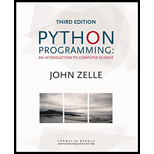
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10, Problem 6PE
Program Plan Intro
Adding method “addLetterGrade”
Program Plan:
- Import the required packages.
- Definition of class Student.
- Definition of “__init__” method.
- Set the name, hours and quality points..
- Definition of “getName()”.
- Return the name.
- Definition of “GetHours()”.
- Return the hours.
- Definition of “getQPoints()”
- Return the quality points.
- Definition of “gpa()”.
- Return the “gpa”.
- Definition of “addGrade()”.
- Calculate the number of hours attended.
- Calculate the grade points.
- Definition of “addLetterGrade()”.
- Assign “letterGrade” to the variable.
- Check whether the “letterGrade” equals to “A”.
- Assign “gradePoint” to “4”.
- Check whether the “letterGrade” equals to “B”.
-
- Assign “gradePoint” to “3”.
- Check whether the “letterGrade” equals to “C”.
-
- Assign “gradePoint” to “2”.
- Check whether the “letterGrade” equals to “D”.
-
- Assign “gradePoint” to “1”.
- Assign “gradePoint” to “4”.
- Definition of “makeStudent()”.
- Return the name, hours and quality points of the student.
- Definition of “__init__” method.
- Declare a main function. Inside the main function,
- Create an object for student.
- Get the grade points and credits from the user.
- Check whether the entered digit is letter.
- Evaluate the expression.
- Call the method “addGrade()”.
- Otherwise, call the method “addLetterGrade()”.
- Print the result.
- Call the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the code for the timeTick method in ClockDisplay that displays hours, minutes, and seconds, or even implement the whole class if you wish.
Can you implement the Student class using the concepts of encapsulation? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first.
You are given a Student class in the editor. Your task is to add two fields:
● String name
● String rollNumber
and provide getter/setters for these fields:
● getName
● setName
● getRollNumber ● setRollNumber
Implement this class according to the rules of encapsulation.
Input #
Checking all fields and getters/setters
Output #
Expecting perfectly defined fields and getter/setters.
There is no need to add constructors in this class.
Create a new project for this program called TestOldMaid and add a class with a main() method.
In the project:
Copy your Deck and Card class from the earlier project into it.
Create a subclass of Deck called OldMaidDeck. It is special because one of the Queens is missing so it only has 51 cards. Create a constructor method that calls the super class constructor, then removes a queen. Override the toString method so it returns the name of the deck and the number of cards in it.
Write the test main() method. Create an OldMaidDeck object and deal all the cards to six players. It is ok if not everyone has an equal number of cards. Use arrays or ArrayLists for the players hands.
Show the hands of all 6 players.
Refer to the web to find out more about the Old Maid card game: https://bicyclecards.com/how-to-play/old-maid/
Fully document all classes with your name, date and description. And each data member and method is documented. Each block that does something is also documented.
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 10 - Prob. 1TFCh. 10 - Prob. 2TFCh. 10 - Prob. 3TFCh. 10 - Prob. 4TFCh. 10 - Prob. 5TFCh. 10 - Prob. 6TFCh. 10 - Prob. 7TFCh. 10 - Prob. 8TFCh. 10 - Prob. 9TFCh. 10 - Prob. 10TF
Ch. 10 - Prob. 1MCCh. 10 - Prob. 2MCCh. 10 - Prob. 3MCCh. 10 - Prob. 4MCCh. 10 - Prob. 5MCCh. 10 - Prob. 6MCCh. 10 - Prob. 7MCCh. 10 - Prob. 8MCCh. 10 - Prob. 9MCCh. 10 - Prob. 10MCCh. 10 - Prob. 1DCh. 10 - Prob. 3DCh. 10 - Prob. 1PECh. 10 - Prob. 2PECh. 10 - Prob. 3PECh. 10 - Prob. 4PECh. 10 - Prob. 5PECh. 10 - Prob. 6PECh. 10 - Prob. 7PECh. 10 - Prob. 8PECh. 10 - Prob. 9PECh. 10 - Prob. 10PECh. 10 - Prob. 11PECh. 10 - Prob. 13PECh. 10 - Prob. 15PECh. 10 - Prob. 16PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create a new project for this program called TestOldMaid and add a class with a main() method. In the project: Copy your Deck and Card class from the earlier project into it. Create a subclass of Deck called OldMaidDeck. It is special because one of the Queens is missing so it only has 51 cards. Create a constructor method that calls the super class constructor, then removes a queen. Override the toString method so it returns the name of the deck and the number of cards in it. Write the test main() method. Create an OldMaidDeck object and deal all the cards to six players. It is ok if not everyone has an equal number of cards. Use arrays or ArrayLists for the players hands. Show the hands of all 6 players. Refer to the web to find out more about the Old Maid card game. Fully document all classes with your name, date and description. And each data member and method is documented. Each block that does something is also documented. Without removing the Queensarrow_forwardalso need help Write a second constructor that takes a String array of pages as input and sets the String array instance variable equal to the input. Continue to default the page number to zero. Part 2: Write a getter and a setter method for the current page number variable. The setter should check to make sure that the input is a valid page number and only update the variable if the new value is valid. Part 3: Write a getCurrentPage method that returns the String of the current page indexed by current_page. public class Ebook{ private String[] pages; private int current_page; //constructor public Ebook() { this.pages = {"See Spot.", "See Spot run.", "Run, Spot, run."}; this.current_page = 0; }}arrow_forwardIn this exercise, you are going to build on your Circleclass from the previous exercise. You are going to add 2 method, areaDifference and perimeterDifference. Both methods take a doubleradius of a second circle and return the difference from the current circle. For example, if you create a Circle object with a radius of 4 and call areaDifference(3), you will return the diffence between the area of a circle with radius 4 and the area of a circle with a radius of 3. perimeterDifferencewould be the same. Make sure you create at least one Circle and test and print the results of your methods. in javaarrow_forward
