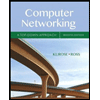
There is something wrong with this Java program but I do not know what it is. can someone help
2 import java.awt.*;
3 import javax.swing.*;
4 import java.awt.event.*;
5 import java.awt.Color;
6
7
8 class Radiobutton extends JFrame {
9
10
11 JRadioButton jRadioButton1;
12 JRadioButton jRadioButton2;
13 JButton jButton;
14 ButtonGroup G1;
15 JLabel L1;
16
17 public Radiobutton()
18 {
19 this.setLayout(null);
20 jRadioButton1 = new JRadioButton();
21 jRadioButton2 = new JRadioButton();
22 jButton = new JButton("Click");
23 G1 = new ButtonGroup();
24 L1 = new JLabel("Qualification");
25
26 jRadioButton1.setText("Under-Graduate");
27 jRadioButton2.setText("Graduate");
28
29 jRadioButton1.setBounds(120, 30, 120, 50);
30 jRadioButton2.setBounds(250, 30, 80, 50);
31 jButton.setBounds(125, 90, 80, 30);
32 L1.setBounds(20, 30, 150, 50);
33
34 this.add(jRadioButton1);
35 this.add(jRadioButton2);
36 this.add(jButton);
37 this.add(L1);
38
39 G1.add(jRadioButton1);
40 G1.add(jRadioButton2);
41
42 jButton.addActionListener(new ActionListener() {
43
44
45 public void actionPerformed(ActionEvent e) {
46
47 String qual = " ";
48
49 if (jRadioButton1.isSelected()) {
50
51 qual = "Under-Graduate";
52 }
53
54 else if (jRadioButton2.isSelected()) {
55
56 qual = "Graduate";
57 }
58 else {
59
60 qual = "NO Button selected";
61 }
62
63 JOptionPane.showMessageDialog(Radiobutton.this, qual);
64 }
65 });
66 }
67 }
68
69 class RadioButton {
70
71 public static void main(String args[]) {
72 Radiobutton f = new Radiobutton();
73 f.setBounds(100, 100, 400, 200);
74 f.setTitle("RadioButtons");
75 f.getContentPane().setBackground(Color.LIGHT_GRAY);
76 f.setVisible(true);
77 }
78 }

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- The first one is answered as follows. Help with the second one. package test;import javax.swing.*;import java.awt.*;import java.awt.event.*; public class JavaApplication1 { public static void main(String[] arguments) { JFrame.setDefaultLookAndFeelDecorated(true);JFrame frame = new JFrame("print X and Y Coordinates");frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setLayout(new BorderLayout());frame.setSize(500,400); final JTextField output = new JTextField();;frame.add(output,BorderLayout.SOUTH); frame.addMouseListener(new MouseListener() {public void mousePressed(MouseEvent me) { }public void mouseReleased(MouseEvent me) { }public void mouseEntered(MouseEvent me) { }public void mouseExited(MouseEvent me) { }public void mouseClicked(MouseEvent me) {int x = me.getX();int y = me.getY();output.setText("Coordinate X:" + x + "|| Coordinate Y:" + y);}}); frame.setVisible(true);}}arrow_forwardThis is Java Programming! We are working with MaxHeap Instructions: Don't modify the two codes below. You need to make a MaxHeapInterface.java that implements the bstMaxHeap in the Driver.java code. If you haven't look at the code, look at them now. Driver.java code: /** A driver that demonstrates the class BstMaxHeap. @author Frank M. Carrano @author Timothy M. Henry @version 5.0*/public class Driver {public static void main(String[] args) { String jared = "Jared"; String brittany = "Brittany"; String brett = "Brett"; String doug = "Doug"; String megan = "Megan"; String jim = "Jim"; String whitney = "Whitney"; String matt = "Matt"; String regis = "Regis"; MaxHeapInterface<String> aHeap = new BstMaxHeap<>(); aHeap.add(jared); aHeap.add(brittany); aHeap.add(brett); aHeap.add(doug); aHeap.add(megan); aHeap.add(jim); aHeap.add(whitney); aHeap.add(matt); aHeap.add(regis); if (aHeap.isEmpty()) System.out.println("The heap is empty -…arrow_forwardImplement the following in the .NET Console App. Write the Bus class. A Bus has a length, a color, and a number of wheels. a. Implement data fields using auto-implemented Properies b. Include 3 constructors: default, the one that receives the Bus length, color and a number of wheels as input (utilize the Properties) and the Constructor that takes the Bus number (an integer) as input.arrow_forward
