Can you tell me where the graphic code portion and how can I alter to make it just ask about insurance like it asks for their skill level? import java.util.*; import javax.swing.*; import java.lang.*; import java.util.Scanner; public class Pay { private final int REGULAR_HOURS =40; private int skillLevel; private int hoursWorked; private int insuranceOption1; private int insuranceOption2; private int insuranceOption3; private int retirementPlan; private double hourlyPayRate; private double regularPay; private int overtimeHoursWorked; private double overtimePay; private double grossPay; private double deductions; private double insuranceCost; private double retirementCost; private double netPay; Pay(int newSkillLevel, int newHoursWorked, int NewInsuranceOption1, int NewInsuranceOption2, int NewInsuranceOption3, int newRetirementPlan) { skillLevel = newSkillLevel; hoursWorked = newHoursWorked; insuranceOption1 = NewInsuranceOption1; insuranceOption2 = NewInsuranceOption2; insuranceOption3 = NewInsuranceOption3; retirementPlan = newRetirementPlan; setHourlyPayRate(); setOvertimeHoursWorked(); calculateRegularPay(); calculateOvertimePay(); calculateGrossPay(); setInsuranceCost(); setRetirementCost(); calculateDeductions(); calculateNetPay(); } private void setHourlyPayRate() { switch(skillLevel) { case 1: hourlyPayRate = 17.00; break; case 2: hourlyPayRate = 20.00; break; case 3: hourlyPayRate = 22.00; break; default: hourlyPayRate = 0.0; } } private void setOvertimeHoursWorked() { if(hoursWorked > REGULAR_HOURS) overtimeHoursWorked = hoursWorked - REGULAR_HOURS; else overtimeHoursWorked = 0; } private void calculateRegularPay() { if(hoursWorked > 40) regularPay = REGULAR_HOURS * hourlyPayRate; else regularPay = hoursWorked * hourlyPayRate; } private void calculateOvertimePay() {overtimePay=overtimeHoursWorked*(hourlyPayRate*1.5); } private void calculateGrossPay() { grossPay = regularPay + overtimePay; } private void setInsuranceCost() { insuranceCost= 0.0; if(insuranceOption1 == 1) insuranceCost += 32.50; if(insuranceOption2 == 1) insuranceCost += 20.00; if(insuranceOption3 == 1) insuranceCost += 10.00; } private void setRetirementCost() { if(retirementPlan == 1) retirementCost = grossPay * 3./100.; else retirementCost = 0; } private void calculateDeductions() { deductions = insuranceCost + retirementCost; if(deductions > grossPay) deductions = 0; } private void calculateNetPay() { netPay = grossPay - deductions; } public void displayEmployeeDetails() { System.out.println("\nEmployee details..."); System.out.println("Hours worked: " + hoursWorked); System.out.println("Hourly pay rate: " + hourlyPayRate); System.out.println("Regular pay: " + regularPay); System.out.println("Overtime pay: " + overtimePay); System.out.println("Gross pay: " + grossPay); if(deductions > grossPay) System.out.println("Error: the deductions exceed the gross pay, so deductions set to 0."); else System.out.println("Total deductions: " + deductions); System.out.println("Net pay: " + netPay); } public static void main(String[] args) { int in; int skillLevel; int hoursWorked; int insuranceOption1; int insuranceOption2; int insuranceOption3; int retirementPlan; skillLevel = 0; hoursWorked = 0; insuranceOption1 = 0; insuranceOption2 = 0; insuranceOption3 = 0; retirementPlan = 0; Scanner input = new Scanner(System.in); do { System.out.print("Enter skill level of an employee (1/2/3):"); skillLevel = input.nextInt(); if(skillLevel<1||skillLevel>3) System.out.println("must be 1, 2 or 3"); }while(skillLevel<1||skillLevel>3); System.out.print("Enter hours worked: "); hoursWorked=input.nextInt(); if(skillLevel == 2 || skillLevel == 3) { in= JOptionPane.showConfirmDialog(null, "Do you want medical insurance?",null, JOptionPane.YES_NO_OPTION); if(in==JOptionPane.YES_OPTION) insuranceOption1=1; in= JOptionPane.showConfirmDialog(null, "Do you want Dental insurance?",null, JOptionPane.YES_NO_OPTION); if(in==JOptionPane.YES_OPTION) insuranceOption2=1; in= JOptionPane.showConfirmDialog(null, "Do you want Disability?",null, JOptionPane.YES_NO_OPTION); if(in==JOptionPane.YES_OPTION) insuranceOption3=1; } if(skillLevel == 3) { System.out.print("Enter 1 for retirement plan or enter 0: "); retirementPlan = input.nextInt(); } Pay pay= new Pay(skillLevel, hoursWorked, insuranceOption1, insuranceOption2, insuranceOption3, retirementPlan); pay.displayEmployeeDetails(); } }
Can you tell me where the graphic code portion and how can I alter to make it just ask about insurance like it asks for their skill level?
