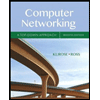
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
starter code: in java pls and thank you!
public class LinkedList {
private Node head;
private Node tail;
public void add(String item) {
Node newItem = new Node(item);
// handles the case where the new item
// is the only thing in the list
if (head == null) {
head = newItem;
tail = newItem;
return;
}
tail.next = newItem;
tail = newItem;
}
public void print() {
Node current = head;
while (current != null) {
System.out.println(current.item);
current = current.next;
}
}
public void printWithSkips() {
// TODO your code here
}
class Node {
String item;
Node next;
public Node(String item) {
this.item = item;
this.next = null;
}
}
}
and
public class Driver {
public static void main(String[] args) {
LinkedList list = new LinkedList();
list.add("Falcons");
list.add("Bears");
list.add("Titans");
list.add("Eagles");
list.add("Panthers");
list.add("Cowboys");
list.add("Steelers");
list.add("49ers");
list.add("Vikings");
list.add("Saints");
list.add("Seahawks");
list.print();
System.out.println("\n");
list.printWithSkips();
}
}
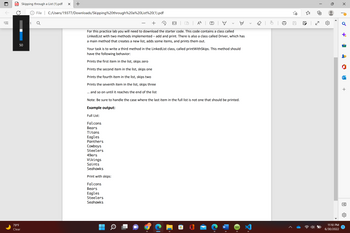
Transcribed Image Text:!!!
PDF
Skipping through a List (1).pdf X +
50
79°F
Clear
1
File C:/Users/19377/Downloads/Skipping%20through%20a%20List%20(1).pdf
T
For this practice lab you will need to download the starter code. This code contains a class called
Linked List with two methods implemented - add and print. There is also a class called Driver, which has
a main method that creates a new list, adds some items, and prints them out.
Your task is to write a third method in the LinkedList class, called printWithSkips. This method should
have the following behavior:
Prints the first item in the list, skips zero
Prints the second item in the list, skips one
Prints the fourth item in the list, skips two
Prints the seventh item in the list, skips three
and so on until it reaches the end of the list
Note: Be sure to handle the case where the last item in the full list is not one that should be printed.
Example output:
Full List:
Falcons
Bears
Titans
Eagles
Panthers
Cowboys
Steelers
➡ | D | A | Ⓡ
→
T
49ers
Vikings
Saints
Seahawks
Print with skips:
Falcons
Bears
Eagles
Steelers
Seahawks
W
|
1+
11:10 PM
6/30/2022
O
+
A
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 4 images

Knowledge Booster
Similar questions
- The numbers on the left margin denote line numbers. line#01 class LL_node {02 int val;03 LL_node next;04 public LL_node (int n) {05 val = n;06 next = null;07 }08 public void set_next (LL_node nextNode) {09 next = nextNode;10 }11 public LL_node get_next () {12 return next;13 }14 public void set_value (int input) {15 val = input;16 }17 public int get_value () {18 return val;19 }20 }21 public class LL {22 protected LL_node head;23 protected LL_node tail;24 public LL () {25 head = null;26 tail = null;27 }28 public int append (int n) {29 if (head == null) {30 head = new LL_node(n);31 tail = head;32 } else {33 LL_node new_node;34 new_node = new LL_node(n);35 tail.set_next (new_node);36 tail = new_node;37 }38 return n;39 }40 } which the following statement…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardRedesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forward
- class Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forward1.Assume some Node class with info link fields . Complete this method in class List that returns a reference to the node containing the data item in the argument findThis, Assume that findThis is in the list public class list { protected Node head ; Protected int size ; Public Node find (char find this) { Node curr = head; while(curr != null) { if(curr.info == findThis) return curr; curr = curr.link; } return -1; } }arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
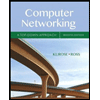
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
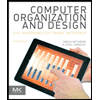
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
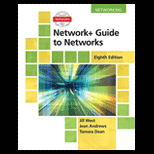
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
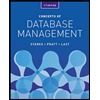
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
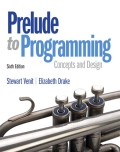
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
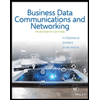
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY