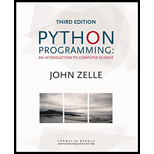
Three Button Monte
Program Plan:
- Import the required packages.
- Declare a main function. Inside the main function,
- Create the application window.
- Draw the interface widget by creating three buttons.
- Assign the text to the interface.
- Call the method “draw()”.
- Get the random number.
- Assign the values to “false”.
- Check the condition.
- Assign “point1” to “true”.
- Check the condition.
- Assign the “point2” to “true”.
- Otherwise, Assign the “point3” to “true”
- Activate all the three doors.
- Get the action mouse clicked.
- Check the condition using “while” loop.
- Check the condition for selecting the door 1 to be clicked.
- Check the condition for selecting the door 2 to be clicked.
- Check the condition for selecting the door 3 to be clicked.
- Call the “getMouse()” function
- Call the main function.

Explanation of Solution
Program:
Refer the program “button.py” given in the “Chapter 10” from the text book. Add the method “update()” along with the given code.
#Define the method update
def update(self, win, label):
#Call the method undraw()
self.label.undraw()
#Assign the position to centre
center = self.center
#Assign the label
self.label = Text(center, label)
#Set active to false
self.active = False
#Call the method draw()
self.label.draw(win)
Main.py:
#Import the required packages
from button import Button
from graphics import GraphWin, Point, Text
from random import random
#Definition of main method
def main():
#Creating the application window by setting title, cords and background
win = GraphWin("Three Button Monte", 500, 300)
win.setCoords(-12, -12, 12, 12)
win.setBackground("green3")
#Draw the interface widget by creating three button
door1 = Button(win, Point(-7.5, -3), 5, 6, "Door 1")
door2 = Button(win, Point(0, -3), 5, 6, "Door 2")
door3 = Button(win, Point(7.5, -3), 5, 6, "Door 3")
#Assign the text to the interface
direction = Text(Point(0, 10), "Pick a Door")
#Call the method draw
direction.draw(win)
#Get the random number
x = random() * 3
#Assign the values to false
point1 = point2 = point3 = False
#check the condition
if 0 <= x <1:
#Assign point1 to True
point1 = True
#Chcek the condition
elif 1 <= x < 2:
#Assign the point2 to True
point2 = True
#Otherwise
else:
#Assign the point3 to True
point3 = True
#Activate all the three doors
door1.activate()
door2.activate()
door3.activate()
#Get the action mouse click
click = win.getMouse()
#Check the condition using "while" loop
while door1.clicked(click) or door2.clicked(click) or door3.clicked(click):
#If door1 is clicked
if door1.clicked(click):
#Assign point1 to true
if point1 == True:
#Update the interface
door1.update(win,"Victory!")
else:
#If door1 is clicked
if door2.clicked(click):
#Update the interface
door2.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door3.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
#If door2 is clicked
elif door2.clicked(click):
#Assign point2 to true
if point2 == True:
#Update the interface
door2.update(win,"Victory!")
else:
#If door2 is clicked
if door3.clicked(click):
#Update the interface
door3.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door1.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
else:
#Assign point3 to true
if point3 == True:
#Update the interface
door3.update(win,"Victory!")
else:
#If door2 is clicked
if door2.clicked(click):
#Update the interface
door2.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door1.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
#Call the getMouse()
win.getMouse()
#Call the main function
main()
Output:
Screenshot of output
Screenshot of output
Want to see more full solutions like this?
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Write a program that plays rock paper scissors against the user. Ask the user for what they want to throw (rock, paper, or scissors) and then have the computer randomly pick their throw. Then check to see who wins (or if it’s a tie). You can interpret 1 as rock, 2 as paper, and 3 as scissors if you’d like (or any other combination of numbers). 2. Craps is a dice game played at many casinos. A player rolls a pair of normal six-sided dice. If the initial roll is 2, 3, or 12, the player loses. If the roll is 7 or 11, the player wins. Any other initial roll causes the player to roll for point. That is, the player keeps rolling the dice until either rolling a 7 or re-rolling the value of the initial roll. If the player re-rolls the initial value before rolling a 7, it’s a win. Rolling a 7 first is a loss. Write a program to simulate multiple games of craps and estimate the probability that the player wins. For example, if the player wins 249 out of 500 games, then the estimated probability…arrow_forwardWrite a program that displays twocircles with radius 10 at location (40, 40) and (120, 150) with a line connectingthe two circles, as shown in Figure . The distance between the circlesis displayed along the line. The user can drag a circle. When that happens, thecircle and its line are moved, and the distance between the circles is updated.arrow_forwardWrite a program that asks the user to enter a 1st color choice from a set of options: red, yellow or blue, and then asks them to enter a 2nd color choice from the remaining 2arrow_forward
- Write a program that simulates a snail trying to crawl up a building of height 6steps. The snail starts on the ground, at height 0. In each iteration, the snaileither crawls up one step, or slips off one step and falls all the way back to theground. In each iteration you would take a number as input from user and if the number is less 50 than the snail will slip otherwise it will crawl up. The program should keep going until the snail gets to the top of the building. It should then print out the number of falls that the snail took before it finally reached the top.Here is a sample execution:Iteration 1: Please enter a number between [1,100]: 60 Snail Moved: UpIteration 2: Please enter a number between [1,100]: 60 Snail Moved: UpIteration 2: Please enter a number between [1,100]: 40 Snail Moved: DownNumber of falls: 8arrow_forwardWrite a program that displays the classic BINGO game, displays a BINGO card (5x5 square), and tests the bingo card for a winner via 2 usersarrow_forwardWrite a program that prompts the user to enter thecenter x-, y-coordinates, width, and height of two rectangles and determineswhether the second rectangle is inside the first or overlaps with the first, as shownin Figure . Test your program to cover all cases.arrow_forward
- Write a program that plays a dice game called "21" It is a variation on BlackJack where one player plays against the computer trying to get 21 or as close to 21 without going over. Here are the rules of the game: You will play with dice that have numbers from 1 to 11. To win, the player or the computer has to get to 21, or as close as possible without going over. If the player or computer goes over 21, they instantly lose. If there is a tie, the computer wins. Starting the game: The player is asked to give the computer a name. For now, we'll simply call the computer opponent, "computer." The game starts with rolling four dice. The first two dice are for the player. These two dice are added up and the total outputted to the screen. The other two dice are for the computer. Likewise, their total is outputted to the screen. Player: If a total of 21 has been reached by either the player or the computer, the game instantly stops and the winner is declared. Otherwise,…arrow_forwardWrite a program that plays a dice game called "21" It is a variation on BlackJack where one player plays against the computer trying to get 21 or as close to 21 without going over. Here are the rules of the game: You will play with dice that have numbers from 1 to 11. To win, the player or the computer has to get to 21, or as close as possible without going over. If the player or computer goes over 21, they instantly lose. If there is a tie, the computer wins. Starting the game: The player is asked to give the computer a name. For now, we'll simply call the computer opponent, "computer." The game starts with rolling four dice. The first two dice are for the player. These two dice are added up and the total outputted to the screen. The other two dice are for the computer. Likewise, their total is outputted to the screen. Player: If a total of 21 has been reached by either the player or the computer, the game instantly stops and the winner is declared. Otherwise,…arrow_forwardWrite a program that prompts the user to enter the radius of the rings and draws an Olympic symbol of five rings of the same size with the colors blue, black, red, yellow, and green, as shown in Figure 3.5c.arrow_forward
- Write a program that prompts the user to enter the three dimensions, possibly with decimals, of a cardboard shipping box. The program should then print the volume of the box accurate to two decimal places and the total surface area of the box accurate to three decimal places.arrow_forwardWrite a program to determine the price for a portrait sitting. The price is determined by subjects in portraits, background chosen, and sitting appointment day. The fee schedule is shown below. Fancy background and sitting appointments cost an extra 10 percent more than the base price.arrow_forwardWrite a program that animates a pendulum swinging,as shown in Figure . Press the up arrow key to increase the speed, and thedown arrow key to decrease it. Press the S key to stop animation of and the Rkey to resume it.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
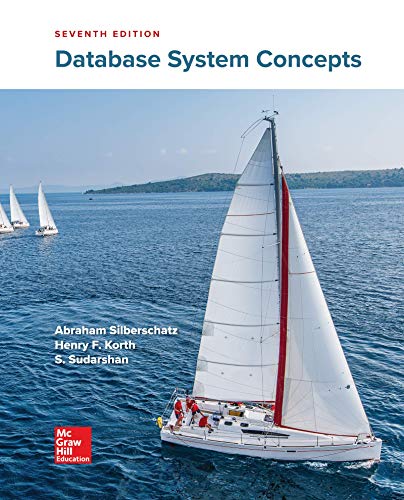
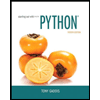
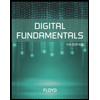
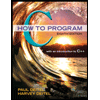
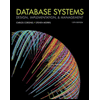
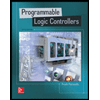