import java.util.*;
import javax.swing.*;
import java.lang.*;
import java.util.Scanner;
public class Pay
{
private final int REGULAR_HOURS =40;
private int skillLevel;
private int hoursWorked;
private int insuranceOption1;
private int insuranceOption2;
private int insuranceOption3;
private int retirementPlan;
private double hourlyPayRate;
private double regularPay;
private int overtimeHoursWorked;
private double overtimePay;
private double grossPay;
private double deductions;
private double insuranceCost;
private double retirementCost;
private double netPay;
Pay(int newSkillLevel, int newHoursWorked, int NewInsuranceOption1, int NewInsuranceOption2, int
NewInsuranceOption3, int newRetirementPlan)
{
skillLevel = newSkillLevel;
hoursWorked = newHoursWorked;
insuranceOption1 = NewInsuranceOption1;
insuranceOption2 = NewInsuranceOption2;
insuranceOption3 = NewInsuranceOption3;
retirementPlan = newRetirementPlan;
setHourlyPayRate();
setOvertimeHoursWorked();
calculateRegularPay();
calculateOvertimePay();
calculateGrossPay();
setInsuranceCost();
setRetirementCost();
calculateDeductions();
calculateNetPay();
}
private void setHourlyPayRate()
{
switch(skillLevel)
{
case 1:
hourlyPayRate = 17.00;
break;
case 2:
hourlyPayRate = 20.00;
break;
case 3:
hourlyPayRate = 22.00;
break;
default:
hourlyPayRate = 0.0;
}
}
private void setOvertimeHoursWorked()
{
if(hoursWorked > REGULAR_HOURS)
overtimeHoursWorked = hoursWorked - REGULAR_HOURS;
else
overtimeHoursWorked = 0;
}
private void calculateRegularPay()
{
if(hoursWorked > 40)
regularPay = REGULAR_HOURS * hourlyPayRate;
else
regularPay = hoursWorked * hourlyPayRate;
}
private void calculateOvertimePay()
{overtimePay=overtimeHoursWorked*(hourlyPayRate*1.5);
}
private void calculateGrossPay()
{
grossPay = regularPay + overtimePay;
}
private void setInsuranceCost()
{
insuranceCost= 0.0;
if(insuranceOption1 == 1)
insuranceCost += 32.50;
if(insuranceOption2 == 1)
insuranceCost += 20.00;
if(insuranceOption3 == 1)
insuranceCost += 10.00;
}
private void setRetirementCost()
{
if(retirementPlan == 1)
retirementCost = grossPay * 3./100.;
else
retirementCost = 0;
}
private void calculateDeductions()
{
deductions = insuranceCost + retirementCost;
if(deductions > grossPay)
deductions = 0;
}
private void calculateNetPay()
{
netPay = grossPay - deductions;
}
public void displayEmployeeDetails()
{
System.out.println("\nEmployee details...");
System.out.println("Hours worked: " + hoursWorked);
System.out.println("Hourly pay rate: " + hourlyPayRate);
System.out.println("Regular pay: " + regularPay);
System.out.println("Overtime pay: " + overtimePay);
System.out.println("Gross pay: " + grossPay);
if(deductions > grossPay)
System.out.println("Error: the deductions exceed the gross pay, so deductions set to 0.");
else
System.out.println("Total deductions: " + deductions);
System.out.println("Net pay: " + netPay);
}
public static void main(String[] args)
{
int in;
int skillLevel;
int hoursWorked;
int insuranceOption1;
int insuranceOption2;
int insuranceOption3;
int retirementPlan;
skillLevel = 0;
hoursWorked = 0;
insuranceOption1 = 0;
insuranceOption2 = 0;
insuranceOption3 = 0;
retirementPlan = 0;
Scanner input = new Scanner(System.in);
do
{
System.out.print("Enter skill level of an employee (1/2/3):");
skillLevel = input.nextInt();
if(skillLevel<1||skillLevel>3)
System.out.println("must be 1, 2 or 3");
}while(skillLevel<1||skillLevel>3);
System.out.print("Enter hours worked: ");
hoursWorked=input.nextInt();
if(skillLevel == 2 || skillLevel == 3)
{
in= JOptionPane.showConfirmDialog(null,
"Do you want medical insurance?",null, JOptionPane.YES_NO_OPTION);
if(in==JOptionPane.YES_OPTION)
insuranceOption1=1;
in= JOptionPane.showConfirmDialog(null,
"Do you want Dental insurance?",null, JOptionPane.YES_NO_OPTION);
if(in==JOptionPane.YES_OPTION)
insuranceOption2=1;
in= JOptionPane.showConfirmDialog(null,
"Do you want Disability?",null, JOptionPane.YES_NO_OPTION);
if(in==JOptionPane.YES_OPTION)
insuranceOption3=1;
}
if(skillLevel == 3)
{
System.out.print("Enter 1 for retirement plan or enter 0: ");
retirementPlan = input.nextInt();
}
Pay pay= new Pay(skillLevel, hoursWorked, insuranceOption1, insuranceOption2, insuranceOption3,
retirementPlan);
pay.displayEmployeeDetails();
}
}

The graphics code portion is Highlighted in the below code
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

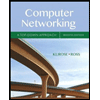
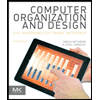
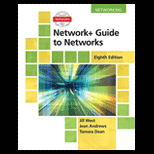
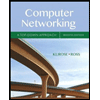
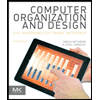
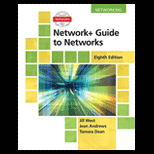
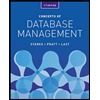
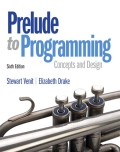
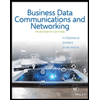