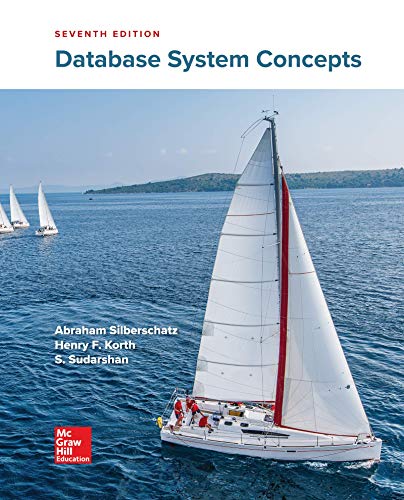
Write a javaFX program . . . (rest of question on picture)
Second picture if for what BounceBallControl.java looks like formatted.
**BounceBallControl.java here**
package chapter15;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.input.KeyCode;
import javafx.scene.input.MouseEvent;
public class BounceBallControl extends Application {
@Override // Override the start method in the Application class
public void start(Stage primaryStage) {
BallPane ballPane = new BallPane(); // Create a ball pane
// Pause and resume animation
// ballPane.setOnMousePressed(e -> ballPane.pause());
EventHandler<MouseEvent> handle1 = new EventHandler<MouseEvent>() {
@Override
public void handle(MouseEvent arg0) {
ballPane.pause();
}};
ballPane.setOnMousePressed(handle1);
ballPane.setOnMouseReleased(e ->{
ballPane.play();
System.out.println("mouse released");
});
// Increase and decrease animation
ballPane.setOnKeyPressed(e -> {
if (e.getCode() == KeyCode.UP) {
ballPane.increaseSpeed();
}
else if (e.getCode() == KeyCode.DOWN) {
ballPane.decreaseSpeed();
}
});
// Create a scene and place it in the stage
Scene scene = new Scene(ballPane, 400, 300);
primaryStage.setTitle("BounceBallControl"); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
// Must request focus after the primary stage is displayed
ballPane.requestFocus();
}
/**
* The main method is only needed for the IDE with limited
* JavaFX support. Not needed for running from the command line.
*/
public static void main(String[] args) {
launch(args);
}
}
}

![package chapter15;
import javafx.application.Application;
import javafx.event.ActionEvent;
5
import javafx.event.EventHandler;
6
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.input. Keycode;
9
import javafx.scene.input.MouseEvent;
10
public class BounceBallControl extends Application {
@override // Override the start method in the Application class
public void start(Stage primaryStage) {
BallPane bal1Pane = new BallPane(); // Create a ball pane
11
12
13
14
15
// Pause and resume animation
// ballPane.setOnMousePressed(e -> ballPane.pause());
16
17
18
19
EventHandlercMouseEvent> handlel = new EventHandler<MouseEvent> () {
20
21
@Override
22
public void handle (MouseEvent arge) {
23
ballPane.pause();
24
}};
25
ballPane.set0nMousePressed(handle1);
26
27
28
ballPane.setOnMouseReleased (e ->{
29
ballPane.play();
30
System.out.printin("mouse released");
31
});
32
// Increase and decrease animation
ballPane.setonkeyPressed (e -> {
if (e.getCode() == KeyCode.UP) {
ballPane.increaseSpeed ();
33
34
35
36
37
else if (e.getCode() KeyCode. DOWN) {
ballPane.decreaseSpeed ();
38
39
40
41
});
42
43
// Create a scene and place it in the stage
44
Scene scene = new Scene(ballPane, 400, 300);
primarystage. setTitle("BounceBallControl1"); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
45
46
47
primarystage. show(); // Display the stage
48
// Must request focus after the primary stage is displayed
ballPane.requestFocus ();
49
50
51
}
52
53
/**
* The main method is only needed for the IDE with limited
* JavaFX support. Not needed for running from the command line.
54
55
56
*/
57
public static void main(String[] args) {
58
launch(args);
59
}
60
}](https://content.bartleby.com/qna-images/question/376a0849-0c16-44f0-be40-275abe9469bd/1285edba-23e4-4968-a610-b3e0ecf396d5/llapqoi_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Hi I want to create a JAVA program that will allow me to create a canvas (specifically in eclipse). Can someone straighten my code import java.awt.*;import javax.swing.*;class Main extends JFrame { // constructor Main() { super("canvas"); // create a empty canvas Main c = new Main() { // paint the canvas public void paint(Graphics g) { // set color to red g.setColor(Color.red); // set Font g.setFont(new Font("Bold", 1, 20)); // draw a string g.drawString("This is a canvas", 100, 100); } }; // set background c.setBackground(Color.black); add(c); setSize(400, 300); show(); } // Main Method public static void main(String args[]) { Main c = new Main(); }} ReplyForwardarrow_forwardPlease help me fix the java program below. I keep on getting hello world when I run it AnimatedBall.java import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forwardPlease help me fix the errors in this java program. I can’t get the program to work Class: AnimatedBall Import java.swing.*; import java.awt.*; import java.awt.event.*; public class AnimatedBall extends JFrame implements ActionListener { private Button btnBounce; // declare a button to play private TextField txtSpeed; // declare a text field to enter number private Label lblDelay; private Panel controls; // generic panel for controls private BouncingBall display; // drawing panel for ball Container frame; public AnimatedBall () { // Set up the controls on the applet frame = getContentPane(); btnBounce = new Button ("Bounce Ball"); //create the objects txtSpeed = new TextField("10000", 10); lblDelay = new Label("Enter Delay"); display = new BouncingBall();//create the panel objects controls = new Panel(); setLayout(new BorderLayout()); // set the frame layout controls.add (btnBounce); // add controls to panel controls.add (lblDelay); controls.add (txtSpeed); frame.add(controls,…arrow_forward
- Please help and thank you in advance. The language is Java. I have to write a program that takes and validates a date but it won't output the full date. Here is my current code: import java.util.Scanner; public class P1 { publicstaticvoidmain(String[]args) { // project 1 // date input // date output // Create a Scanner object to read from the keyboard. Scannerkeyboard=newScanner(System.in); // Identifier declarations charmonth; intday; intyear; booleanisLeapyear; StringmonthName; intmaxDay; // Asks user to input date System.out.println("Please enter month: "); month=(char)keyboard.nextInt(); System.out.println("Please enter day: "); day=keyboard.nextInt(); System.out.println("Please enter year: "); year=keyboard.nextInt(); // Formating months switch(month){ case1: monthName="January"; break; case2: monthName="February"; break; case3: monthName="March"; break; case4: monthName="April"; break; case5: monthName="May"; break; case6: monthName="June"; break; case7:…arrow_forwardCreate a class RectangleExample in eclipse. Copy the following code into the class. RectangleExample.java package mypackage; import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Rectangle; public class RectangleExample extends Application { Rectangle rect1, rect2; @Override public void start(Stage stage) { Group root = new Group(); //Drawing a Rectangle rect1 = new Rectangle(); //Setting the properties of the rectangle rect1.setX(20.0f); rect1.setY(20.0f); rect1.setWidth(300.0f); rect1.setHeight(10.0f); rect1.setFill(javafx.scene.paint.Color.RED); //Add to a Group object root.getChildren().add(rect1); //Drawing a Rectangle rect2 = new Rectangle(); //Setting the properties of the rectangle rect2.setX(20.0f); rect2.setY(40.0f); rect2.setWidth(200.0f); rect2.setHeight(10.0f); rect2.setFill(javafx.scene.paint.Color.GREEN); //Add to a Group object root.getChildren().add(rect2); //Creating a scene…arrow_forwardcan someone help me resize the picture in Java. This is my code: import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.IOException;import javax.imageio.ImageIO; class ImageDisplayGUI { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { createAndShowGUI(); }); } private static void createAndShowGUI() { JFrame frame = new JFrame("Bears"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(800, 600); frame.setLayout(new BorderLayout()); // Load and display the JPG image try { BufferedImage image = ImageIO.read(new File("two bears.png")); // Replace with your image path ImageIcon icon = new ImageIcon(image); JLabel imageLabel = new JLabel(icon); frame.add(imageLabel, BorderLayout.CENTER); } catch (IOException e) { // Handle image loading error…arrow_forward
