters: title, author, and price. Implement this method to ensure that the bookstore's inventory is updated accordingly. Instantiate a new object of the bookstore, naming it 'ReadersHaven, utilizing the extended class with the newly added 'addBook' method. Your next step is to add three distinct custom books to 'ReadersHaven-for example, 'The Silent Observer® by Jane Doe, priced at $20.99, Adventures in Wonderland" by Lewis Carroll, priced at $15.50, and 'Programming Mastery by John Coder, priced at $30.75. To conclude, utilize a 'displayinventory' method to showcase the updated information about 'ReadersHaven." Verify that the output…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
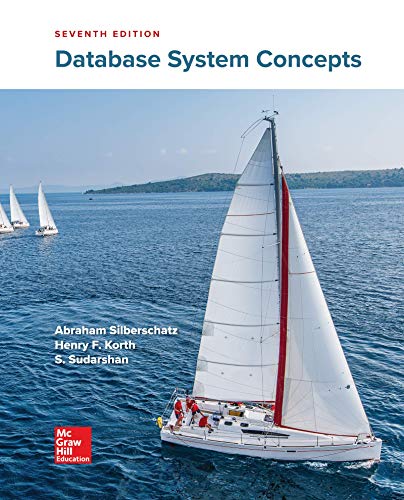
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
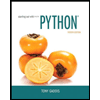
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
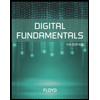
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
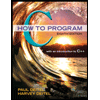
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
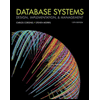
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
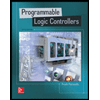
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education