- I know it is a lot but can someone explain (with comments) line by line what is happening in the below Java program. It assists in my learning/gives me a deeper understanding. Please and thank you! Source code: import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import javax.swing.SwingUtilities; class ProductCalculator { public static int calculateProduct(int[] numbers, int index) { if (index == numbers.length - 1) { return numbers[index]; } else { return numbers[index] * calculateProduct(numbers, index + 1); } }} class RecursiveProductCalculatorView extends JFrame { private JTextField[] numberFields; private JButton calculateButton; private JLabel resultLabel; public RecursiveProductCalculatorView() { setTitle("Recursive Product Calculator"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new GridLayout(7, 1)); numberFields =…arrow_forwardModify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forwardPlease help me with this using java. A starters code is provided (I created a starters code). For more info refer to the image provided. Please ensure tge code works Starter code:import javax.swing.*; import java.applet.*; import java.awt.event.*; import java.awt.*;public class halloween extends Applet implements ActionListener{ int hallow[] [] = ? int rows = 4; int cols = 3; JButton pics[] = new JButton [rows * cols]; public void init () { Panel grid = new Panel (new GridLayout (rows, cols)); int m = 0; for (int i = 0 ; i < rows ; i++) { for (int j = 0 ; j < cols ; j++) { pics [m] = new JButton (createImageIcon ("back.png")); pics [m].addActionListener (this); pics [m].setActionCommand (m + ""); pics [m].setPreferredSize (new Dimension (128, 128)); grid.add (pics [m]); } }m++; }}add (grid); }public void actionPerformed (ActionEvent…arrow_forward
- Using this link: https://docs.oracle.com/javase/tutorial/java/concepts/interface.html and https://docs.oracle.com/javase/tutorial/java/concepts/class.html Create in Java: An interface named bicycle as described in the section: What is an interface? Add a new method for the interface bicycle: ring the bell with a parameter indicating how many time. Pick a bicycle brand and create a new class of bicycles for that brand, as described in the sections What is an interface? and What is a Class? For the new method implementation, write in the console "Clang! Clang!..." for how many times the parameter indicates. Create another class, with a main method, in which you will take a trip aroound your house, changing your bicycle attributes, starting and ending from a complete stop, and ringing the bell once at start and twice at the end. Use as inspiration the method in What is a Class? Your trip must have at least 5 different parts in which you change your bicycle…arrow_forwardJava Program ASAP I want only one program. Please pay attention to the screenshot for test case 2 and 3 and please make sure you match the result. Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.ArrayList;import java.util.Arrays;import java.util.InputMismatchException;import java.util.Scanner;public class FileSorting { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while (true) { System.out.println("Please enter the file name or type QUIT to exit:"); String fileName = scanner.nextLine(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { ArrayList<String> lines = readFile(fileName); if (lines.isEmpty()) { System.out.println("File " + fileName + " is…arrow_forwardI need help With this problem for JAVAFX. if possible can it work in eclipse. GeometricObjects code public abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; }…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
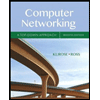
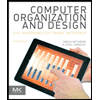
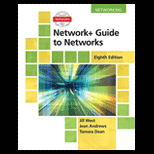
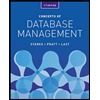
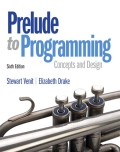
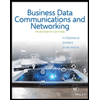