- The first one is answered as follows. Help with the second one. package test;import javax.swing.*;import java.awt.*;import java.awt.event.*; public class JavaApplication1 { public static void main(String[] arguments) { JFrame.setDefaultLookAndFeelDecorated(true);JFrame frame = new JFrame("print X and Y Coordinates");frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setLayout(new BorderLayout());frame.setSize(500,400); final JTextField output = new JTextField();;frame.add(output,BorderLayout.SOUTH); frame.addMouseListener(new MouseListener() {public void mousePressed(MouseEvent me) { }public void mouseReleased(MouseEvent me) { }public void mouseEntered(MouseEvent me) { }public void mouseExited(MouseEvent me) { }public void mouseClicked(MouseEvent me) {int x = me.getX();int y = me.getY();output.setText("Coordinate X:" + x + "|| Coordinate Y:" + y);}}); frame.setVisible(true);}}arrow_forwardThere is something wrong with this Java program but I do not know what it is. can someone help 2 import java.awt.*; 3 import javax.swing.*; 4 import java.awt.event.*; 5 import java.awt.Color; 6 7 8 class Radiobutton extends JFrame { 9 10 11 JRadioButton jRadioButton1; 12 JRadioButton jRadioButton2; 13 JButton jButton; 14 ButtonGroup G1; 15 JLabel L1; 16 17 public Radiobutton() 18 { 19 this.setLayout(null); 20 jRadioButton1 = new JRadioButton(); 21 jRadioButton2 = new JRadioButton(); 22 jButton = new JButton("Click"); 23 G1 = new ButtonGroup(); 24 L1 = new JLabel("Qualification"); 25 26 jRadioButton1.setText("Under-Graduate"); 27 jRadioButton2.setText("Graduate"); 28 29 jRadioButton1.setBounds(120, 30, 120, 50); 30 jRadioButton2.setBounds(250, 30, 80, 50); 31 jButton.setBounds(125, 90, 80, 30); 32 L1.setBounds(20, 30, 150, 50); 33 34 this.add(jRadioButton1); 35…arrow_forwardThis question is related from my previous question, when I compile this code it gave me several errors. Here's the code with the instructions: import java.text.ParseException;import java.text.SimpleDateFormat;import java.time.LocalDate;import java.time.format.DateTimeFormatter; public class TestWedding{private static SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");//Method to display Wedding details - Couple, Location and Wedding datepublic static void displayWedding(Wedding w){System.out.println("");System.out.println("=== Wedding Information ===");Person p1 = w.getCouple().getFirstPerson();Person p2 = w.getCouple().getSecondPerson();System.out.println(p1.getFirstName() + "" + p2.getLastName() + "(born " + p1.getBirthDate().format(DateTimeFormatter.ISO_DATE)+")"+ " weds " + p2.getFirstName()+ "" + p2.getLastName() + "(born " +…arrow_forward
- I know it is a lot but can someone explain (with comments) line by line what is happening in the below Java program. It assists in my learning/gives me a deeper understanding. Please and thank you! Source code: import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import javax.swing.SwingUtilities; class ProductCalculator { public static int calculateProduct(int[] numbers, int index) { if (index == numbers.length - 1) { return numbers[index]; } else { return numbers[index] * calculateProduct(numbers, index + 1); } }} class RecursiveProductCalculatorView extends JFrame { private JTextField[] numberFields; private JButton calculateButton; private JLabel resultLabel; public RecursiveProductCalculatorView() { setTitle("Recursive Product Calculator"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new GridLayout(7, 1)); numberFields =…arrow_forwardPlease help me fix the errors in the java program below. There are two class AnimatedBall and BouncingBall import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forwardThe question is attached to the image. Please help!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
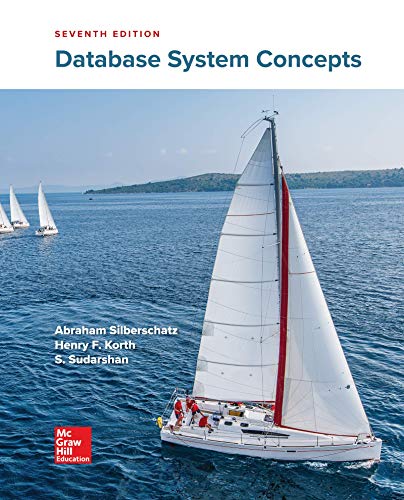
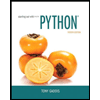
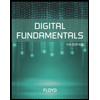
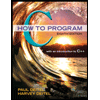
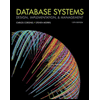
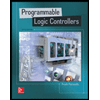