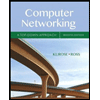
I need help With this problem for JAVAFX. if possible can it work in eclipse.
GeometricObjects code
public abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; } @Override public String toString() { return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled; } /** Abstract method getArea */ public abstract double getArea(); /** Abstract method getPerimeter */ public abstract double getPerimeter(); }
GeometricObjectComparator
import java.util.Comparator; public class GeometricObjectComparator implements Comparator<GeometricObject>, java.io.Serializable { public int compare(GeometricObject o1, GeometricObject o2) { return o1.getArea() > o2.getArea() ? 1 : o1.getArea() == o2.getArea() ? 0 : -1; } }
the error i get is in one of the pictures.
![Write the following generic method using selection sort and a comparator:
public static <E> void selectionSort (E[] list, Comparator<? super E> comparator)
Write a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObject Comparator introduced in Listing 20.5 to sort the elements.
Display the sorted elements. Use the following statement to create the array:
GeometricObject[] list1 = {new Circle(5), new Rectangle(4, 5),
new Circle(5.5), new Rectangle(2.4, 5), new Circle (0.5),
new Rectangle(4, 65), new Circle(4.5), new Rectangle(4.4, 1),
new Circle(6.5), new Rectangle(4, 5)};
Also in the same program, write the code that sorts six strings by their last character. Use the following statement to create the array:
String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"};](https://content.bartleby.com/qna-images/question/c4b4111f-0cb3-46a3-bff1-74a667fd5dd6/fbbaef3e-83e0-483c-9414-98ecb8724539/90zbdiy_thumbnail.png)
![1 package application;
2 import java.util.Comparator;
3 ¤¶
4 public class Main {9
50
public static <E> void selectionSort (E[] list, Comparator<?. super. E> comparator). {9
for (int i = 0; i < list.length 1; i++) {9
int minIndex = i;
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20 ¤¶
210
22
X23
24
25
26
27
28
29
30
31
32
33
34
x35
36
37
38
39
40
41 ¤¶
x
2
for (int j =i+1; j<·list.length; j++) {¤9
if (comparator.compare(list[j], list[minIndex]) < 0) {
minIndex = •j;ng
}ng
}*9
if (minIndex != i) {9
·E· temp·=·list[i];*9
·list[i] = list [minIndex];
.list[minIndex] = temp;
·}x9
public static <GeometricObject> void main(String[] args) {9
.//. Test sorting GeometricObject array
GeometricObject[] list1 = {
new Circle(5), new Rectangle(4, 5),
new Circle(5.5), new Rectangle(2.4, 5), new Circle(0.5)
new Rectangle(4, 65), new Circle (4.5) new Rectangle(4.41),FI
new Circle(6.5), new Rectangle(4, 5)
System.out.println("Before sorting:");"
for. (GeometricObject obj: list1). {9
System.out.println (obj);
selectionSort (list1, new GeometricObjectComparatoc());
System.out.println("\nAfter sorting:");
for (GeometricObject obj: list1). {9
System.out.println(obj);
}¤¶](https://content.bartleby.com/qna-images/question/c4b4111f-0cb3-46a3-bff1-74a667fd5dd6/fbbaef3e-83e0-483c-9414-98ecb8724539/88lq43q_thumbnail.png)

We have been given GeometricObject[] list1 = {new Circle (5), new Rectangle(4, 5),
new Circle(5.5), new Rectangle(2.4, 5), new Circle(0.5),
new Rectangle(4, 65), new Circle(4.5), new Rectangle(4.4, 1),
new Circle(6.5), new Rectangle(4, 5)}; We have to create a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObjectComparator.
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Please help me with this. I am really struggling Please help me modify my java matching with the image provide below or at least fix the errors import java.awt.*; import java.awt.event.*; import javax.swing.*; public class Game extends JFrame implements ActionListener { int hallo[][] = {{4,6,2}, {1,4,3}, {5,5,1}, {2,3,6}}; int rows = 4; int cols = 3; JButton pics[] = new JButton[rows*cols]; public Game() { setSize(600,600); JPanel grid = new JPanel(new GridLayout(rows,cols)); int m = 0; for(int i = 0; iarrow_forwardHere is a command-line inheritance program exercise that has to operate in javaFx. Most of the structure is there, you just need to add functionality. This is the prompt: public class LandAnimal {//make this a subclass of Animal// enum CoveringType fur, skin, scales // make sure all the enum values are public // by convention the enum values should be capitalized // *these should be private// String decoration // variable to store the description of color and patterns - for example "blue fur with orange spots"// CoveringType covering //variable to store CoveringType // make a constructor // add getters and setters for all the attributes}arrow_forwardimport bridges.base.ColorGrid;import bridges.base.Color;public class Scene {/* Creates a Scene with a maximum capacity of Marks andwith a background color.maxMarks: the maximum capacity of MarksbackgroundColor: the background color of this Scene*/public Scene(int maxMarks, Color backgroundColor) {}// returns true if the Scene has no room for additional Marksprivate boolean isFull() {return false;}/* Adds a Mark to this Scene. When drawn, the Markwill appear on top of the background and previously added Marksm: the Mark to add*/public void addMark(Mark m) {if (isFull()) throw new IllegalStateException("No room to add more Marks");}/*Helper method: deletes the Mark at an index.If no Marks have been previously deleted, the methoddeletes the ith Mark that was added (0 based).i: the index*/protected void deleteMark(int i) {}/*Deletes all Marks from this Scene thathave a given Colorc: the Color*/public void deleteMarksByColor(Color c) {}/* draws the Marks in this Scene over a background…arrow_forward
- I need help fix this Java code described below so that it can be followed in the image below package classPackage; import interfaces.RatInterface; public class Rat implements RatInterface { private int ratEnergy = 5; @Override public String move() { // TODO Auto-generated method stub returnnull; } @Override publicint getAliveState() { // TODO Auto-generated method stub return 0; } @Override publicvoid refresh() { ratEnergy = 5; } @Override publicvoid wearDown() { ratEnergy -= 1; } @Override public String getId() { // TODO Auto-generated method stub returnnull; } }arrow_forwardI need help with creating a Java program described in the image below: StringInstrument.java: // TODO: Define a class: StringInstrument that is derived from the Instrument classpublic class StringInstrument extends Instrument { // TODO: Declare private fields // TODO: Define mutator methods - // setNumOfStrings(), setNumOfFrets(), setIsBowed() // TODO: Define accessor methods - // getNumOfStrings(), getNumOfFrets(), getIsBowed() } Instrument.java: public class Instrument { protected String instrumentName; protected String instrumentManufacturer; protected int yearBuilt, cost; public void setName(String userName) { instrumentName = userName; } public String getName() { return instrumentName; } public void setManufacturer(String userManufacturer) { instrumentManufacturer = userManufacturer; } public String getManufacturer(){ return instrumentManufacturer; } public void setYearBuilt(int…arrow_forwardRefer to the following interface: Provide two examples (screenshots) that in many ways could improve the above or similar interface using the principles of direct manipulation. Explain the relevant improvements as well. (Examples might have different object, labels and organization)arrow_forward
- Please teach me how to fix an error from my JAVA source code. I have set up the source code with class name "ProductionWorker" and there were a error occured on second page of sceenshot where it is highlighted. //import the file import java.util.*; //create the class class Employee { //create the variable private String emp_num; //create the date variable private Date hiring_date; // default constructor public Employee() { //assign the emp num. this.emp_num = "000-A"; //assign the Date this.hiring_date = new Date(); } // constructor parameter public Employee(String emp_num, Date hiring_date) { this.emp_num = emp_num; this.hiring_date = hiring_date; } // getter method public String getEmployeeNumber() { return this.emp_num; } // call the gethiringdate function public Date gethiringDate() { // return the date return this.hiring_date; } // setter method public void setEmployeeNumber(String emp_num) { //set the emp num. this.emp_num = emp_num; } //set the hiring date public void…arrow_forwardWhy does the Number Class from this slide need to be abstract?arrow_forwardImplement a date picker in javafx having following properties1) only numerical value can be typed in it2) / ( forward slash) appears in it when form prompt and user can write date in it3)only 8 digits can be typed in itarrow_forward
- please code in java..refer the 2 codes BankAccount and AccountTest.. BankAccount source code is given below and AccountTest source code is added in image Question is to add code to be able to lock the account. for example if someone withdraws a certain amount of money the accounts locks, etc /** The BankAccount class simulates a bank account.*/ public class BankAccount{ private double balance; // Account balance /** This constructor sets the starting balance at 0.0. */ public BankAccount() { balance = 0.0; } /** This constructor sets the starting balance to the value passed as an argument. @param startBalance The starting balance. */ public BankAccount(double startBalance) { balance = startBalance; } /** This constructor sets the starting balance to the value in the String argument. @param str The starting balance, as a String. */ public BankAccount(String str) { balance =…arrow_forwardI need help creating a Java code package in a pyramid with 3 sides (pyramid3) and pyramid with 4 sides (pyramid4) classes as described in the image below package objectsPackage; import interfacePackage.ShapesInterface; public class Pyramid3 implements ShapesInterface { @Override publicdouble getArea() { // TODO Auto-generated method stub return 0; } @Override publicdouble getVolume() { // TODO Auto-generated method stub return 0; } @Override publicvoid displayArea() { // TODO Auto-generated method stub } @Override publicvoid displayVolume() { // TODO Auto-generated method stub } }arrow_forwardPlease help me with this using java. A starters code is provided (I created a starters code). For more info refer to the image provided. Please ensure tge code works Starter code:import javax.swing.*; import java.applet.*; import java.awt.event.*; import java.awt.*;public class halloween extends Applet implements ActionListener{ int hallow[] [] = ? int rows = 4; int cols = 3; JButton pics[] = new JButton [rows * cols]; public void init () { Panel grid = new Panel (new GridLayout (rows, cols)); int m = 0; for (int i = 0 ; i < rows ; i++) { for (int j = 0 ; j < cols ; j++) { pics [m] = new JButton (createImageIcon ("back.png")); pics [m].addActionListener (this); pics [m].setActionCommand (m + ""); pics [m].setPreferredSize (new Dimension (128, 128)); grid.add (pics [m]); } }m++; }}add (grid); }public void actionPerformed (ActionEvent…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
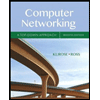
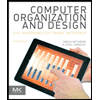
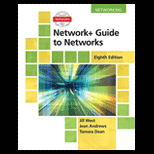
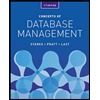
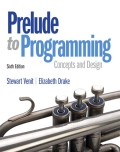
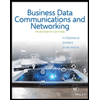