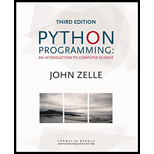
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 11PE
Program Plan Intro
Playing cards
Program Plan:
playingcard.py
- Import the required packages.
- Definition of main “init” method.
- Assign the rank and suit.
- Definition of “getRank” method.
- Return the rank value.
- Definition of “getSuit” method.
- Return the suit value.
- Definition “BJValue” method.
- Check the condition whether the rank value exits between 10 and 13.
- Assign rank to 10.
- Otherwise, Assign the rank value and return the rank.
- Assign rank to 10.
- Check the condition whether the rank value exits between 10 and 13.
- Definition of “str” method.
- Assign rank to “n”.
- Check the “d” equal to the value of suit.
- Assign “Diamonds” to “s”.
- Check the “s” equal to the value of suit.
- Assign “Spades” to “s”.
- Check the “h” equal to the value of suit.
- Assign “Hearts” to “s”.
- Otherwise, Assign “Clubs” to “s”.
- Create an array for the set of card values.
- Loop to execute the values of card.
- Return the values of card name.
- Check the “s” equal to the value of suit.
- Assign “Diamonds” to “s”.
Main.py:
- Import the required packages.
- Definition of main method.
- Assign “20” to “n”.
- Traverse the “for” loop until reaches the value of n.
- Get the random number and store it in “x”.
- Get the random number and store it in “y”.
- Create an array named suit.
- Call the method “PlayingCard”.
- Print the result.
- Call the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
solve with java
Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods:
set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero.
set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero.
display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9”
getScore: it will return the value of score.
greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score.
Create a class “Main” having main method to perform following tasks.
Create two objects of Subject class having…
Die
Write a Die class to model a die (like you play Yahtzee or Monopoly with).
Fields
faces
The number of sides on the die.
value
The last rolled value (generated by the roll() method).
Methods
__init__(self, faces=6)
Constructor
roll(self)
returns a random number between 1 and numSides (inclusive)
lick(self)
Prints "You licked a die." to the screen.
__str__(self)
returns the string version of a die;
Example: "[d6] 3" where 6 is the number of sides and 3 is the value.
DieSet
Write a class named DiceSet that contains a list of Die objects.
Fields
dice
a list of Die objects
Methods
__init__(self)
The constructor should instantiate the dice list to an empty list.
pop_die(self, value)
Remove and return the first die with the given value from the list.
add_die(self, die)
Adds the given die to the dice list.
roll(self)
Roll all the die objects in the list of dice and return their sum.
lick(self)
Lick all the die objects in the list of dice.…
Die
Write a Die class to model a die (like you play Yahtzee or Monopoly with).
Fields
faces
The number of sides on the die.
value
The last rolled value (generated by the roll() method).
Methods
__init__(self, faces=6)
Constructor
roll(self)
returns a random number between 1 and numSides (inclusive)
lick(self)
Prints "You licked a die." to the screen.
__str__(self)
returns the string version of a die;
Example: "[d6] 3" where 6 is the number of sides and 3 is the value.
DieSet
Write a class named DiceSet that contains a list of Die objects.
Fields
dice
a list of Die objects
Methods
__init__(self)
The constructor should instantiate the dice list to an empty list.
pop_die(self, value)
Remove and return the first die with the given value from the list.
add_die(self, die)
Adds the given die to the dice list.
roll(self)
Roll all the die objects in the list of dice and return their sum.
lick(self)
Lick all the die objects in the list of dice.…
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 10 - Prob. 1TFCh. 10 - Prob. 2TFCh. 10 - Prob. 3TFCh. 10 - Prob. 4TFCh. 10 - Prob. 5TFCh. 10 - Prob. 6TFCh. 10 - Prob. 7TFCh. 10 - Prob. 8TFCh. 10 - Prob. 9TFCh. 10 - Prob. 10TF
Ch. 10 - Prob. 1MCCh. 10 - Prob. 2MCCh. 10 - Prob. 3MCCh. 10 - Prob. 4MCCh. 10 - Prob. 5MCCh. 10 - Prob. 6MCCh. 10 - Prob. 7MCCh. 10 - Prob. 8MCCh. 10 - Prob. 9MCCh. 10 - Prob. 10MCCh. 10 - Prob. 1DCh. 10 - Prob. 3DCh. 10 - Prob. 1PECh. 10 - Prob. 2PECh. 10 - Prob. 3PECh. 10 - Prob. 4PECh. 10 - Prob. 5PECh. 10 - Prob. 6PECh. 10 - Prob. 7PECh. 10 - Prob. 8PECh. 10 - Prob. 9PECh. 10 - Prob. 10PECh. 10 - Prob. 11PECh. 10 - Prob. 13PECh. 10 - Prob. 15PECh. 10 - Prob. 16PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Charge Account ValidationCreate a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forwardsolve eith java :- Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having…arrow_forwardGreetings. The programming language to be used is: JAVA Please write a GUI that implements a game, where the user has to click the buttons in the order of their numbering. * The 9 buttons are arranged in a grid of 3 by 3. Each one has a different number from 1 to 9. * If the user selects the correct button, it should turn green and become disabled. The score should beincremented by 1. * If the user selects the incorrect button, it should turn red and all the buttons should become disabled. * If the user selects the “Reset” button, all buttons should be white and become enabled, their random numbers should be reassigned and the score set to 0. Thank you.arrow_forward
- ✓ Exercises Exercise: Write a method _ and _ that computes the region representing the intersection of two regions. Exercise: Write a method _contains__, which checks whether a n-dimensional point belongs to the region. Remember, a point belongs to the region if it belongs to one of the rectangles in the region. ✓ Membership of a point in a region #@title Membership of a point in a region def region_contains (self, p): ### YOUR SOLUTION HERE Region._contains = region_contains [ ] # Tests 10 points. assert (2, 1) in Region (Rectangle((0, 2), (0, 3)), Rectangle((4, 6), (5,8))) assert (2, 1) not in Region (Rectangle((0, 1), (0, 3)), Rectangle((4, 6), (5, 8))) Exercise: Write a method _le_ for regions such that R <= S if the region R is contained in the region S. You can test this by checking that the difference between R and S is empty.arrow_forwardCharge Account Validation Using Java programming Create a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forwardRainfall Type Create a RainFall class that keeps the total rainfall in an array of doubles for each of the 12 months. Methods in the programme should return the following values: • the total annual rainfall; • the average monthly rainfall • the wettest month of the year • the month with the least amount of rain Demonstrate the class in its entirety. Validation of Input: Accepting negative amounts for monthly rainfall data is not permitted.arrow_forward
- Exercise 1: Write a Java application that simulates a test. The test contains at least five questions about first three lectures of this course. Each question should be a multiple-choice question with 4 options. Design a Test class. Use programmer-defined methods to implement your solution. For example: create a method to simulate the questions – simulateQuestion create a method to check the answer – checkAnswer create a method to display a random message for the user – generateMessage create a method to interact with the user - inputAnswer Display the questions using methods of JOptionPane class. Use a loop to show all the questions. For each question: If the user finds the right answer, display a random congratulatory message (“Excellent!”,”Good!”,”Keep up the good work!”, or “Nice work!”). If the user responds incorrectly, display an appropriate message and the correct answer (“No. Please try again”, “Wrong. Try once more”, “Don't give up!”, “No. Keep trying..”). Use…arrow_forwardDice Rolling Class In this problem, you will need to create a program that simulates rolling dice. To start this project, you will first need to define the properties and behaviors of a single die that can be reused multiple times in your future code. This will be done by creating a Dice class. Create a Dice class that contains the following members: Two private integer variables to store the minimum and maximum roll possible. Two constructors that initialize the data members that store the min/max possible values of rolls. a constructor with default min/max values. a constructor that takes 2 input arguments corresponding to the min and max roll values Create a roll() function that returns a random number that is uniformly distributed between the minimum and maximum possible roll values. Create a small test program that asks the user to give a minValue and maxValue for a die, construct a single object of the Dice class with the constructor that initializes the min and max…arrow_forwardJava Programming Travel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. This process is illustrated in the following example: Step 1. Enter the ticket number; for example, 123454. Step 2. Remove the last digit, leaving 12345. Step 3. Determine the remainder when the ticket number is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4. Assign the Boolean value of the comparison between the remainder and the digit dropped from the ticket number. Step 5. Display the result—true or false—in a message box. Accept the…arrow_forward
- Using oop in java Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score.arrow_forwardUse Java programming language Write a program that asks the user to enter 5 test grades (use an array to store them). Output the grades entered, the lowest and highest grade, the average grade, how many grades are above the average and how many are below and the letter grade for the average grade. Create a method that returns the lowest grade. Create a method that returns the highest grade. Create a method that returns the average grade. Create a method that returns how many grades were above the average. Create a method that returns how many grades were below the average. Create a method that returns the letter grade of the average (90-100 - A, 80-89 - B, 70-79 - C, < 70 - F)arrow_forwardTravel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. Accept the ticket number from the agent and verify whether it is a valid number. Test the application with the following ticket numbers: . 123454; the comparison should evaluate to true . 147103; the comparison should evaluate to true . 154123; the comparison should evaluate to false Save the program as TicketNumber.java.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
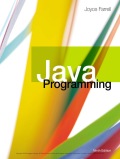
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
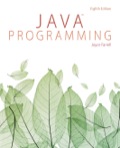
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY