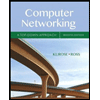
Write a javaFX program . . . (rest of question on picture)
**This is the ClockAnimation.java**
package chapter15;
import chapter14.ClockPane;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.util.Duration;
public class ClockAnimation extends Application {
@Override // Override the start method in the Application class
public void start(Stage primaryStage) {
ClockPane clock = new ClockPane(); // Create a clock
// Create a handler for animation
EventHandler<ActionEvent> eventHandler = e -> {
clock.setCurrentTime(); // Set a new clock time
};
// Create an animation for a running clock
Timeline animation = new Timeline(
new KeyFrame(Duration.millis(1000), eventHandler));
animation.setCycleCount(Timeline.INDEFINITE);
animation.play(); // Start animation
// Create a scene and place it in the stage
Scene scene = new Scene(clock, 250, 250);
primaryStage.setTitle("ClockAnimation"); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
}
/**
* The main method is only needed for the IDE with limited
* JavaFX support. Not needed for running from the command line.
*/
public static void main(String[] args) {
launch(args);
}
}


Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write in Java a code give out what is on the image. Essentially write in the main function so that it prints what is in the image. Im stuck at that part it would be much appreciated if you could help. package javaapplication31; import java.util.Random;import java.util.Scanner;/**** @author */public class JavaApplication31 {String name;/*** @param args the command line arguments*/public static void main(String[] args) {int totalScore = 300;int itrcount =12;int reward;char direction;int x = 0;int y = 0;System.out.println(reward());System.out.println(inputDirection());}public static void displayInfo(int x, int y, int itrCount, int totalScore){x = 0;y = 0;itrCount = 0;totalScore =300;System.out.println("");}public static boolean doesExceed (int x,int y, char direction){return (y > 4 && Character.toLowerCase(direction) == 'u' || x < 0 && Character.toLowerCase(direction) == '1'||y < 0 && Character.toLowerCase(direction) == 'd' || x > 4 &&…arrow_forwardCan you please check what is wrong with my project. I am trying to do a convolution on the following picture, but the error is connected with bounds. Please correct my code. import javax.imageio.ImageIO;import javax.swing.*;import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.IOException;import java.util.Scanner;import java.awt.Color;import java.awt.FlowLayout;import java.awt.image.BufferedImage;import java.io.File;import java.io.IOException;import javax.imageio.ImageIO;import javax.swing.ImageIcon;import javax.swing.JFrame;import javax.swing.JLabel;public class project {public static void main(String[] args) throws IOException {String imagePath = "C:\\Users\\hasib\\Downloads\\11″ TUFTEX BLACK.jpg";int[][] matrix = {{1,0,-1},{2,0,-2},{1,0,-1}}; //edge detectionBufferedImage image = ImageIO.read(new File(imagePath));JFrame frame = new JFrame();frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setLayout(new…arrow_forwardWrite a JavaFX program that acts as a short surveya. Include 3 Questionsi. Ask for favorite Color1. Present choices Red, orange, blue,green as radio buttonsii. Ask For users age1. Do this using a spinner with a range of 10-100iii. Ask user to pick favorite programming language1. Present Java, C++, Python, C# in a choice boxarrow_forward
- What is interesting and difficult about GUI concepts in Java? For instance event handlers, layout managers, frames, panels. polymorphism, JavaFX.arrow_forwardHi I want to create a JAVA program that will allow me to create a canvas (specifically in eclipse). Can someone straighten my code import java.awt.*;import javax.swing.*;class Main extends JFrame { // constructor Main() { super("canvas"); // create a empty canvas Main c = new Main() { // paint the canvas public void paint(Graphics g) { // set color to red g.setColor(Color.red); // set Font g.setFont(new Font("Bold", 1, 20)); // draw a string g.drawString("This is a canvas", 100, 100); } }; // set background c.setBackground(Color.black); add(c); setSize(400, 300); show(); } // Main Method public static void main(String args[]) { Main c = new Main(); }} ReplyForwardarrow_forwardPlease help me fix the java program below. I keep on getting hello world when I run it AnimatedBall.java import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forward
- Please help and thank you in advance. The language is Java. I have to write a program that takes and validates a date but it won't output the full date. Here is my current code: import java.util.Scanner; public class P1 { publicstaticvoidmain(String[]args) { // project 1 // date input // date output // Create a Scanner object to read from the keyboard. Scannerkeyboard=newScanner(System.in); // Identifier declarations charmonth; intday; intyear; booleanisLeapyear; StringmonthName; intmaxDay; // Asks user to input date System.out.println("Please enter month: "); month=(char)keyboard.nextInt(); System.out.println("Please enter day: "); day=keyboard.nextInt(); System.out.println("Please enter year: "); year=keyboard.nextInt(); // Formating months switch(month){ case1: monthName="January"; break; case2: monthName="February"; break; case3: monthName="March"; break; case4: monthName="April"; break; case5: monthName="May"; break; case6: monthName="June"; break; case7:…arrow_forwardWrite a JAVA program that uses a timer to print the current time once a second. Hint: The following code prints the current time Date now = new Date(); System.out.println(now); The Date class is in the Java.util package.arrow_forwardImplement a simple e-mail messaging system. A message has a recipient, a sender, and a message text. A mailbox can store messages. Supply a number of mailboxes for different users and a user interface for users to log insend messages to other users, read their own messages, and log out. Follow the design process that was described in this chapter . Solve by Javaarrow_forward
- The first one is answered as follows. Help with the second one. package test;import javax.swing.*;import java.awt.*;import java.awt.event.*; public class JavaApplication1 { public static void main(String[] arguments) { JFrame.setDefaultLookAndFeelDecorated(true);JFrame frame = new JFrame("print X and Y Coordinates");frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setLayout(new BorderLayout());frame.setSize(500,400); final JTextField output = new JTextField();;frame.add(output,BorderLayout.SOUTH); frame.addMouseListener(new MouseListener() {public void mousePressed(MouseEvent me) { }public void mouseReleased(MouseEvent me) { }public void mouseEntered(MouseEvent me) { }public void mouseExited(MouseEvent me) { }public void mouseClicked(MouseEvent me) {int x = me.getX();int y = me.getY();output.setText("Coordinate X:" + x + "|| Coordinate Y:" + y);}}); frame.setVisible(true);}}arrow_forwardThere is something wrong with this Java program but I do not know what it is. can someone help 2 import java.awt.*; 3 import javax.swing.*; 4 import java.awt.event.*; 5 import java.awt.Color; 6 7 8 class Radiobutton extends JFrame { 9 10 11 JRadioButton jRadioButton1; 12 JRadioButton jRadioButton2; 13 JButton jButton; 14 ButtonGroup G1; 15 JLabel L1; 16 17 public Radiobutton() 18 { 19 this.setLayout(null); 20 jRadioButton1 = new JRadioButton(); 21 jRadioButton2 = new JRadioButton(); 22 jButton = new JButton("Click"); 23 G1 = new ButtonGroup(); 24 L1 = new JLabel("Qualification"); 25 26 jRadioButton1.setText("Under-Graduate"); 27 jRadioButton2.setText("Graduate"); 28 29 jRadioButton1.setBounds(120, 30, 120, 50); 30 jRadioButton2.setBounds(250, 30, 80, 50); 31 jButton.setBounds(125, 90, 80, 30); 32 L1.setBounds(20, 30, 150, 50); 33 34 this.add(jRadioButton1); 35…arrow_forwardThis question is related from my previous question, when I compile this code it gave me several errors. Here's the code with the instructions: import java.text.ParseException;import java.text.SimpleDateFormat;import java.time.LocalDate;import java.time.format.DateTimeFormatter; public class TestWedding{private static SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");//Method to display Wedding details - Couple, Location and Wedding datepublic static void displayWedding(Wedding w){System.out.println("");System.out.println("=== Wedding Information ===");Person p1 = w.getCouple().getFirstPerson();Person p2 = w.getCouple().getSecondPerson();System.out.println(p1.getFirstName() + "" + p2.getLastName() + "(born " + p1.getBirthDate().format(DateTimeFormatter.ISO_DATE)+")"+ " weds " + p2.getFirstName()+ "" + p2.getLastName() + "(born " +…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
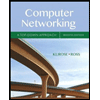
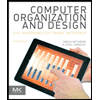
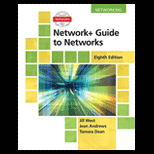
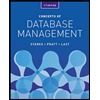
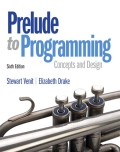
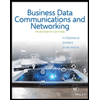