- An obvious continuation is to use random numbers to create namesfor the stars and planets. Instead of creating random strings of characters, names usually follow certain rules. Select a set of real-world names (e.g. from J.R.R. Tolkien’sworld) and invent a set of rules that they follow. Design and implement a methodthat creates new names based on the set of rules and random numbers.arrow_forwardIn this exercise, you are going to build on your Circleclass from the previous exercise. You are going to add 2 method, areaDifference and perimeterDifference. Both methods take a doubleradius of a second circle and return the difference from the current circle. For example, if you create a Circle object with a radius of 4 and call areaDifference(3), you will return the diffence between the area of a circle with radius 4 and the area of a circle with a radius of 3. perimeterDifferencewould be the same. Make sure you create at least one Circle and test and print the results of your methods. given: public class Circle{private double radius;public Circle(double theRadius){radius = theRadius;}// Add a method called area that returns the area of a circle// using Math.PIpublic double area(){return Math.PI*radius*radius;}// Add a method called perimeter that returns the perimeter of a// circle using Math.PIpublic double perimeter(){return Math.PI*2*radius;}}arrow_forwardThe obvious next step is to name the stars and planets using random numbers. Names usually follow certain rules rather than being made up of random strings of characters. Choose a set of real-world names (for example, from J.R.R. Tolkien's world) and create a set of rules for them to follow. Create and implement a method for generating new names based on a set of rules and random numbers.arrow_forward
- Add a method called multiplesOfFive to the Exercise class. The method must have a void return type and take a single int parameter called limit. In the body of the method, write a while loop that prints out all multiples of 5 between 10 and limit (inclusive) on a single line. For instance, if the value of limit were 15 then the method will print: 10 15 You can use the printEvenNumbers method that is already in the Exercise class as an example to help you work out how to complete this method. In the main method of the Main class, add a call on the Exercise object to your multiplesOfFive method that prints the multiples of 5 between 10 and 25. Add a method called sum to the Exercise class. The method must have a void return type and take no parameters. In the body of the method, write a while loop to sum the values 1 to 10 and print the sum once the loop has finished. In the main method of the Main class, add a call on the Exercise object to your sum method. Check that it prints: 55 Add…arrow_forwardWrite a method that takes two string parameters, and tells whether the first is a substring of the second. You can't use framework methods that do this for you, such as indexOf(). In other words, you have to write the loops yourself. But, you can use the primitive methods such as charAt(). Also analyze the program's performance and state the big-O complexity of your method. Provide a screenshot of the code working.arrow_forwardModify the ClockPane class to drawthe clock with more details on the hours and minutes, as shown in Figure .arrow_forward
- Write out what you think the outer wrappers of the Student and LabClass classes might look like; do not worry about the inner part.arrow_forwardAdd additional information to the student class below such as name, address, major, and contact information along with all the getters and setters (methods) needed to access all data. Submit your code along with a sample run of your program. Comment all your code. Cite any sources of information you use. Write a note on the process of completing the assignment in a Microsoft Word document. // ShowStudent.java // client to test the Student class class ShowStudent { public static void main (String args[]) { Student pupil = new Student(); // We need a new student named pupil pupil.setIDnumber(234); // We are setting pupil's ID number to 234 pupil.setPoints(47); // We are setting pupil's points to 47 pupil.setHours(15); // We are setting pupil's hours to 15 pupil.showIDnumber(); // We are showing pupil's ID number pupil.showPoints(); // We are showing pupil's points pupil.showHours(); // We are showing pupil's hourse System.out.println("The grade point average is " +…arrow_forwardPLEASE ENSURE TO USE THE FRAMEWORK PROVIDED IN THE IMAGES, AND THAT IT WORKS WITH THE TESTER CLASS. PLEASE EDIT BOTH THE TEST CLASS, DO NOT EDIT THE MAIN METHOD. Write a BankAccountTester class whose main method constructs a bank account, deposits $1,000, withdraws $500, withdraws another $400, and then prints the remaining balance. Also print the expected result.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
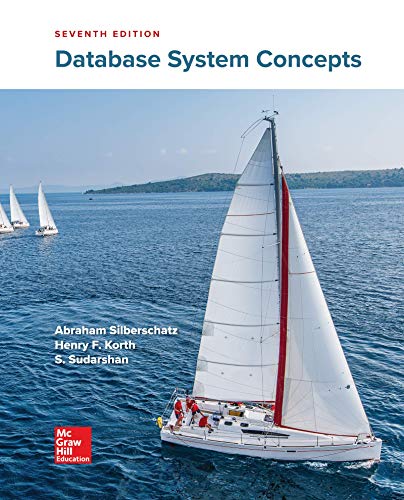
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
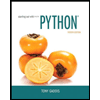
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
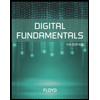
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
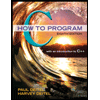
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
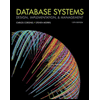
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
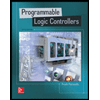
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Java Math Library; Author: Alex Lee;https://www.youtube.com/watch?v=ufegX5o8uc4;License: Standard YouTube License, CC-BY